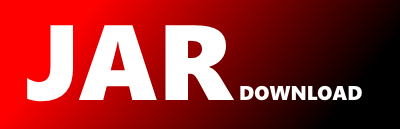
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanation.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ec2.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property aclRules
* @property acls
* @property address
* @property addresses
* @property attachedTos
* @property availabilityZones
* @property cidrs
* @property classicLoadBalancerListeners
* @property components
* @property customerGateways
* @property destinationVpcs
* @property destinations
* @property direction
* @property elasticLoadBalancerListeners
* @property explanationCode
* @property ingressRouteTables
* @property internetGateways
* @property loadBalancerArn
* @property loadBalancerListenerPort
* @property loadBalancerTargetGroup
* @property loadBalancerTargetGroups
* @property loadBalancerTargetPort
* @property missingComponent
* @property natGateways
* @property networkInterfaces
* @property packetField
* @property port
* @property portRanges
* @property prefixLists
* @property protocols
* @property routeTableRoutes
* @property routeTables
* @property securityGroup
* @property securityGroupRules
* @property securityGroups
* @property sourceVpcs
* @property state
* @property subnetRouteTables
* @property subnets
* @property transitGatewayAttachments
* @property transitGatewayRouteTableRoutes
* @property transitGatewayRouteTables
* @property transitGateways
* @property vpcEndpoints
* @property vpcPeeringConnections
* @property vpcs
* @property vpnConnections
* @property vpnGateways
*/
public data class GetNetworkInsightsAnalysisExplanation(
public val aclRules: List,
public val acls: List,
public val address: String,
public val addresses: List,
public val attachedTos: List,
public val availabilityZones: List,
public val cidrs: List,
public val classicLoadBalancerListeners: List,
public val components: List,
public val customerGateways: List,
public val destinationVpcs: List,
public val destinations: List,
public val direction: String,
public val elasticLoadBalancerListeners: List,
public val explanationCode: String,
public val ingressRouteTables: List,
public val internetGateways: List,
public val loadBalancerArn: String,
public val loadBalancerListenerPort: Int,
public val loadBalancerTargetGroup: List,
public val loadBalancerTargetGroups: List,
public val loadBalancerTargetPort: Int,
public val missingComponent: String,
public val natGateways: List,
public val networkInterfaces: List,
public val packetField: String,
public val port: Int,
public val portRanges: List,
public val prefixLists: List,
public val protocols: List,
public val routeTableRoutes: List,
public val routeTables: List,
public val securityGroup: List,
public val securityGroupRules: List,
public val securityGroups: List,
public val sourceVpcs: List,
public val state: String,
public val subnetRouteTables: List,
public val subnets: List,
public val transitGatewayAttachments: List,
public val transitGatewayRouteTableRoutes: List,
public val transitGatewayRouteTables: List,
public val transitGateways: List,
public val vpcEndpoints: List,
public val vpcPeeringConnections: List,
public val vpcs: List,
public val vpnConnections: List,
public val vpnGateways: List,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.ec2.outputs.GetNetworkInsightsAnalysisExplanation): GetNetworkInsightsAnalysisExplanation = GetNetworkInsightsAnalysisExplanation(
aclRules = javaType.aclRules().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationAclRule.Companion.toKotlin(args0)
})
}),
acls = javaType.acls().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationAcl.Companion.toKotlin(args0)
})
}),
address = javaType.address(),
addresses = javaType.addresses().map({ args0 -> args0 }),
attachedTos = javaType.attachedTos().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationAttachedTo.Companion.toKotlin(args0)
})
}),
availabilityZones = javaType.availabilityZones().map({ args0 -> args0 }),
cidrs = javaType.cidrs().map({ args0 -> args0 }),
classicLoadBalancerListeners = javaType.classicLoadBalancerListeners().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationClassicLoadBalancerListener.Companion.toKotlin(args0)
})
}),
components = javaType.components().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationComponent.Companion.toKotlin(args0)
})
}),
customerGateways = javaType.customerGateways().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationCustomerGateway.Companion.toKotlin(args0)
})
}),
destinationVpcs = javaType.destinationVpcs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationDestinationVpc.Companion.toKotlin(args0)
})
}),
destinations = javaType.destinations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationDestination.Companion.toKotlin(args0)
})
}),
direction = javaType.direction(),
elasticLoadBalancerListeners = javaType.elasticLoadBalancerListeners().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationElasticLoadBalancerListener.Companion.toKotlin(args0)
})
}),
explanationCode = javaType.explanationCode(),
ingressRouteTables = javaType.ingressRouteTables().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationIngressRouteTable.Companion.toKotlin(args0)
})
}),
internetGateways = javaType.internetGateways().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationInternetGateway.Companion.toKotlin(args0)
})
}),
loadBalancerArn = javaType.loadBalancerArn(),
loadBalancerListenerPort = javaType.loadBalancerListenerPort(),
loadBalancerTargetGroup = javaType.loadBalancerTargetGroup().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationLoadBalancerTargetGroup.Companion.toKotlin(args0)
})
}),
loadBalancerTargetGroups = javaType.loadBalancerTargetGroups().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationLoadBalancerTargetGroup.Companion.toKotlin(args0)
})
}),
loadBalancerTargetPort = javaType.loadBalancerTargetPort(),
missingComponent = javaType.missingComponent(),
natGateways = javaType.natGateways().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationNatGateway.Companion.toKotlin(args0)
})
}),
networkInterfaces = javaType.networkInterfaces().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationNetworkInterface.Companion.toKotlin(args0)
})
}),
packetField = javaType.packetField(),
port = javaType.port(),
portRanges = javaType.portRanges().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationPortRange.Companion.toKotlin(args0)
})
}),
prefixLists = javaType.prefixLists().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationPrefixList.Companion.toKotlin(args0)
})
}),
protocols = javaType.protocols().map({ args0 -> args0 }),
routeTableRoutes = javaType.routeTableRoutes().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationRouteTableRoute.Companion.toKotlin(args0)
})
}),
routeTables = javaType.routeTables().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationRouteTable.Companion.toKotlin(args0)
})
}),
securityGroup = javaType.securityGroup().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationSecurityGroup.Companion.toKotlin(args0)
})
}),
securityGroupRules = javaType.securityGroupRules().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationSecurityGroupRule.Companion.toKotlin(args0)
})
}),
securityGroups = javaType.securityGroups().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationSecurityGroup.Companion.toKotlin(args0)
})
}),
sourceVpcs = javaType.sourceVpcs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationSourceVpc.Companion.toKotlin(args0)
})
}),
state = javaType.state(),
subnetRouteTables = javaType.subnetRouteTables().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationSubnetRouteTable.Companion.toKotlin(args0)
})
}),
subnets = javaType.subnets().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationSubnet.Companion.toKotlin(args0)
})
}),
transitGatewayAttachments = javaType.transitGatewayAttachments().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationTransitGatewayAttachment.Companion.toKotlin(args0)
})
}),
transitGatewayRouteTableRoutes = javaType.transitGatewayRouteTableRoutes().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationTransitGatewayRouteTableRoute.Companion.toKotlin(args0)
})
}),
transitGatewayRouteTables = javaType.transitGatewayRouteTables().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationTransitGatewayRouteTable.Companion.toKotlin(args0)
})
}),
transitGateways = javaType.transitGateways().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationTransitGateway.Companion.toKotlin(args0)
})
}),
vpcEndpoints = javaType.vpcEndpoints().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationVpcEndpoint.Companion.toKotlin(args0)
})
}),
vpcPeeringConnections = javaType.vpcPeeringConnections().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationVpcPeeringConnection.Companion.toKotlin(args0)
})
}),
vpcs = javaType.vpcs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationVpc.Companion.toKotlin(args0)
})
}),
vpnConnections = javaType.vpnConnections().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationVpnConnection.Companion.toKotlin(args0)
})
}),
vpnGateways = javaType.vpnGateways().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInsightsAnalysisExplanationVpnGateway.Companion.toKotlin(args0)
})
}),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy