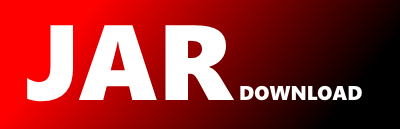
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInterfaceResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ec2.kotlin.outputs
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getNetworkInterface.
* @property arn ARN of the network interface.
* @property associations Association information for an Elastic IP address (IPv4) associated with the network interface. See supported fields below.
* @property attachments
* @property availabilityZone Availability Zone.
* @property description Description of the network interface.
* @property filters
* @property id
* @property interfaceType Type of interface.
* @property ipv6Addresses List of IPv6 addresses to assign to the ENI.
* @property macAddress MAC address.
* @property outpostArn ARN of the Outpost.
* @property ownerId AWS account ID of the owner of the network interface.
* @property privateDnsName Private DNS name.
* @property privateIp Private IPv4 address of the network interface within the subnet.
* @property privateIps Private IPv4 addresses associated with the network interface.
* @property requesterId ID of the entity that launched the instance on your behalf.
* @property securityGroups List of security groups for the network interface.
* @property subnetId ID of the subnet.
* @property tags Any tags assigned to the network interface.
* @property vpcId ID of the VPC.
*/
public data class GetNetworkInterfaceResult(
public val arn: String,
public val associations: List,
public val attachments: List,
public val availabilityZone: String,
public val description: String,
public val filters: List? = null,
public val id: String,
public val interfaceType: String,
public val ipv6Addresses: List,
public val macAddress: String,
public val outpostArn: String,
public val ownerId: String,
public val privateDnsName: String,
public val privateIp: String,
public val privateIps: List,
public val requesterId: String,
public val securityGroups: List,
public val subnetId: String,
public val tags: Map,
public val vpcId: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.ec2.outputs.GetNetworkInterfaceResult): GetNetworkInterfaceResult = GetNetworkInterfaceResult(
arn = javaType.arn(),
associations = javaType.associations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInterfaceAssociation.Companion.toKotlin(args0)
})
}),
attachments = javaType.attachments().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInterfaceAttachment.Companion.toKotlin(args0)
})
}),
availabilityZone = javaType.availabilityZone(),
description = javaType.description(),
filters = javaType.filters().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetNetworkInterfaceFilter.Companion.toKotlin(args0)
})
}),
id = javaType.id(),
interfaceType = javaType.interfaceType(),
ipv6Addresses = javaType.ipv6Addresses().map({ args0 -> args0 }),
macAddress = javaType.macAddress(),
outpostArn = javaType.outpostArn(),
ownerId = javaType.ownerId(),
privateDnsName = javaType.privateDnsName(),
privateIp = javaType.privateIp(),
privateIps = javaType.privateIps().map({ args0 -> args0 }),
requesterId = javaType.requesterId(),
securityGroups = javaType.securityGroups().map({ args0 -> args0 }),
subnetId = javaType.subnetId(),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
vpcId = javaType.vpcId(),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy