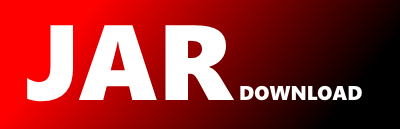
com.pulumi.aws.ecr.kotlin.inputs.GetLifecyclePolicyDocumentRule.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ecr.kotlin.inputs
import com.pulumi.aws.ecr.inputs.GetLifecyclePolicyDocumentRule.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property action Specifies the action type.
* @property description Describes the purpose of a rule within a lifecycle policy.
* @property priority Sets the order in which rules are evaluated, lowest to highest. When you add rules to a lifecycle policy, you must give them each a unique value for `priority`. Values do not need to be sequential across rules in a policy. A rule with a `tag_status` value of "any" must have the highest value for `priority` and be evaluated last.
* @property selection Collects parameters describing the selection criteria for the ECR lifecycle policy:
*/
public data class GetLifecyclePolicyDocumentRule(
public val action: GetLifecyclePolicyDocumentRuleAction? = null,
public val description: String? = null,
public val priority: Int,
public val selection: GetLifecyclePolicyDocumentRuleSelection? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.ecr.inputs.GetLifecyclePolicyDocumentRule =
com.pulumi.aws.ecr.inputs.GetLifecyclePolicyDocumentRule.builder()
.action(action?.let({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.description(description?.let({ args0 -> args0 }))
.priority(priority.let({ args0 -> args0 }))
.selection(selection?.let({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [GetLifecyclePolicyDocumentRule].
*/
@PulumiTagMarker
public class GetLifecyclePolicyDocumentRuleBuilder internal constructor() {
private var action: GetLifecyclePolicyDocumentRuleAction? = null
private var description: String? = null
private var priority: Int? = null
private var selection: GetLifecyclePolicyDocumentRuleSelection? = null
/**
* @param value Specifies the action type.
*/
@JvmName("pdmqcosgsickrwyd")
public suspend fun action(`value`: GetLifecyclePolicyDocumentRuleAction?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.action = mapped
}
/**
* @param argument Specifies the action type.
*/
@JvmName("ahtuugvwnfnywynk")
public suspend fun action(argument: suspend GetLifecyclePolicyDocumentRuleActionBuilder.() -> Unit) {
val toBeMapped = GetLifecyclePolicyDocumentRuleActionBuilder().applySuspend {
argument()
}.build()
val mapped = toBeMapped
this.action = mapped
}
/**
* @param value Describes the purpose of a rule within a lifecycle policy.
*/
@JvmName("mjwwagiclnrphugg")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.description = mapped
}
/**
* @param value Sets the order in which rules are evaluated, lowest to highest. When you add rules to a lifecycle policy, you must give them each a unique value for `priority`. Values do not need to be sequential across rules in a policy. A rule with a `tag_status` value of "any" must have the highest value for `priority` and be evaluated last.
*/
@JvmName("lnbunkjfesxkkyry")
public suspend fun priority(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.priority = mapped
}
/**
* @param value Collects parameters describing the selection criteria for the ECR lifecycle policy:
*/
@JvmName("pahxkjndrmvmjyte")
public suspend fun selection(`value`: GetLifecyclePolicyDocumentRuleSelection?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.selection = mapped
}
/**
* @param argument Collects parameters describing the selection criteria for the ECR lifecycle policy:
*/
@JvmName("cwhhnitaoydpcmqy")
public suspend fun selection(argument: suspend GetLifecyclePolicyDocumentRuleSelectionBuilder.() -> Unit) {
val toBeMapped = GetLifecyclePolicyDocumentRuleSelectionBuilder().applySuspend {
argument()
}.build()
val mapped = toBeMapped
this.selection = mapped
}
internal fun build(): GetLifecyclePolicyDocumentRule = GetLifecyclePolicyDocumentRule(
action = action,
description = description,
priority = priority ?: throw PulumiNullFieldException("priority"),
selection = selection,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy