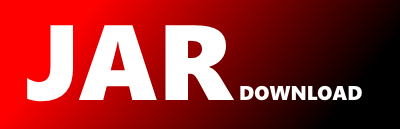
com.pulumi.aws.ec2.kotlin.inputs.FleetSpotOptionsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ec2.kotlin.inputs
import com.pulumi.aws.ec2.inputs.FleetSpotOptionsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property allocationStrategy How to allocate the target capacity across the Spot pools. Valid values: `diversified`, `lowestPrice`, `capacity-optimized`, `capacity-optimized-prioritized` and `price-capacity-optimized`. Default: `lowestPrice`.
* @property instanceInterruptionBehavior Behavior when a Spot Instance is interrupted. Valid values: `hibernate`, `stop`, `terminate`. Default: `terminate`.
* @property instancePoolsToUseCount Number of Spot pools across which to allocate your target Spot capacity. Valid only when Spot `allocation_strategy` is set to `lowestPrice`. Default: `1`.
* @property maintenanceStrategies Nested argument containing maintenance strategies for managing your Spot Instances that are at an elevated risk of being interrupted. Defined below.
*/
public data class FleetSpotOptionsArgs(
public val allocationStrategy: Output? = null,
public val instanceInterruptionBehavior: Output? = null,
public val instancePoolsToUseCount: Output? = null,
public val maintenanceStrategies: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.ec2.inputs.FleetSpotOptionsArgs =
com.pulumi.aws.ec2.inputs.FleetSpotOptionsArgs.builder()
.allocationStrategy(allocationStrategy?.applyValue({ args0 -> args0 }))
.instanceInterruptionBehavior(instanceInterruptionBehavior?.applyValue({ args0 -> args0 }))
.instancePoolsToUseCount(instancePoolsToUseCount?.applyValue({ args0 -> args0 }))
.maintenanceStrategies(
maintenanceStrategies?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [FleetSpotOptionsArgs].
*/
@PulumiTagMarker
public class FleetSpotOptionsArgsBuilder internal constructor() {
private var allocationStrategy: Output? = null
private var instanceInterruptionBehavior: Output? = null
private var instancePoolsToUseCount: Output? = null
private var maintenanceStrategies: Output? = null
/**
* @param value How to allocate the target capacity across the Spot pools. Valid values: `diversified`, `lowestPrice`, `capacity-optimized`, `capacity-optimized-prioritized` and `price-capacity-optimized`. Default: `lowestPrice`.
*/
@JvmName("psduvgplbrwpoxsp")
public suspend fun allocationStrategy(`value`: Output) {
this.allocationStrategy = value
}
/**
* @param value Behavior when a Spot Instance is interrupted. Valid values: `hibernate`, `stop`, `terminate`. Default: `terminate`.
*/
@JvmName("ekhkyokanfnqvwuc")
public suspend fun instanceInterruptionBehavior(`value`: Output) {
this.instanceInterruptionBehavior = value
}
/**
* @param value Number of Spot pools across which to allocate your target Spot capacity. Valid only when Spot `allocation_strategy` is set to `lowestPrice`. Default: `1`.
*/
@JvmName("libgiwcwlsawoops")
public suspend fun instancePoolsToUseCount(`value`: Output) {
this.instancePoolsToUseCount = value
}
/**
* @param value Nested argument containing maintenance strategies for managing your Spot Instances that are at an elevated risk of being interrupted. Defined below.
*/
@JvmName("rvhstbuanalaogmt")
public suspend fun maintenanceStrategies(`value`: Output) {
this.maintenanceStrategies = value
}
/**
* @param value How to allocate the target capacity across the Spot pools. Valid values: `diversified`, `lowestPrice`, `capacity-optimized`, `capacity-optimized-prioritized` and `price-capacity-optimized`. Default: `lowestPrice`.
*/
@JvmName("wdbnutwgcuvfqqor")
public suspend fun allocationStrategy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allocationStrategy = mapped
}
/**
* @param value Behavior when a Spot Instance is interrupted. Valid values: `hibernate`, `stop`, `terminate`. Default: `terminate`.
*/
@JvmName("mdplsojyexghbbyi")
public suspend fun instanceInterruptionBehavior(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.instanceInterruptionBehavior = mapped
}
/**
* @param value Number of Spot pools across which to allocate your target Spot capacity. Valid only when Spot `allocation_strategy` is set to `lowestPrice`. Default: `1`.
*/
@JvmName("srqryurevcboyaeg")
public suspend fun instancePoolsToUseCount(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.instancePoolsToUseCount = mapped
}
/**
* @param value Nested argument containing maintenance strategies for managing your Spot Instances that are at an elevated risk of being interrupted. Defined below.
*/
@JvmName("rkehoripfseuxahm")
public suspend fun maintenanceStrategies(`value`: FleetSpotOptionsMaintenanceStrategiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maintenanceStrategies = mapped
}
/**
* @param argument Nested argument containing maintenance strategies for managing your Spot Instances that are at an elevated risk of being interrupted. Defined below.
*/
@JvmName("rvgriuftpcnbsrwb")
public suspend fun maintenanceStrategies(argument: suspend FleetSpotOptionsMaintenanceStrategiesArgsBuilder.() -> Unit) {
val toBeMapped = FleetSpotOptionsMaintenanceStrategiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.maintenanceStrategies = mapped
}
internal fun build(): FleetSpotOptionsArgs = FleetSpotOptionsArgs(
allocationStrategy = allocationStrategy,
instanceInterruptionBehavior = instanceInterruptionBehavior,
instancePoolsToUseCount = instancePoolsToUseCount,
maintenanceStrategies = maintenanceStrategies,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy