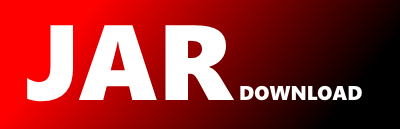
com.pulumi.aws.ec2.kotlin.inputs.GetVpcPeeringConnectionPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ec2.kotlin.inputs
import com.pulumi.aws.ec2.inputs.GetVpcPeeringConnectionPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getVpcPeeringConnection.
* @property cidrBlock Primary CIDR block of the requester VPC of the specific VPC Peering Connection to retrieve.
* @property filters Custom filter block as described below.
* @property id ID of the specific VPC Peering Connection to retrieve.
* @property ownerId AWS account ID of the owner of the requester VPC of the specific VPC Peering Connection to retrieve.
* @property peerCidrBlock Primary CIDR block of the accepter VPC of the specific VPC Peering Connection to retrieve.
* @property peerOwnerId AWS account ID of the owner of the accepter VPC of the specific VPC Peering Connection to retrieve.
* @property peerRegion Region of the accepter VPC of the specific VPC Peering Connection to retrieve.
* @property peerVpcId ID of the accepter VPC of the specific VPC Peering Connection to retrieve.
* @property region Region of the requester VPC of the specific VPC Peering Connection to retrieve.
* @property status Status of the specific VPC Peering Connection to retrieve.
* @property tags Map of tags, each pair of which must exactly match
* a pair on the desired VPC Peering Connection.
* More complex filters can be expressed using one or more `filter` sub-blocks,
* which take the following arguments:
* @property vpcId ID of the requester VPC of the specific VPC Peering Connection to retrieve.
*/
public data class GetVpcPeeringConnectionPlainArgs(
public val cidrBlock: String? = null,
public val filters: List? = null,
public val id: String? = null,
public val ownerId: String? = null,
public val peerCidrBlock: String? = null,
public val peerOwnerId: String? = null,
public val peerRegion: String? = null,
public val peerVpcId: String? = null,
public val region: String? = null,
public val status: String? = null,
public val tags: Map? = null,
public val vpcId: String? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.ec2.inputs.GetVpcPeeringConnectionPlainArgs =
com.pulumi.aws.ec2.inputs.GetVpcPeeringConnectionPlainArgs.builder()
.cidrBlock(cidrBlock?.let({ args0 -> args0 }))
.filters(filters?.let({ args0 -> args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) }) }))
.id(id?.let({ args0 -> args0 }))
.ownerId(ownerId?.let({ args0 -> args0 }))
.peerCidrBlock(peerCidrBlock?.let({ args0 -> args0 }))
.peerOwnerId(peerOwnerId?.let({ args0 -> args0 }))
.peerRegion(peerRegion?.let({ args0 -> args0 }))
.peerVpcId(peerVpcId?.let({ args0 -> args0 }))
.region(region?.let({ args0 -> args0 }))
.status(status?.let({ args0 -> args0 }))
.tags(tags?.let({ args0 -> args0.map({ args0 -> args0.key.to(args0.value) }).toMap() }))
.vpcId(vpcId?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetVpcPeeringConnectionPlainArgs].
*/
@PulumiTagMarker
public class GetVpcPeeringConnectionPlainArgsBuilder internal constructor() {
private var cidrBlock: String? = null
private var filters: List? = null
private var id: String? = null
private var ownerId: String? = null
private var peerCidrBlock: String? = null
private var peerOwnerId: String? = null
private var peerRegion: String? = null
private var peerVpcId: String? = null
private var region: String? = null
private var status: String? = null
private var tags: Map? = null
private var vpcId: String? = null
/**
* @param value Primary CIDR block of the requester VPC of the specific VPC Peering Connection to retrieve.
*/
@JvmName("ottiovumknglbyel")
public suspend fun cidrBlock(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.cidrBlock = mapped
}
/**
* @param value Custom filter block as described below.
*/
@JvmName("ickgbiwbxkdurvvw")
public suspend fun filters(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.filters = mapped
}
/**
* @param argument Custom filter block as described below.
*/
@JvmName("fbobwlhwpqyrcnrd")
public suspend fun filters(argument: List Unit>) {
val toBeMapped = argument.toList().map {
GetVpcPeeringConnectionFilterBuilder().applySuspend {
it()
}.build()
}
val mapped = toBeMapped
this.filters = mapped
}
/**
* @param argument Custom filter block as described below.
*/
@JvmName("pagmenhpstidbnrw")
public suspend fun filters(vararg argument: suspend GetVpcPeeringConnectionFilterBuilder.() -> Unit) {
val toBeMapped = argument.toList().map {
GetVpcPeeringConnectionFilterBuilder().applySuspend {
it()
}.build()
}
val mapped = toBeMapped
this.filters = mapped
}
/**
* @param argument Custom filter block as described below.
*/
@JvmName("ljiwpnsoiwcdhtfl")
public suspend fun filters(argument: suspend GetVpcPeeringConnectionFilterBuilder.() -> Unit) {
val toBeMapped = listOf(
GetVpcPeeringConnectionFilterBuilder().applySuspend {
argument()
}.build(),
)
val mapped = toBeMapped
this.filters = mapped
}
/**
* @param values Custom filter block as described below.
*/
@JvmName("rxppqtbobcqjwpwa")
public suspend fun filters(vararg values: GetVpcPeeringConnectionFilter) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.filters = mapped
}
/**
* @param value ID of the specific VPC Peering Connection to retrieve.
*/
@JvmName("jxxefyfwyblhtdgv")
public suspend fun id(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.id = mapped
}
/**
* @param value AWS account ID of the owner of the requester VPC of the specific VPC Peering Connection to retrieve.
*/
@JvmName("eatwflksesuhieku")
public suspend fun ownerId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.ownerId = mapped
}
/**
* @param value Primary CIDR block of the accepter VPC of the specific VPC Peering Connection to retrieve.
*/
@JvmName("jqquqoywtykdfwmm")
public suspend fun peerCidrBlock(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.peerCidrBlock = mapped
}
/**
* @param value AWS account ID of the owner of the accepter VPC of the specific VPC Peering Connection to retrieve.
*/
@JvmName("wwabblxuowilgfrt")
public suspend fun peerOwnerId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.peerOwnerId = mapped
}
/**
* @param value Region of the accepter VPC of the specific VPC Peering Connection to retrieve.
*/
@JvmName("rlmlvsgyiiwbfwnc")
public suspend fun peerRegion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.peerRegion = mapped
}
/**
* @param value ID of the accepter VPC of the specific VPC Peering Connection to retrieve.
*/
@JvmName("vkaxkkanuvdpueco")
public suspend fun peerVpcId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.peerVpcId = mapped
}
/**
* @param value Region of the requester VPC of the specific VPC Peering Connection to retrieve.
*/
@JvmName("ybxnypecouayiqhy")
public suspend fun region(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.region = mapped
}
/**
* @param value Status of the specific VPC Peering Connection to retrieve.
*/
@JvmName("lelalpxlfvlitaag")
public suspend fun status(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.status = mapped
}
/**
* @param value Map of tags, each pair of which must exactly match
* a pair on the desired VPC Peering Connection.
* More complex filters can be expressed using one or more `filter` sub-blocks,
* which take the following arguments:
*/
@JvmName("vftjjlbyskebtplk")
public suspend fun tags(`value`: Map?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.tags = mapped
}
/**
* @param values Map of tags, each pair of which must exactly match
* a pair on the desired VPC Peering Connection.
* More complex filters can be expressed using one or more `filter` sub-blocks,
* which take the following arguments:
*/
@JvmName("tthdxoyhmtduicdk")
public fun tags(vararg values: Pair) {
val toBeMapped = values.toMap()
val mapped = toBeMapped.let({ args0 -> args0 })
this.tags = mapped
}
/**
* @param value ID of the requester VPC of the specific VPC Peering Connection to retrieve.
*/
@JvmName("bpumtvtavsyeepgb")
public suspend fun vpcId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.vpcId = mapped
}
internal fun build(): GetVpcPeeringConnectionPlainArgs = GetVpcPeeringConnectionPlainArgs(
cidrBlock = cidrBlock,
filters = filters,
id = id,
ownerId = ownerId,
peerCidrBlock = peerCidrBlock,
peerOwnerId = peerOwnerId,
peerRegion = peerRegion,
peerVpcId = peerVpcId,
region = region,
status = status,
tags = tags,
vpcId = vpcId,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy