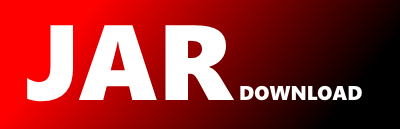
com.pulumi.aws.ec2.kotlin.outputs.GetVpcPeeringConnectionResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ec2.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getVpcPeeringConnection.
* @property accepter Configuration block that describes [VPC Peering Connection]
* (https://docs.aws.amazon.com/vpc/latest/peering/what-is-vpc-peering.html) options set for the accepter VPC.
* @property cidrBlock CIDR block associated to the VPC of the specific VPC Peering Connection.
* @property cidrBlockSets List of objects with IPv4 CIDR blocks of the requester VPC.
* @property filters
* @property id
* @property ipv6CidrBlockSets List of objects with IPv6 CIDR blocks of the requester VPC.
* @property ownerId
* @property peerCidrBlock
* @property peerCidrBlockSets List of objects with IPv4 CIDR blocks of the accepter VPC.
* @property peerIpv6CidrBlockSets List of objects with IPv6 CIDR blocks of the accepter VPC.
* @property peerOwnerId
* @property peerRegion
* @property peerVpcId
* @property region
* @property requester Configuration block that describes [VPC Peering Connection]
* (https://docs.aws.amazon.com/vpc/latest/peering/what-is-vpc-peering.html) options set for the requester VPC.
* @property status
* @property tags
* @property vpcId
*/
public data class GetVpcPeeringConnectionResult(
public val accepter: Map,
public val cidrBlock: String,
public val cidrBlockSets: List,
public val filters: List? = null,
public val id: String,
public val ipv6CidrBlockSets: List,
public val ownerId: String,
public val peerCidrBlock: String,
public val peerCidrBlockSets: List,
public val peerIpv6CidrBlockSets: List,
public val peerOwnerId: String,
public val peerRegion: String,
public val peerVpcId: String,
public val region: String,
public val requester: Map,
public val status: String,
public val tags: Map,
public val vpcId: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.ec2.outputs.GetVpcPeeringConnectionResult): GetVpcPeeringConnectionResult = GetVpcPeeringConnectionResult(
accepter = javaType.accepter().map({ args0 -> args0.key.to(args0.value) }).toMap(),
cidrBlock = javaType.cidrBlock(),
cidrBlockSets = javaType.cidrBlockSets().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetVpcPeeringConnectionCidrBlockSet.Companion.toKotlin(args0)
})
}),
filters = javaType.filters().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetVpcPeeringConnectionFilter.Companion.toKotlin(args0)
})
}),
id = javaType.id(),
ipv6CidrBlockSets = javaType.ipv6CidrBlockSets().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetVpcPeeringConnectionIpv6CidrBlockSet.Companion.toKotlin(args0)
})
}),
ownerId = javaType.ownerId(),
peerCidrBlock = javaType.peerCidrBlock(),
peerCidrBlockSets = javaType.peerCidrBlockSets().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetVpcPeeringConnectionPeerCidrBlockSet.Companion.toKotlin(args0)
})
}),
peerIpv6CidrBlockSets = javaType.peerIpv6CidrBlockSets().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.ec2.kotlin.outputs.GetVpcPeeringConnectionPeerIpv6CidrBlockSet.Companion.toKotlin(args0)
})
}),
peerOwnerId = javaType.peerOwnerId(),
peerRegion = javaType.peerRegion(),
peerVpcId = javaType.peerVpcId(),
region = javaType.region(),
requester = javaType.requester().map({ args0 -> args0.key.to(args0.value) }).toMap(),
status = javaType.status(),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
vpcId = javaType.vpcId(),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy