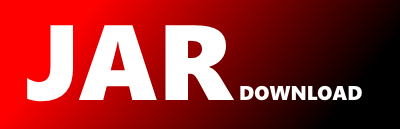
com.pulumi.aws.ec2.kotlin.LaunchConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ec2.kotlin
import com.pulumi.aws.ec2.LaunchConfigurationArgs.builder
import com.pulumi.aws.ec2.kotlin.inputs.LaunchConfigurationEbsBlockDeviceArgs
import com.pulumi.aws.ec2.kotlin.inputs.LaunchConfigurationEbsBlockDeviceArgsBuilder
import com.pulumi.aws.ec2.kotlin.inputs.LaunchConfigurationEphemeralBlockDeviceArgs
import com.pulumi.aws.ec2.kotlin.inputs.LaunchConfigurationEphemeralBlockDeviceArgsBuilder
import com.pulumi.aws.ec2.kotlin.inputs.LaunchConfigurationMetadataOptionsArgs
import com.pulumi.aws.ec2.kotlin.inputs.LaunchConfigurationMetadataOptionsArgsBuilder
import com.pulumi.aws.ec2.kotlin.inputs.LaunchConfigurationRootBlockDeviceArgs
import com.pulumi.aws.ec2.kotlin.inputs.LaunchConfigurationRootBlockDeviceArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Provides a resource to create a new launch configuration, used for autoscaling groups.
* !> **WARNING:** The use of launch configurations is discouraged in favor of launch templates. Read more in the [AWS EC2 Documentation](https://docs.aws.amazon.com/autoscaling/ec2/userguide/launch-configurations.html).
* > **Note** When using `aws.ec2.LaunchConfiguration` with `aws.autoscaling.Group`, it is recommended to use the `name_prefix` (Optional) instead of the `name` (Optional) attribute.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const ubuntu = aws.ec2.getAmi({
* mostRecent: true,
* filters: [
* {
* name: "name",
* values: ["ubuntu/images/hvm-ssd/ubuntu-trusty-14.04-amd64-server-*"],
* },
* {
* name: "virtualization-type",
* values: ["hvm"],
* },
* ],
* owners: ["099720109477"],
* });
* const asConf = new aws.ec2.LaunchConfiguration("as_conf", {
* name: "web_config",
* imageId: ubuntu.then(ubuntu => ubuntu.id),
* instanceType: "t2.micro",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* ubuntu = aws.ec2.get_ami(most_recent=True,
* filters=[
* {
* "name": "name",
* "values": ["ubuntu/images/hvm-ssd/ubuntu-trusty-14.04-amd64-server-*"],
* },
* {
* "name": "virtualization-type",
* "values": ["hvm"],
* },
* ],
* owners=["099720109477"])
* as_conf = aws.ec2.LaunchConfiguration("as_conf",
* name="web_config",
* image_id=ubuntu.id,
* instance_type="t2.micro")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var ubuntu = Aws.Ec2.GetAmi.Invoke(new()
* {
* MostRecent = true,
* Filters = new[]
* {
* new Aws.Ec2.Inputs.GetAmiFilterInputArgs
* {
* Name = "name",
* Values = new[]
* {
* "ubuntu/images/hvm-ssd/ubuntu-trusty-14.04-amd64-server-*",
* },
* },
* new Aws.Ec2.Inputs.GetAmiFilterInputArgs
* {
* Name = "virtualization-type",
* Values = new[]
* {
* "hvm",
* },
* },
* },
* Owners = new[]
* {
* "099720109477",
* },
* });
* var asConf = new Aws.Ec2.LaunchConfiguration("as_conf", new()
* {
* Name = "web_config",
* ImageId = ubuntu.Apply(getAmiResult => getAmiResult.Id),
* InstanceType = "t2.micro",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ec2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* ubuntu, err := ec2.LookupAmi(ctx, &ec2.LookupAmiArgs{
* MostRecent: pulumi.BoolRef(true),
* Filters: []ec2.GetAmiFilter{
* {
* Name: "name",
* Values: []string{
* "ubuntu/images/hvm-ssd/ubuntu-trusty-14.04-amd64-server-*",
* },
* },
* {
* Name: "virtualization-type",
* Values: []string{
* "hvm",
* },
* },
* },
* Owners: []string{
* "099720109477",
* },
* }, nil)
* if err != nil {
* return err
* }
* _, err = ec2.NewLaunchConfiguration(ctx, "as_conf", &ec2.LaunchConfigurationArgs{
* Name: pulumi.String("web_config"),
* ImageId: pulumi.String(ubuntu.Id),
* InstanceType: pulumi.String("t2.micro"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.Ec2Functions;
* import com.pulumi.aws.ec2.inputs.GetAmiArgs;
* import com.pulumi.aws.ec2.LaunchConfiguration;
* import com.pulumi.aws.ec2.LaunchConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var ubuntu = Ec2Functions.getAmi(GetAmiArgs.builder()
* .mostRecent(true)
* .filters(
* GetAmiFilterArgs.builder()
* .name("name")
* .values("ubuntu/images/hvm-ssd/ubuntu-trusty-14.04-amd64-server-*")
* .build(),
* GetAmiFilterArgs.builder()
* .name("virtualization-type")
* .values("hvm")
* .build())
* .owners("099720109477")
* .build());
* var asConf = new LaunchConfiguration("asConf", LaunchConfigurationArgs.builder()
* .name("web_config")
* .imageId(ubuntu.applyValue(getAmiResult -> getAmiResult.id()))
* .instanceType("t2.micro")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* asConf:
* type: aws:ec2:LaunchConfiguration
* name: as_conf
* properties:
* name: web_config
* imageId: ${ubuntu.id}
* instanceType: t2.micro
* variables:
* ubuntu:
* fn::invoke:
* Function: aws:ec2:getAmi
* Arguments:
* mostRecent: true
* filters:
* - name: name
* values:
* - ubuntu/images/hvm-ssd/ubuntu-trusty-14.04-amd64-server-*
* - name: virtualization-type
* values:
* - hvm
* owners:
* - '099720109477'
* ```
*
* ## Import
* Using `pulumi import`, import launch configurations using the `name`. For example:
* ```sh
* $ pulumi import aws:ec2/launchConfiguration:LaunchConfiguration as_conf pulumi-lg-123456
* ```
* @property associatePublicIpAddress Associate a public ip address with an instance in a VPC.
* @property ebsBlockDevices Additional EBS block devices to attach to the instance. See Block Devices below for details.
* @property ebsOptimized If true, the launched EC2 instance will be EBS-optimized.
* @property enableMonitoring Enables/disables detailed monitoring. This is enabled by default.
* @property ephemeralBlockDevices Customize Ephemeral (also known as "Instance Store") volumes on the instance. See Block Devices below for details.
* @property iamInstanceProfile The name attribute of the IAM instance profile to associate with launched instances.
* @property imageId The EC2 image ID to launch.
* @property instanceType The size of instance to launch.
* The following arguments are optional:
* @property keyName The key name that should be used for the instance.
* @property metadataOptions The metadata options for the instance.
* @property name The name of the launch configuration. If you leave this blank, this provider will auto-generate a unique name. Conflicts with `name_prefix`.
* @property namePrefix Creates a unique name beginning with the specified prefix. Conflicts with `name`.
* @property placementTenancy The tenancy of the instance. Valid values are `default` or `dedicated`, see [AWS's Create Launch Configuration](http://docs.aws.amazon.com/AutoScaling/latest/APIReference/API_CreateLaunchConfiguration.html) for more details.
* @property rootBlockDevice Customize details about the root block device of the instance. See Block Devices below for details.
* @property securityGroups A list of associated security group IDS.
* @property spotPrice The maximum price to use for reserving spot instances.
* @property userData The user data to provide when launching the instance. Do not pass gzip-compressed data via this argument; see `user_data_base64` instead.
* @property userDataBase64 Can be used instead of `user_data` to pass base64-encoded binary data directly. Use this instead of `user_data` whenever the value is not a valid UTF-8 string. For example, gzip-encoded user data must be base64-encoded and passed via this argument to avoid corruption.
*/
public data class LaunchConfigurationArgs(
public val associatePublicIpAddress: Output? = null,
public val ebsBlockDevices: Output>? = null,
public val ebsOptimized: Output? = null,
public val enableMonitoring: Output? = null,
public val ephemeralBlockDevices: Output>? =
null,
public val iamInstanceProfile: Output? = null,
public val imageId: Output? = null,
public val instanceType: Output? = null,
public val keyName: Output? = null,
public val metadataOptions: Output? = null,
public val name: Output? = null,
public val namePrefix: Output? = null,
public val placementTenancy: Output? = null,
public val rootBlockDevice: Output? = null,
public val securityGroups: Output>? = null,
public val spotPrice: Output? = null,
public val userData: Output? = null,
public val userDataBase64: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.ec2.LaunchConfigurationArgs =
com.pulumi.aws.ec2.LaunchConfigurationArgs.builder()
.associatePublicIpAddress(associatePublicIpAddress?.applyValue({ args0 -> args0 }))
.ebsBlockDevices(
ebsBlockDevices?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.ebsOptimized(ebsOptimized?.applyValue({ args0 -> args0 }))
.enableMonitoring(enableMonitoring?.applyValue({ args0 -> args0 }))
.ephemeralBlockDevices(
ephemeralBlockDevices?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.iamInstanceProfile(iamInstanceProfile?.applyValue({ args0 -> args0 }))
.imageId(imageId?.applyValue({ args0 -> args0 }))
.instanceType(instanceType?.applyValue({ args0 -> args0 }))
.keyName(keyName?.applyValue({ args0 -> args0 }))
.metadataOptions(metadataOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.name(name?.applyValue({ args0 -> args0 }))
.namePrefix(namePrefix?.applyValue({ args0 -> args0 }))
.placementTenancy(placementTenancy?.applyValue({ args0 -> args0 }))
.rootBlockDevice(rootBlockDevice?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.securityGroups(securityGroups?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.spotPrice(spotPrice?.applyValue({ args0 -> args0 }))
.userData(userData?.applyValue({ args0 -> args0 }))
.userDataBase64(userDataBase64?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LaunchConfigurationArgs].
*/
@PulumiTagMarker
public class LaunchConfigurationArgsBuilder internal constructor() {
private var associatePublicIpAddress: Output? = null
private var ebsBlockDevices: Output>? = null
private var ebsOptimized: Output? = null
private var enableMonitoring: Output? = null
private var ephemeralBlockDevices: Output>? =
null
private var iamInstanceProfile: Output? = null
private var imageId: Output? = null
private var instanceType: Output? = null
private var keyName: Output? = null
private var metadataOptions: Output? = null
private var name: Output? = null
private var namePrefix: Output? = null
private var placementTenancy: Output? = null
private var rootBlockDevice: Output? = null
private var securityGroups: Output>? = null
private var spotPrice: Output? = null
private var userData: Output? = null
private var userDataBase64: Output? = null
/**
* @param value Associate a public ip address with an instance in a VPC.
*/
@JvmName("gpwnnaoulovmdboi")
public suspend fun associatePublicIpAddress(`value`: Output) {
this.associatePublicIpAddress = value
}
/**
* @param value Additional EBS block devices to attach to the instance. See Block Devices below for details.
*/
@JvmName("jnggbsgfanhaaxfj")
public suspend fun ebsBlockDevices(`value`: Output>) {
this.ebsBlockDevices = value
}
@JvmName("ckatfqsevbrmwcxt")
public suspend fun ebsBlockDevices(vararg values: Output) {
this.ebsBlockDevices = Output.all(values.asList())
}
/**
* @param values Additional EBS block devices to attach to the instance. See Block Devices below for details.
*/
@JvmName("vvbyqbdkbpoieohp")
public suspend fun ebsBlockDevices(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy