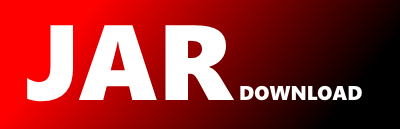
com.pulumi.aws.ecr.kotlin.inputs.GetLifecyclePolicyDocumentRuleSelection.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ecr.kotlin.inputs
import com.pulumi.aws.ecr.inputs.GetLifecyclePolicyDocumentRuleSelection.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property countNumber Specify a count number. If the `count_type` used is "imageCountMoreThan", then the value is the maximum number of images that you want to retain in your repository. If the `count_type` used is "sinceImagePushed", then the value is the maximum age limit for your images.
* @property countType Specify a count type to apply to the images. If `count_type` is set to "imageCountMoreThan", you also specify `count_number` to create a rule that sets a limit on the number of images that exist in your repository. If `count_type` is set to "sinceImagePushed", you also specify `count_unit` and `count_number` to specify a time limit on the images that exist in your repository.
* @property countUnit Specify a count unit of days to indicate that as the unit of time, in addition to `count_number`, which is the number of days.
* @property tagPatternLists You must specify a comma-separated list of image tag patterns that may contain wildcards (\*) on which to take action with your lifecycle policy. For example, if your images are tagged as `prod`, `prod1`, `prod2`, and so on, you would use the tag pattern list `["prod\*"]` to specify all of them. If you specify multiple tags, only the images with all specified tags are selected. There is a maximum limit of four wildcards (\*) per string. For example, `["*test*1*2*3", "test*1*2*3*"]` is valid but `["test*1*2*3*4*5*6"]` is invalid.
* @property tagPrefixLists You must specify a comma-separated list of image tag prefixes on which to take action with your lifecycle policy. For example, if your images are tagged as `prod`, `prod1`, `prod2`, and so on, you would use the tag prefix "prod" to specify all of them. If you specify multiple tags, only images with all specified tags are selected.
* @property tagStatus Determines whether the lifecycle policy rule that you are adding specifies a tag for an image. Acceptable options are "tagged", "untagged", or "any". If you specify "any", then all images have the rule applied to them. If you specify "tagged", then you must also specify a `tag_prefix_list` value. If you specify "untagged", then you must omit `tag_prefix_list`.
*/
public data class GetLifecyclePolicyDocumentRuleSelection(
public val countNumber: Int,
public val countType: String,
public val countUnit: String? = null,
public val tagPatternLists: List? = null,
public val tagPrefixLists: List? = null,
public val tagStatus: String,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.ecr.inputs.GetLifecyclePolicyDocumentRuleSelection =
com.pulumi.aws.ecr.inputs.GetLifecyclePolicyDocumentRuleSelection.builder()
.countNumber(countNumber.let({ args0 -> args0 }))
.countType(countType.let({ args0 -> args0 }))
.countUnit(countUnit?.let({ args0 -> args0 }))
.tagPatternLists(tagPatternLists?.let({ args0 -> args0.map({ args0 -> args0 }) }))
.tagPrefixLists(tagPrefixLists?.let({ args0 -> args0.map({ args0 -> args0 }) }))
.tagStatus(tagStatus.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetLifecyclePolicyDocumentRuleSelection].
*/
@PulumiTagMarker
public class GetLifecyclePolicyDocumentRuleSelectionBuilder internal constructor() {
private var countNumber: Int? = null
private var countType: String? = null
private var countUnit: String? = null
private var tagPatternLists: List? = null
private var tagPrefixLists: List? = null
private var tagStatus: String? = null
/**
* @param value Specify a count number. If the `count_type` used is "imageCountMoreThan", then the value is the maximum number of images that you want to retain in your repository. If the `count_type` used is "sinceImagePushed", then the value is the maximum age limit for your images.
*/
@JvmName("awhjusdfucmkgmsp")
public suspend fun countNumber(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.countNumber = mapped
}
/**
* @param value Specify a count type to apply to the images. If `count_type` is set to "imageCountMoreThan", you also specify `count_number` to create a rule that sets a limit on the number of images that exist in your repository. If `count_type` is set to "sinceImagePushed", you also specify `count_unit` and `count_number` to specify a time limit on the images that exist in your repository.
*/
@JvmName("hnydmjpptpyajckc")
public suspend fun countType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.countType = mapped
}
/**
* @param value Specify a count unit of days to indicate that as the unit of time, in addition to `count_number`, which is the number of days.
*/
@JvmName("harutplpnbygxnds")
public suspend fun countUnit(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.countUnit = mapped
}
/**
* @param value You must specify a comma-separated list of image tag patterns that may contain wildcards (\*) on which to take action with your lifecycle policy. For example, if your images are tagged as `prod`, `prod1`, `prod2`, and so on, you would use the tag pattern list `["prod\*"]` to specify all of them. If you specify multiple tags, only the images with all specified tags are selected. There is a maximum limit of four wildcards (\*) per string. For example, `["*test*1*2*3", "test*1*2*3*"]` is valid but `["test*1*2*3*4*5*6"]` is invalid.
*/
@JvmName("gbpbipptirxaifki")
public suspend fun tagPatternLists(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.tagPatternLists = mapped
}
/**
* @param values You must specify a comma-separated list of image tag patterns that may contain wildcards (\*) on which to take action with your lifecycle policy. For example, if your images are tagged as `prod`, `prod1`, `prod2`, and so on, you would use the tag pattern list `["prod\*"]` to specify all of them. If you specify multiple tags, only the images with all specified tags are selected. There is a maximum limit of four wildcards (\*) per string. For example, `["*test*1*2*3", "test*1*2*3*"]` is valid but `["test*1*2*3*4*5*6"]` is invalid.
*/
@JvmName("klihbekaltvwtbwg")
public suspend fun tagPatternLists(vararg values: String) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.tagPatternLists = mapped
}
/**
* @param value You must specify a comma-separated list of image tag prefixes on which to take action with your lifecycle policy. For example, if your images are tagged as `prod`, `prod1`, `prod2`, and so on, you would use the tag prefix "prod" to specify all of them. If you specify multiple tags, only images with all specified tags are selected.
*/
@JvmName("hamjylftyqiypyiu")
public suspend fun tagPrefixLists(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.tagPrefixLists = mapped
}
/**
* @param values You must specify a comma-separated list of image tag prefixes on which to take action with your lifecycle policy. For example, if your images are tagged as `prod`, `prod1`, `prod2`, and so on, you would use the tag prefix "prod" to specify all of them. If you specify multiple tags, only images with all specified tags are selected.
*/
@JvmName("cweppdnrsaheqrvx")
public suspend fun tagPrefixLists(vararg values: String) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.tagPrefixLists = mapped
}
/**
* @param value Determines whether the lifecycle policy rule that you are adding specifies a tag for an image. Acceptable options are "tagged", "untagged", or "any". If you specify "any", then all images have the rule applied to them. If you specify "tagged", then you must also specify a `tag_prefix_list` value. If you specify "untagged", then you must omit `tag_prefix_list`.
*/
@JvmName("tudaklmfnebdvbud")
public suspend fun tagStatus(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.tagStatus = mapped
}
internal fun build(): GetLifecyclePolicyDocumentRuleSelection =
GetLifecyclePolicyDocumentRuleSelection(
countNumber = countNumber ?: throw PulumiNullFieldException("countNumber"),
countType = countType ?: throw PulumiNullFieldException("countType"),
countUnit = countUnit,
tagPatternLists = tagPatternLists,
tagPrefixLists = tagPrefixLists,
tagStatus = tagStatus ?: throw PulumiNullFieldException("tagStatus"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy