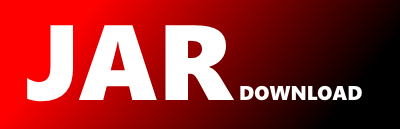
com.pulumi.aws.ecs.kotlin.inputs.GetTaskExecutionOverridesContainerOverride.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ecs.kotlin.inputs
import com.pulumi.aws.ecs.inputs.GetTaskExecutionOverridesContainerOverride.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property commands The command to send to the container that overrides the default command from the Docker image or the task definition.
* @property cpu The number of cpu units reserved for the container, instead of the default value from the task definition.
* @property environments The environment variables to send to the container. You can add new environment variables, which are added to the container at launch, or you can override the existing environment variables from the Docker image or the task definition. See below.
* @property memory The hard limit (in MiB) of memory to present to the container, instead of the default value from the task definition. If your container attempts to exceed the memory specified here, the container is killed.
* @property memoryReservation The soft limit (in MiB) of memory to reserve for the container, instead of the default value from the task definition.
* @property name The name of the container that receives the override. This parameter is required if any override is specified.
* @property resourceRequirements The type and amount of a resource to assign to a container, instead of the default value from the task definition. The only supported resource is a GPU. See below.
*/
public data class GetTaskExecutionOverridesContainerOverride(
public val commands: List? = null,
public val cpu: Int? = null,
public val environments: List? = null,
public val memory: Int? = null,
public val memoryReservation: Int? = null,
public val name: String,
public val resourceRequirements: List? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.ecs.inputs.GetTaskExecutionOverridesContainerOverride =
com.pulumi.aws.ecs.inputs.GetTaskExecutionOverridesContainerOverride.builder()
.commands(commands?.let({ args0 -> args0.map({ args0 -> args0 }) }))
.cpu(cpu?.let({ args0 -> args0 }))
.environments(
environments?.let({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.memory(memory?.let({ args0 -> args0 }))
.memoryReservation(memoryReservation?.let({ args0 -> args0 }))
.name(name.let({ args0 -> args0 }))
.resourceRequirements(
resourceRequirements?.let({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [GetTaskExecutionOverridesContainerOverride].
*/
@PulumiTagMarker
public class GetTaskExecutionOverridesContainerOverrideBuilder internal constructor() {
private var commands: List? = null
private var cpu: Int? = null
private var environments: List? = null
private var memory: Int? = null
private var memoryReservation: Int? = null
private var name: String? = null
private var resourceRequirements:
List? = null
/**
* @param value The command to send to the container that overrides the default command from the Docker image or the task definition.
*/
@JvmName("reujvjpvmloepwte")
public suspend fun commands(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.commands = mapped
}
/**
* @param values The command to send to the container that overrides the default command from the Docker image or the task definition.
*/
@JvmName("bfrkujaskbvlfnfd")
public suspend fun commands(vararg values: String) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.commands = mapped
}
/**
* @param value The number of cpu units reserved for the container, instead of the default value from the task definition.
*/
@JvmName("janfanmtlvydbhhd")
public suspend fun cpu(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.cpu = mapped
}
/**
* @param value The environment variables to send to the container. You can add new environment variables, which are added to the container at launch, or you can override the existing environment variables from the Docker image or the task definition. See below.
*/
@JvmName("mlvddogpitcebojf")
public suspend fun environments(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.environments = mapped
}
/**
* @param argument The environment variables to send to the container. You can add new environment variables, which are added to the container at launch, or you can override the existing environment variables from the Docker image or the task definition. See below.
*/
@JvmName("abuwhandjvhbthjl")
public suspend fun environments(argument: List Unit>) {
val toBeMapped = argument.toList().map {
GetTaskExecutionOverridesContainerOverrideEnvironmentBuilder().applySuspend { it() }.build()
}
val mapped = toBeMapped
this.environments = mapped
}
/**
* @param argument The environment variables to send to the container. You can add new environment variables, which are added to the container at launch, or you can override the existing environment variables from the Docker image or the task definition. See below.
*/
@JvmName("filaexocubqqeqbt")
public suspend fun environments(vararg argument: suspend GetTaskExecutionOverridesContainerOverrideEnvironmentBuilder.() -> Unit) {
val toBeMapped = argument.toList().map {
GetTaskExecutionOverridesContainerOverrideEnvironmentBuilder().applySuspend { it() }.build()
}
val mapped = toBeMapped
this.environments = mapped
}
/**
* @param argument The environment variables to send to the container. You can add new environment variables, which are added to the container at launch, or you can override the existing environment variables from the Docker image or the task definition. See below.
*/
@JvmName("lcycqhrqoanumtoj")
public suspend fun environments(argument: suspend GetTaskExecutionOverridesContainerOverrideEnvironmentBuilder.() -> Unit) {
val toBeMapped =
listOf(
GetTaskExecutionOverridesContainerOverrideEnvironmentBuilder().applySuspend {
argument()
}.build(),
)
val mapped = toBeMapped
this.environments = mapped
}
/**
* @param values The environment variables to send to the container. You can add new environment variables, which are added to the container at launch, or you can override the existing environment variables from the Docker image or the task definition. See below.
*/
@JvmName("itnkqmsyauvtbitt")
public suspend fun environments(vararg values: GetTaskExecutionOverridesContainerOverrideEnvironment) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.environments = mapped
}
/**
* @param value The hard limit (in MiB) of memory to present to the container, instead of the default value from the task definition. If your container attempts to exceed the memory specified here, the container is killed.
*/
@JvmName("pjqmoqjqtbewdmgq")
public suspend fun memory(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.memory = mapped
}
/**
* @param value The soft limit (in MiB) of memory to reserve for the container, instead of the default value from the task definition.
*/
@JvmName("cdaqqvaalwlptngm")
public suspend fun memoryReservation(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.memoryReservation = mapped
}
/**
* @param value The name of the container that receives the override. This parameter is required if any override is specified.
*/
@JvmName("npefcpnpqiiimovc")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.name = mapped
}
/**
* @param value The type and amount of a resource to assign to a container, instead of the default value from the task definition. The only supported resource is a GPU. See below.
*/
@JvmName("jhfwdkhlfkxaogod")
public suspend fun resourceRequirements(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.resourceRequirements = mapped
}
/**
* @param argument The type and amount of a resource to assign to a container, instead of the default value from the task definition. The only supported resource is a GPU. See below.
*/
@JvmName("cmqcorrxhsymbchs")
public suspend fun resourceRequirements(argument: List Unit>) {
val toBeMapped = argument.toList().map {
GetTaskExecutionOverridesContainerOverrideResourceRequirementBuilder().applySuspend {
it()
}.build()
}
val mapped = toBeMapped
this.resourceRequirements = mapped
}
/**
* @param argument The type and amount of a resource to assign to a container, instead of the default value from the task definition. The only supported resource is a GPU. See below.
*/
@JvmName("vpghsiqtfpginkfa")
public suspend fun resourceRequirements(vararg argument: suspend GetTaskExecutionOverridesContainerOverrideResourceRequirementBuilder.() -> Unit) {
val toBeMapped = argument.toList().map {
GetTaskExecutionOverridesContainerOverrideResourceRequirementBuilder().applySuspend {
it()
}.build()
}
val mapped = toBeMapped
this.resourceRequirements = mapped
}
/**
* @param argument The type and amount of a resource to assign to a container, instead of the default value from the task definition. The only supported resource is a GPU. See below.
*/
@JvmName("evvmacpxtsqqsqcg")
public suspend fun resourceRequirements(argument: suspend GetTaskExecutionOverridesContainerOverrideResourceRequirementBuilder.() -> Unit) {
val toBeMapped =
listOf(
GetTaskExecutionOverridesContainerOverrideResourceRequirementBuilder().applySuspend {
argument()
}.build(),
)
val mapped = toBeMapped
this.resourceRequirements = mapped
}
/**
* @param values The type and amount of a resource to assign to a container, instead of the default value from the task definition. The only supported resource is a GPU. See below.
*/
@JvmName("dxlpjowjxssnhlul")
public suspend fun resourceRequirements(vararg values: GetTaskExecutionOverridesContainerOverrideResourceRequirement) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.resourceRequirements = mapped
}
internal fun build(): GetTaskExecutionOverridesContainerOverride =
GetTaskExecutionOverridesContainerOverride(
commands = commands,
cpu = cpu,
environments = environments,
memory = memory,
memoryReservation = memoryReservation,
name = name ?: throw PulumiNullFieldException("name"),
resourceRequirements = resourceRequirements,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy