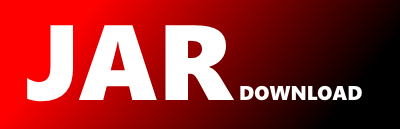
com.pulumi.aws.ecr.kotlin.LifecyclePolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ecr.kotlin
import com.pulumi.aws.ecr.LifecyclePolicyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages an ECR repository lifecycle policy.
* > **NOTE:** Only one `aws.ecr.LifecyclePolicy` resource can be used with the same ECR repository. To apply multiple rules, they must be combined in the `policy` JSON.
* > **NOTE:** The AWS ECR API seems to reorder rules based on `rulePriority`. If you define multiple rules that are not sorted in ascending `rulePriority` order in the this provider code, the resource will be flagged for recreation every deployment.
* ## Example Usage
* ### Policy on untagged image
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.ecr.Repository("example", {name: "example-repo"});
* const exampleLifecyclePolicy = new aws.ecr.LifecyclePolicy("example", {
* repository: example.name,
* policy: `{
* "rules": [
* {
* "rulePriority": 1,
* "description": "Expire images older than 14 days",
* "selection": {
* "tagStatus": "untagged",
* "countType": "sinceImagePushed",
* "countUnit": "days",
* "countNumber": 14
* },
* "action": {
* "type": "expire"
* }
* }
* ]
* }
* `,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.ecr.Repository("example", name="example-repo")
* example_lifecycle_policy = aws.ecr.LifecyclePolicy("example",
* repository=example.name,
* policy="""{
* "rules": [
* {
* "rulePriority": 1,
* "description": "Expire images older than 14 days",
* "selection": {
* "tagStatus": "untagged",
* "countType": "sinceImagePushed",
* "countUnit": "days",
* "countNumber": 14
* },
* "action": {
* "type": "expire"
* }
* }
* ]
* }
* """)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Ecr.Repository("example", new()
* {
* Name = "example-repo",
* });
* var exampleLifecyclePolicy = new Aws.Ecr.LifecyclePolicy("example", new()
* {
* Repository = example.Name,
* Policy = @"{
* ""rules"": [
* {
* ""rulePriority"": 1,
* ""description"": ""Expire images older than 14 days"",
* ""selection"": {
* ""tagStatus"": ""untagged"",
* ""countType"": ""sinceImagePushed"",
* ""countUnit"": ""days"",
* ""countNumber"": 14
* },
* ""action"": {
* ""type"": ""expire""
* }
* }
* ]
* }
* ",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ecr"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := ecr.NewRepository(ctx, "example", &ecr.RepositoryArgs{
* Name: pulumi.String("example-repo"),
* })
* if err != nil {
* return err
* }
* _, err = ecr.NewLifecyclePolicy(ctx, "example", &ecr.LifecyclePolicyArgs{
* Repository: example.Name,
* Policy: pulumi.Any(`{
* "rules": [
* {
* "rulePriority": 1,
* "description": "Expire images older than 14 days",
* "selection": {
* "tagStatus": "untagged",
* "countType": "sinceImagePushed",
* "countUnit": "days",
* "countNumber": 14
* },
* "action": {
* "type": "expire"
* }
* }
* ]
* }
* `),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ecr.Repository;
* import com.pulumi.aws.ecr.RepositoryArgs;
* import com.pulumi.aws.ecr.LifecyclePolicy;
* import com.pulumi.aws.ecr.LifecyclePolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Repository("example", RepositoryArgs.builder()
* .name("example-repo")
* .build());
* var exampleLifecyclePolicy = new LifecyclePolicy("exampleLifecyclePolicy", LifecyclePolicyArgs.builder()
* .repository(example.name())
* .policy("""
* {
* "rules": [
* {
* "rulePriority": 1,
* "description": "Expire images older than 14 days",
* "selection": {
* "tagStatus": "untagged",
* "countType": "sinceImagePushed",
* "countUnit": "days",
* "countNumber": 14
* },
* "action": {
* "type": "expire"
* }
* }
* ]
* }
* """)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:ecr:Repository
* properties:
* name: example-repo
* exampleLifecyclePolicy:
* type: aws:ecr:LifecyclePolicy
* name: example
* properties:
* repository: ${example.name}
* policy: |
* {
* "rules": [
* {
* "rulePriority": 1,
* "description": "Expire images older than 14 days",
* "selection": {
* "tagStatus": "untagged",
* "countType": "sinceImagePushed",
* "countUnit": "days",
* "countNumber": 14
* },
* "action": {
* "type": "expire"
* }
* }
* ]
* }
* ```
*
* ### Policy on tagged image
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.ecr.Repository("example", {name: "example-repo"});
* const exampleLifecyclePolicy = new aws.ecr.LifecyclePolicy("example", {
* repository: example.name,
* policy: `{
* "rules": [
* {
* "rulePriority": 1,
* "description": "Keep last 30 images",
* "selection": {
* "tagStatus": "tagged",
* "tagPrefixList": ["v"],
* "countType": "imageCountMoreThan",
* "countNumber": 30
* },
* "action": {
* "type": "expire"
* }
* }
* ]
* }
* `,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.ecr.Repository("example", name="example-repo")
* example_lifecycle_policy = aws.ecr.LifecyclePolicy("example",
* repository=example.name,
* policy="""{
* "rules": [
* {
* "rulePriority": 1,
* "description": "Keep last 30 images",
* "selection": {
* "tagStatus": "tagged",
* "tagPrefixList": ["v"],
* "countType": "imageCountMoreThan",
* "countNumber": 30
* },
* "action": {
* "type": "expire"
* }
* }
* ]
* }
* """)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Ecr.Repository("example", new()
* {
* Name = "example-repo",
* });
* var exampleLifecyclePolicy = new Aws.Ecr.LifecyclePolicy("example", new()
* {
* Repository = example.Name,
* Policy = @"{
* ""rules"": [
* {
* ""rulePriority"": 1,
* ""description"": ""Keep last 30 images"",
* ""selection"": {
* ""tagStatus"": ""tagged"",
* ""tagPrefixList"": [""v""],
* ""countType"": ""imageCountMoreThan"",
* ""countNumber"": 30
* },
* ""action"": {
* ""type"": ""expire""
* }
* }
* ]
* }
* ",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ecr"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := ecr.NewRepository(ctx, "example", &ecr.RepositoryArgs{
* Name: pulumi.String("example-repo"),
* })
* if err != nil {
* return err
* }
* _, err = ecr.NewLifecyclePolicy(ctx, "example", &ecr.LifecyclePolicyArgs{
* Repository: example.Name,
* Policy: pulumi.Any(`{
* "rules": [
* {
* "rulePriority": 1,
* "description": "Keep last 30 images",
* "selection": {
* "tagStatus": "tagged",
* "tagPrefixList": ["v"],
* "countType": "imageCountMoreThan",
* "countNumber": 30
* },
* "action": {
* "type": "expire"
* }
* }
* ]
* }
* `),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ecr.Repository;
* import com.pulumi.aws.ecr.RepositoryArgs;
* import com.pulumi.aws.ecr.LifecyclePolicy;
* import com.pulumi.aws.ecr.LifecyclePolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Repository("example", RepositoryArgs.builder()
* .name("example-repo")
* .build());
* var exampleLifecyclePolicy = new LifecyclePolicy("exampleLifecyclePolicy", LifecyclePolicyArgs.builder()
* .repository(example.name())
* .policy("""
* {
* "rules": [
* {
* "rulePriority": 1,
* "description": "Keep last 30 images",
* "selection": {
* "tagStatus": "tagged",
* "tagPrefixList": ["v"],
* "countType": "imageCountMoreThan",
* "countNumber": 30
* },
* "action": {
* "type": "expire"
* }
* }
* ]
* }
* """)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:ecr:Repository
* properties:
* name: example-repo
* exampleLifecyclePolicy:
* type: aws:ecr:LifecyclePolicy
* name: example
* properties:
* repository: ${example.name}
* policy: |
* {
* "rules": [
* {
* "rulePriority": 1,
* "description": "Keep last 30 images",
* "selection": {
* "tagStatus": "tagged",
* "tagPrefixList": ["v"],
* "countType": "imageCountMoreThan",
* "countNumber": 30
* },
* "action": {
* "type": "expire"
* }
* }
* ]
* }
* ```
*
* ## Import
* Using `pulumi import`, import ECR Lifecycle Policy using the name of the repository. For example:
* ```sh
* $ pulumi import aws:ecr/lifecyclePolicy:LifecyclePolicy example tf-example
* ```
* @property policy The policy document. This is a JSON formatted string. See more details about [Policy Parameters](http://docs.aws.amazon.com/AmazonECR/latest/userguide/LifecyclePolicies.html#lifecycle_policy_parameters) in the official AWS docs. Consider using the `aws.ecr.getLifecyclePolicyDocument` data_source to generate/manage the JSON document used for the `policy` argument.
* @property repository Name of the repository to apply the policy.
*/
public data class LifecyclePolicyArgs(
public val policy: Output? = null,
public val repository: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.ecr.LifecyclePolicyArgs =
com.pulumi.aws.ecr.LifecyclePolicyArgs.builder()
.policy(policy?.applyValue({ args0 -> args0 }))
.repository(repository?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LifecyclePolicyArgs].
*/
@PulumiTagMarker
public class LifecyclePolicyArgsBuilder internal constructor() {
private var policy: Output? = null
private var repository: Output? = null
/**
* @param value The policy document. This is a JSON formatted string. See more details about [Policy Parameters](http://docs.aws.amazon.com/AmazonECR/latest/userguide/LifecyclePolicies.html#lifecycle_policy_parameters) in the official AWS docs. Consider using the `aws.ecr.getLifecyclePolicyDocument` data_source to generate/manage the JSON document used for the `policy` argument.
*/
@JvmName("njennluhvodruqqr")
public suspend fun policy(`value`: Output) {
this.policy = value
}
/**
* @param value Name of the repository to apply the policy.
*/
@JvmName("wnyqkipqlfsepgbk")
public suspend fun repository(`value`: Output) {
this.repository = value
}
/**
* @param value The policy document. This is a JSON formatted string. See more details about [Policy Parameters](http://docs.aws.amazon.com/AmazonECR/latest/userguide/LifecyclePolicies.html#lifecycle_policy_parameters) in the official AWS docs. Consider using the `aws.ecr.getLifecyclePolicyDocument` data_source to generate/manage the JSON document used for the `policy` argument.
*/
@JvmName("artfjsrnjatdhtog")
public suspend fun policy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.policy = mapped
}
/**
* @param value Name of the repository to apply the policy.
*/
@JvmName("rvdpeurkkkprflgc")
public suspend fun repository(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.repository = mapped
}
internal fun build(): LifecyclePolicyArgs = LifecyclePolicyArgs(
policy = policy,
repository = repository,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy