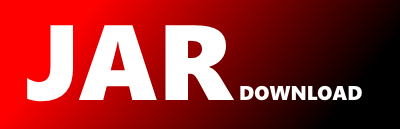
com.pulumi.aws.ecs.kotlin.inputs.TaskDefinitionVolumeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ecs.kotlin.inputs
import com.pulumi.aws.ecs.inputs.TaskDefinitionVolumeArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property configureAtLaunch Whether the volume should be configured at launch time. This is used to create Amazon EBS volumes for standalone tasks or tasks created as part of a service. Each task definition revision may only have one volume configured at launch in the volume configuration.
* @property dockerVolumeConfiguration Configuration block to configure a docker volume. Detailed below.
* @property efsVolumeConfiguration Configuration block for an EFS volume. Detailed below.
* @property fsxWindowsFileServerVolumeConfiguration Configuration block for an FSX Windows File Server volume. Detailed below.
* @property hostPath Path on the host container instance that is presented to the container. If not set, ECS will create a nonpersistent data volume that starts empty and is deleted after the task has finished.
* @property name Name of the volume. This name is referenced in the `sourceVolume`
* parameter of container definition in the `mountPoints` section.
*/
public data class TaskDefinitionVolumeArgs(
public val configureAtLaunch: Output? = null,
public val dockerVolumeConfiguration: Output? =
null,
public val efsVolumeConfiguration: Output? = null,
public val fsxWindowsFileServerVolumeConfiguration: Output? = null,
public val hostPath: Output? = null,
public val name: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.ecs.inputs.TaskDefinitionVolumeArgs =
com.pulumi.aws.ecs.inputs.TaskDefinitionVolumeArgs.builder()
.configureAtLaunch(configureAtLaunch?.applyValue({ args0 -> args0 }))
.dockerVolumeConfiguration(
dockerVolumeConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.efsVolumeConfiguration(
efsVolumeConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.fsxWindowsFileServerVolumeConfiguration(
fsxWindowsFileServerVolumeConfiguration?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.hostPath(hostPath?.applyValue({ args0 -> args0 }))
.name(name.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TaskDefinitionVolumeArgs].
*/
@PulumiTagMarker
public class TaskDefinitionVolumeArgsBuilder internal constructor() {
private var configureAtLaunch: Output? = null
private var dockerVolumeConfiguration: Output? =
null
private var efsVolumeConfiguration: Output? = null
private var fsxWindowsFileServerVolumeConfiguration:
Output? = null
private var hostPath: Output? = null
private var name: Output? = null
/**
* @param value Whether the volume should be configured at launch time. This is used to create Amazon EBS volumes for standalone tasks or tasks created as part of a service. Each task definition revision may only have one volume configured at launch in the volume configuration.
*/
@JvmName("mgiiplccuaggyijn")
public suspend fun configureAtLaunch(`value`: Output) {
this.configureAtLaunch = value
}
/**
* @param value Configuration block to configure a docker volume. Detailed below.
*/
@JvmName("ynenweurkfgvoron")
public suspend fun dockerVolumeConfiguration(`value`: Output) {
this.dockerVolumeConfiguration = value
}
/**
* @param value Configuration block for an EFS volume. Detailed below.
*/
@JvmName("enyqrenkdlskcsoj")
public suspend fun efsVolumeConfiguration(`value`: Output) {
this.efsVolumeConfiguration = value
}
/**
* @param value Configuration block for an FSX Windows File Server volume. Detailed below.
*/
@JvmName("eglbapbvsxrchuke")
public suspend fun fsxWindowsFileServerVolumeConfiguration(`value`: Output) {
this.fsxWindowsFileServerVolumeConfiguration = value
}
/**
* @param value Path on the host container instance that is presented to the container. If not set, ECS will create a nonpersistent data volume that starts empty and is deleted after the task has finished.
*/
@JvmName("jqwnbxeatqyspcgf")
public suspend fun hostPath(`value`: Output) {
this.hostPath = value
}
/**
* @param value Name of the volume. This name is referenced in the `sourceVolume`
* parameter of container definition in the `mountPoints` section.
*/
@JvmName("juykfxunpoxyrmpm")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Whether the volume should be configured at launch time. This is used to create Amazon EBS volumes for standalone tasks or tasks created as part of a service. Each task definition revision may only have one volume configured at launch in the volume configuration.
*/
@JvmName("xcvxbexhmxwoneoh")
public suspend fun configureAtLaunch(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.configureAtLaunch = mapped
}
/**
* @param value Configuration block to configure a docker volume. Detailed below.
*/
@JvmName("vebloncqjvgrksej")
public suspend fun dockerVolumeConfiguration(`value`: TaskDefinitionVolumeDockerVolumeConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dockerVolumeConfiguration = mapped
}
/**
* @param argument Configuration block to configure a docker volume. Detailed below.
*/
@JvmName("cpdpggejkwtmgjux")
public suspend fun dockerVolumeConfiguration(argument: suspend TaskDefinitionVolumeDockerVolumeConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = TaskDefinitionVolumeDockerVolumeConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.dockerVolumeConfiguration = mapped
}
/**
* @param value Configuration block for an EFS volume. Detailed below.
*/
@JvmName("vulfdgebwjjwpcxg")
public suspend fun efsVolumeConfiguration(`value`: TaskDefinitionVolumeEfsVolumeConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.efsVolumeConfiguration = mapped
}
/**
* @param argument Configuration block for an EFS volume. Detailed below.
*/
@JvmName("dwitkqdwpghihoow")
public suspend fun efsVolumeConfiguration(argument: suspend TaskDefinitionVolumeEfsVolumeConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = TaskDefinitionVolumeEfsVolumeConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.efsVolumeConfiguration = mapped
}
/**
* @param value Configuration block for an FSX Windows File Server volume. Detailed below.
*/
@JvmName("xuxvohgxwdhytkrl")
public suspend fun fsxWindowsFileServerVolumeConfiguration(`value`: TaskDefinitionVolumeFsxWindowsFileServerVolumeConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fsxWindowsFileServerVolumeConfiguration = mapped
}
/**
* @param argument Configuration block for an FSX Windows File Server volume. Detailed below.
*/
@JvmName("huwioorehalodatt")
public suspend fun fsxWindowsFileServerVolumeConfiguration(argument: suspend TaskDefinitionVolumeFsxWindowsFileServerVolumeConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
TaskDefinitionVolumeFsxWindowsFileServerVolumeConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.fsxWindowsFileServerVolumeConfiguration = mapped
}
/**
* @param value Path on the host container instance that is presented to the container. If not set, ECS will create a nonpersistent data volume that starts empty and is deleted after the task has finished.
*/
@JvmName("fstiddvbvljdjkmj")
public suspend fun hostPath(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hostPath = mapped
}
/**
* @param value Name of the volume. This name is referenced in the `sourceVolume`
* parameter of container definition in the `mountPoints` section.
*/
@JvmName("snceavyskesrteex")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
internal fun build(): TaskDefinitionVolumeArgs = TaskDefinitionVolumeArgs(
configureAtLaunch = configureAtLaunch,
dockerVolumeConfiguration = dockerVolumeConfiguration,
efsVolumeConfiguration = efsVolumeConfiguration,
fsxWindowsFileServerVolumeConfiguration = fsxWindowsFileServerVolumeConfiguration,
hostPath = hostPath,
name = name ?: throw PulumiNullFieldException("name"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy