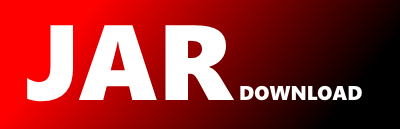
com.pulumi.aws.ec2.kotlin.outputs.GetVpcEndpointResult.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ec2.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getVpcEndpoint.
* @property arn ARN of the VPC endpoint.
* @property cidrBlocks List of CIDR blocks for the exposed AWS service. Applicable for endpoints of type `Gateway`.
* @property dnsEntries DNS entries for the VPC Endpoint. Applicable for endpoints of type `Interface`. DNS entry blocks are documented below.
* @property dnsOptions DNS options for the VPC Endpoint. DNS options blocks are documented below.
* @property filters
* @property id
* @property ipAddressType
* @property networkInterfaceIds One or more network interfaces for the VPC Endpoint. Applicable for endpoints of type `Interface`.
* @property ownerId ID of the AWS account that owns the VPC endpoint.
* @property policy Policy document associated with the VPC Endpoint. Applicable for endpoints of type `Gateway`.
* @property prefixListId Prefix list ID of the exposed AWS service. Applicable for endpoints of type `Gateway`.
* @property privateDnsEnabled Whether or not the VPC is associated with a private hosted zone - `true` or `false`. Applicable for endpoints of type `Interface`.
* @property requesterManaged Whether or not the VPC Endpoint is being managed by its service - `true` or `false`.
* @property routeTableIds One or more route tables associated with the VPC Endpoint. Applicable for endpoints of type `Gateway`.
* @property securityGroupIds One or more security groups associated with the network interfaces. Applicable for endpoints of type `Interface`.
* @property serviceName
* @property state
* @property subnetIds One or more subnets in which the VPC Endpoint is located. Applicable for endpoints of type `Interface`.
* @property tags
* @property vpcEndpointType VPC Endpoint type, `Gateway` or `Interface`.
* @property vpcId
*/
public data class GetVpcEndpointResult(
public val arn: String,
public val cidrBlocks: List,
public val dnsEntries: List,
public val dnsOptions: List,
public val filters: List? = null,
public val id: String,
public val ipAddressType: String,
public val networkInterfaceIds: List,
public val ownerId: String,
public val policy: String,
public val prefixListId: String,
public val privateDnsEnabled: Boolean,
public val requesterManaged: Boolean,
public val routeTableIds: List,
public val securityGroupIds: List,
public val serviceName: String,
public val state: String,
public val subnetIds: List,
public val tags: Map,
public val vpcEndpointType: String,
public val vpcId: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.ec2.outputs.GetVpcEndpointResult): GetVpcEndpointResult = GetVpcEndpointResult(
arn = javaType.arn(),
cidrBlocks = javaType.cidrBlocks().map({ args0 -> args0 }),
dnsEntries = javaType.dnsEntries().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetVpcEndpointDnsEntry.Companion.toKotlin(args0)
})
}),
dnsOptions = javaType.dnsOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetVpcEndpointDnsOption.Companion.toKotlin(args0)
})
}),
filters = javaType.filters().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetVpcEndpointFilter.Companion.toKotlin(args0)
})
}),
id = javaType.id(),
ipAddressType = javaType.ipAddressType(),
networkInterfaceIds = javaType.networkInterfaceIds().map({ args0 -> args0 }),
ownerId = javaType.ownerId(),
policy = javaType.policy(),
prefixListId = javaType.prefixListId(),
privateDnsEnabled = javaType.privateDnsEnabled(),
requesterManaged = javaType.requesterManaged(),
routeTableIds = javaType.routeTableIds().map({ args0 -> args0 }),
securityGroupIds = javaType.securityGroupIds().map({ args0 -> args0 }),
serviceName = javaType.serviceName(),
state = javaType.state(),
subnetIds = javaType.subnetIds().map({ args0 -> args0 }),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
vpcEndpointType = javaType.vpcEndpointType(),
vpcId = javaType.vpcId(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy