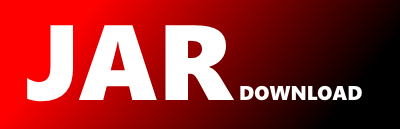
com.pulumi.aws.ec2.kotlin.inputs.AmiCopyEbsBlockDeviceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ec2.kotlin.inputs
import com.pulumi.aws.ec2.inputs.AmiCopyEbsBlockDeviceArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property deleteOnTermination Boolean controlling whether the EBS volumes created to
* support each created instance will be deleted once that instance is terminated.
* @property deviceName Path at which the device is exposed to created instances.
* @property encrypted Boolean controlling whether the created EBS volumes will be encrypted. Can't be used with `snapshot_id`.
* @property iops Number of I/O operations per second the
* created volumes will support.
* @property outpostArn ARN of the Outpost on which the snapshot is stored.
* > **Note:** You can specify `encrypted` or `snapshot_id` but not both.
* @property snapshotId ID of an EBS snapshot that will be used to initialize the created
* EBS volumes. If set, the `volume_size` attribute must be at least as large as the referenced
* snapshot.
* @property throughput Throughput that the EBS volume supports, in MiB/s. Only valid for `volume_type` of `gp3`.
* @property volumeSize Size of created volumes in GiB.
* If `snapshot_id` is set and `volume_size` is omitted then the volume will have the same size
* as the selected snapshot.
* @property volumeType Type of EBS volume to create. Can be `standard`, `gp2`, `gp3`, `io1`, `io2`, `sc1` or `st1` (Default: `standard`).
*/
public data class AmiCopyEbsBlockDeviceArgs(
public val deleteOnTermination: Output? = null,
public val deviceName: Output? = null,
public val encrypted: Output? = null,
public val iops: Output? = null,
public val outpostArn: Output? = null,
public val snapshotId: Output? = null,
public val throughput: Output? = null,
public val volumeSize: Output? = null,
public val volumeType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.ec2.inputs.AmiCopyEbsBlockDeviceArgs =
com.pulumi.aws.ec2.inputs.AmiCopyEbsBlockDeviceArgs.builder()
.deleteOnTermination(deleteOnTermination?.applyValue({ args0 -> args0 }))
.deviceName(deviceName?.applyValue({ args0 -> args0 }))
.encrypted(encrypted?.applyValue({ args0 -> args0 }))
.iops(iops?.applyValue({ args0 -> args0 }))
.outpostArn(outpostArn?.applyValue({ args0 -> args0 }))
.snapshotId(snapshotId?.applyValue({ args0 -> args0 }))
.throughput(throughput?.applyValue({ args0 -> args0 }))
.volumeSize(volumeSize?.applyValue({ args0 -> args0 }))
.volumeType(volumeType?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AmiCopyEbsBlockDeviceArgs].
*/
@PulumiTagMarker
public class AmiCopyEbsBlockDeviceArgsBuilder internal constructor() {
private var deleteOnTermination: Output? = null
private var deviceName: Output? = null
private var encrypted: Output? = null
private var iops: Output? = null
private var outpostArn: Output? = null
private var snapshotId: Output? = null
private var throughput: Output? = null
private var volumeSize: Output? = null
private var volumeType: Output? = null
/**
* @param value Boolean controlling whether the EBS volumes created to
* support each created instance will be deleted once that instance is terminated.
*/
@JvmName("rddrcdliahbfcvlc")
public suspend fun deleteOnTermination(`value`: Output) {
this.deleteOnTermination = value
}
/**
* @param value Path at which the device is exposed to created instances.
*/
@JvmName("avjygpbxvhmgfwjj")
public suspend fun deviceName(`value`: Output) {
this.deviceName = value
}
/**
* @param value Boolean controlling whether the created EBS volumes will be encrypted. Can't be used with `snapshot_id`.
*/
@JvmName("djmeapjtlkfrmxos")
public suspend fun encrypted(`value`: Output) {
this.encrypted = value
}
/**
* @param value Number of I/O operations per second the
* created volumes will support.
*/
@JvmName("rnmxqnidlumpwvhr")
public suspend fun iops(`value`: Output) {
this.iops = value
}
/**
* @param value ARN of the Outpost on which the snapshot is stored.
* > **Note:** You can specify `encrypted` or `snapshot_id` but not both.
*/
@JvmName("hcrpwcsrtvwexwot")
public suspend fun outpostArn(`value`: Output) {
this.outpostArn = value
}
/**
* @param value ID of an EBS snapshot that will be used to initialize the created
* EBS volumes. If set, the `volume_size` attribute must be at least as large as the referenced
* snapshot.
*/
@JvmName("wxyalfncoepsbmlf")
public suspend fun snapshotId(`value`: Output) {
this.snapshotId = value
}
/**
* @param value Throughput that the EBS volume supports, in MiB/s. Only valid for `volume_type` of `gp3`.
*/
@JvmName("ahvvsgsabdmrnnes")
public suspend fun throughput(`value`: Output) {
this.throughput = value
}
/**
* @param value Size of created volumes in GiB.
* If `snapshot_id` is set and `volume_size` is omitted then the volume will have the same size
* as the selected snapshot.
*/
@JvmName("ivnwlysakcstdovf")
public suspend fun volumeSize(`value`: Output) {
this.volumeSize = value
}
/**
* @param value Type of EBS volume to create. Can be `standard`, `gp2`, `gp3`, `io1`, `io2`, `sc1` or `st1` (Default: `standard`).
*/
@JvmName("sxewxtxemdyyspfs")
public suspend fun volumeType(`value`: Output) {
this.volumeType = value
}
/**
* @param value Boolean controlling whether the EBS volumes created to
* support each created instance will be deleted once that instance is terminated.
*/
@JvmName("xypkpvvxxcctgmav")
public suspend fun deleteOnTermination(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deleteOnTermination = mapped
}
/**
* @param value Path at which the device is exposed to created instances.
*/
@JvmName("envaqghoqxuvyjxo")
public suspend fun deviceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deviceName = mapped
}
/**
* @param value Boolean controlling whether the created EBS volumes will be encrypted. Can't be used with `snapshot_id`.
*/
@JvmName("wybuyjwybfvalwiq")
public suspend fun encrypted(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encrypted = mapped
}
/**
* @param value Number of I/O operations per second the
* created volumes will support.
*/
@JvmName("cuywiuewutmambxj")
public suspend fun iops(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.iops = mapped
}
/**
* @param value ARN of the Outpost on which the snapshot is stored.
* > **Note:** You can specify `encrypted` or `snapshot_id` but not both.
*/
@JvmName("jfhmowtrreavsejl")
public suspend fun outpostArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.outpostArn = mapped
}
/**
* @param value ID of an EBS snapshot that will be used to initialize the created
* EBS volumes. If set, the `volume_size` attribute must be at least as large as the referenced
* snapshot.
*/
@JvmName("gnwwdkeomqppptqa")
public suspend fun snapshotId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.snapshotId = mapped
}
/**
* @param value Throughput that the EBS volume supports, in MiB/s. Only valid for `volume_type` of `gp3`.
*/
@JvmName("cqokswsfnvhgirpr")
public suspend fun throughput(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.throughput = mapped
}
/**
* @param value Size of created volumes in GiB.
* If `snapshot_id` is set and `volume_size` is omitted then the volume will have the same size
* as the selected snapshot.
*/
@JvmName("clefkbpagxinydef")
public suspend fun volumeSize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.volumeSize = mapped
}
/**
* @param value Type of EBS volume to create. Can be `standard`, `gp2`, `gp3`, `io1`, `io2`, `sc1` or `st1` (Default: `standard`).
*/
@JvmName("oiavkbitrafhfvqn")
public suspend fun volumeType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.volumeType = mapped
}
internal fun build(): AmiCopyEbsBlockDeviceArgs = AmiCopyEbsBlockDeviceArgs(
deleteOnTermination = deleteOnTermination,
deviceName = deviceName,
encrypted = encrypted,
iops = iops,
outpostArn = outpostArn,
snapshotId = snapshotId,
throughput = throughput,
volumeSize = volumeSize,
volumeType = volumeType,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy