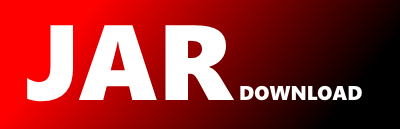
com.pulumi.aws.ec2.kotlin.NetworkInsightsAnalysis.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ec2.kotlin
import com.pulumi.aws.ec2.kotlin.outputs.NetworkInsightsAnalysisAlternatePathHint
import com.pulumi.aws.ec2.kotlin.outputs.NetworkInsightsAnalysisExplanation
import com.pulumi.aws.ec2.kotlin.outputs.NetworkInsightsAnalysisForwardPathComponent
import com.pulumi.aws.ec2.kotlin.outputs.NetworkInsightsAnalysisReturnPathComponent
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.aws.ec2.kotlin.outputs.NetworkInsightsAnalysisAlternatePathHint.Companion.toKotlin as networkInsightsAnalysisAlternatePathHintToKotlin
import com.pulumi.aws.ec2.kotlin.outputs.NetworkInsightsAnalysisExplanation.Companion.toKotlin as networkInsightsAnalysisExplanationToKotlin
import com.pulumi.aws.ec2.kotlin.outputs.NetworkInsightsAnalysisForwardPathComponent.Companion.toKotlin as networkInsightsAnalysisForwardPathComponentToKotlin
import com.pulumi.aws.ec2.kotlin.outputs.NetworkInsightsAnalysisReturnPathComponent.Companion.toKotlin as networkInsightsAnalysisReturnPathComponentToKotlin
/**
* Builder for [NetworkInsightsAnalysis].
*/
@PulumiTagMarker
public class NetworkInsightsAnalysisResourceBuilder internal constructor() {
public var name: String? = null
public var args: NetworkInsightsAnalysisArgs = NetworkInsightsAnalysisArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend NetworkInsightsAnalysisArgsBuilder.() -> Unit) {
val builder = NetworkInsightsAnalysisArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): NetworkInsightsAnalysis {
val builtJavaResource = com.pulumi.aws.ec2.NetworkInsightsAnalysis(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return NetworkInsightsAnalysis(builtJavaResource)
}
}
/**
* Provides a Network Insights Analysis resource. Part of the "Reachability Analyzer" service in the AWS VPC console.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const path = new aws.ec2.NetworkInsightsPath("path", {
* source: source.id,
* destination: destination.id,
* protocol: "tcp",
* });
* const analysis = new aws.ec2.NetworkInsightsAnalysis("analysis", {networkInsightsPathId: path.id});
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* path = aws.ec2.NetworkInsightsPath("path",
* source=source["id"],
* destination=destination["id"],
* protocol="tcp")
* analysis = aws.ec2.NetworkInsightsAnalysis("analysis", network_insights_path_id=path.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var path = new Aws.Ec2.NetworkInsightsPath("path", new()
* {
* Source = source.Id,
* Destination = destination.Id,
* Protocol = "tcp",
* });
* var analysis = new Aws.Ec2.NetworkInsightsAnalysis("analysis", new()
* {
* NetworkInsightsPathId = path.Id,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ec2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* path, err := ec2.NewNetworkInsightsPath(ctx, "path", &ec2.NetworkInsightsPathArgs{
* Source: pulumi.Any(source.Id),
* Destination: pulumi.Any(destination.Id),
* Protocol: pulumi.String("tcp"),
* })
* if err != nil {
* return err
* }
* _, err = ec2.NewNetworkInsightsAnalysis(ctx, "analysis", &ec2.NetworkInsightsAnalysisArgs{
* NetworkInsightsPathId: path.ID(),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.NetworkInsightsPath;
* import com.pulumi.aws.ec2.NetworkInsightsPathArgs;
* import com.pulumi.aws.ec2.NetworkInsightsAnalysis;
* import com.pulumi.aws.ec2.NetworkInsightsAnalysisArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var path = new NetworkInsightsPath("path", NetworkInsightsPathArgs.builder()
* .source(source.id())
* .destination(destination.id())
* .protocol("tcp")
* .build());
* var analysis = new NetworkInsightsAnalysis("analysis", NetworkInsightsAnalysisArgs.builder()
* .networkInsightsPathId(path.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* path:
* type: aws:ec2:NetworkInsightsPath
* properties:
* source: ${source.id}
* destination: ${destination.id}
* protocol: tcp
* analysis:
* type: aws:ec2:NetworkInsightsAnalysis
* properties:
* networkInsightsPathId: ${path.id}
* ```
*
* ## Import
* Using `pulumi import`, import Network Insights Analyzes using the `id`. For example:
* ```sh
* $ pulumi import aws:ec2/networkInsightsAnalysis:NetworkInsightsAnalysis test nia-0462085c957f11a55
* ```
*/
public class NetworkInsightsAnalysis internal constructor(
override val javaResource: com.pulumi.aws.ec2.NetworkInsightsAnalysis,
) : KotlinCustomResource(javaResource, NetworkInsightsAnalysisMapper) {
/**
* Potential intermediate components of a feasible path. Described below.
*/
public val alternatePathHints: Output>
get() = javaResource.alternatePathHints().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> networkInsightsAnalysisAlternatePathHintToKotlin(args0) })
})
})
/**
* ARN of the Network Insights Analysis.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* Explanation codes for an unreachable path. See the [AWS documentation](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_Explanation.html) for details.
*/
public val explanations: Output>
get() = javaResource.explanations().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
networkInsightsAnalysisExplanationToKotlin(args0)
})
})
})
/**
* A list of ARNs for resources the path must traverse.
*/
public val filterInArns: Output>?
get() = javaResource.filterInArns().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
/**
* The components in the path from source to destination. See the [AWS documentation](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_PathComponent.html) for details.
*/
public val forwardPathComponents: Output>
get() = javaResource.forwardPathComponents().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> networkInsightsAnalysisForwardPathComponentToKotlin(args0) })
})
})
/**
* ID of the Network Insights Path to run an analysis on.
* The following arguments are optional:
*/
public val networkInsightsPathId: Output
get() = javaResource.networkInsightsPathId().applyValue({ args0 -> args0 })
/**
* Set to `true` if the destination was reachable.
*/
public val pathFound: Output
get() = javaResource.pathFound().applyValue({ args0 -> args0 })
/**
* The components in the path from destination to source. See the [AWS documentation](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_PathComponent.html) for details.
*/
public val returnPathComponents: Output>
get() = javaResource.returnPathComponents().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> networkInsightsAnalysisReturnPathComponentToKotlin(args0) })
})
})
/**
* The date/time the analysis was started.
*/
public val startDate: Output
get() = javaResource.startDate().applyValue({ args0 -> args0 })
/**
* The status of the analysis. `succeeded` means the analysis was completed, not that a path was found, for that see `path_found`.
*/
public val status: Output
get() = javaResource.status().applyValue({ args0 -> args0 })
/**
* A message to provide more context when the `status` is `failed`.
*/
public val statusMessage: Output
get() = javaResource.statusMessage().applyValue({ args0 -> args0 })
/**
* Map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy