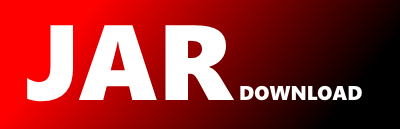
com.pulumi.aws.ecs.kotlin.inputs.ServiceVolumeConfigurationManagedEbsVolumeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ecs.kotlin.inputs
import com.pulumi.aws.ecs.inputs.ServiceVolumeConfigurationManagedEbsVolumeArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property encrypted Whether the volume should be encrypted. Default value is `true`.
* @property fileSystemType Linux filesystem type for the volume. For volumes created from a snapshot, same filesystem type must be specified that the volume was using when the snapshot was created. Valid values are `ext3`, `ext4`, `xfs`. Default value is `xfs`.
* @property iops Number of I/O operations per second (IOPS).
* @property kmsKeyId Amazon Resource Name (ARN) identifier of the Amazon Web Services Key Management Service key to use for Amazon EBS encryption.
* @property roleArn Amazon ECS infrastructure IAM role that is used to manage your Amazon Web Services infrastructure. Recommended using the Amazon ECS-managed `AmazonECSInfrastructureRolePolicyForVolumes` IAM policy with this role.
* @property sizeInGb Size of the volume in GiB. You must specify either a `size_in_gb` or a `snapshot_id`. You can optionally specify a volume size greater than or equal to the snapshot size.
* @property snapshotId Snapshot that Amazon ECS uses to create the volume. You must specify either a `size_in_gb` or a `snapshot_id`.
* @property tagSpecifications The tags to apply to the volume. See below.
* @property throughput Throughput to provision for a volume, in MiB/s, with a maximum of 1,000 MiB/s.
* @property volumeType Volume type.
*/
public data class ServiceVolumeConfigurationManagedEbsVolumeArgs(
public val encrypted: Output? = null,
public val fileSystemType: Output? = null,
public val iops: Output? = null,
public val kmsKeyId: Output? = null,
public val roleArn: Output,
public val sizeInGb: Output? = null,
public val snapshotId: Output? = null,
public val tagSpecifications: Output>? = null,
public val throughput: Output? = null,
public val volumeType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.ecs.inputs.ServiceVolumeConfigurationManagedEbsVolumeArgs =
com.pulumi.aws.ecs.inputs.ServiceVolumeConfigurationManagedEbsVolumeArgs.builder()
.encrypted(encrypted?.applyValue({ args0 -> args0 }))
.fileSystemType(fileSystemType?.applyValue({ args0 -> args0 }))
.iops(iops?.applyValue({ args0 -> args0 }))
.kmsKeyId(kmsKeyId?.applyValue({ args0 -> args0 }))
.roleArn(roleArn.applyValue({ args0 -> args0 }))
.sizeInGb(sizeInGb?.applyValue({ args0 -> args0 }))
.snapshotId(snapshotId?.applyValue({ args0 -> args0 }))
.tagSpecifications(
tagSpecifications?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.throughput(throughput?.applyValue({ args0 -> args0 }))
.volumeType(volumeType?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ServiceVolumeConfigurationManagedEbsVolumeArgs].
*/
@PulumiTagMarker
public class ServiceVolumeConfigurationManagedEbsVolumeArgsBuilder internal constructor() {
private var encrypted: Output? = null
private var fileSystemType: Output? = null
private var iops: Output? = null
private var kmsKeyId: Output? = null
private var roleArn: Output? = null
private var sizeInGb: Output? = null
private var snapshotId: Output? = null
private var tagSpecifications:
Output>? = null
private var throughput: Output? = null
private var volumeType: Output? = null
/**
* @param value Whether the volume should be encrypted. Default value is `true`.
*/
@JvmName("lqkidmmnwyvarypo")
public suspend fun encrypted(`value`: Output) {
this.encrypted = value
}
/**
* @param value Linux filesystem type for the volume. For volumes created from a snapshot, same filesystem type must be specified that the volume was using when the snapshot was created. Valid values are `ext3`, `ext4`, `xfs`. Default value is `xfs`.
*/
@JvmName("ttebexjqoiaxjvfh")
public suspend fun fileSystemType(`value`: Output) {
this.fileSystemType = value
}
/**
* @param value Number of I/O operations per second (IOPS).
*/
@JvmName("mbsnwfvhlkwhsvvb")
public suspend fun iops(`value`: Output) {
this.iops = value
}
/**
* @param value Amazon Resource Name (ARN) identifier of the Amazon Web Services Key Management Service key to use for Amazon EBS encryption.
*/
@JvmName("ccjbwnwdegfogehw")
public suspend fun kmsKeyId(`value`: Output) {
this.kmsKeyId = value
}
/**
* @param value Amazon ECS infrastructure IAM role that is used to manage your Amazon Web Services infrastructure. Recommended using the Amazon ECS-managed `AmazonECSInfrastructureRolePolicyForVolumes` IAM policy with this role.
*/
@JvmName("fixhqvixtsnspkys")
public suspend fun roleArn(`value`: Output) {
this.roleArn = value
}
/**
* @param value Size of the volume in GiB. You must specify either a `size_in_gb` or a `snapshot_id`. You can optionally specify a volume size greater than or equal to the snapshot size.
*/
@JvmName("nnprlfxkkrcjsrlm")
public suspend fun sizeInGb(`value`: Output) {
this.sizeInGb = value
}
/**
* @param value Snapshot that Amazon ECS uses to create the volume. You must specify either a `size_in_gb` or a `snapshot_id`.
*/
@JvmName("lweoqyaikgwilfvm")
public suspend fun snapshotId(`value`: Output) {
this.snapshotId = value
}
/**
* @param value The tags to apply to the volume. See below.
*/
@JvmName("fvbxclulvywqvnpa")
public suspend fun tagSpecifications(`value`: Output>) {
this.tagSpecifications = value
}
@JvmName("dmxkguuufyopsggt")
public suspend fun tagSpecifications(vararg values: Output) {
this.tagSpecifications = Output.all(values.asList())
}
/**
* @param values The tags to apply to the volume. See below.
*/
@JvmName("geqgyhfuwmvvxfcs")
public suspend fun tagSpecifications(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy