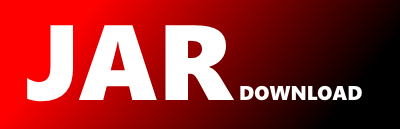
com.pulumi.aws.efs.kotlin.MountTargetArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.efs.kotlin
import com.pulumi.aws.efs.MountTargetArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Provides an Elastic File System (EFS) mount target.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const foo = new aws.ec2.Vpc("foo", {cidrBlock: "10.0.0.0/16"});
* const alphaSubnet = new aws.ec2.Subnet("alpha", {
* vpcId: foo.id,
* availabilityZone: "us-west-2a",
* cidrBlock: "10.0.1.0/24",
* });
* const alpha = new aws.efs.MountTarget("alpha", {
* fileSystemId: fooAwsEfsFileSystem.id,
* subnetId: alphaSubnet.id,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* foo = aws.ec2.Vpc("foo", cidr_block="10.0.0.0/16")
* alpha_subnet = aws.ec2.Subnet("alpha",
* vpc_id=foo.id,
* availability_zone="us-west-2a",
* cidr_block="10.0.1.0/24")
* alpha = aws.efs.MountTarget("alpha",
* file_system_id=foo_aws_efs_file_system["id"],
* subnet_id=alpha_subnet.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var foo = new Aws.Ec2.Vpc("foo", new()
* {
* CidrBlock = "10.0.0.0/16",
* });
* var alphaSubnet = new Aws.Ec2.Subnet("alpha", new()
* {
* VpcId = foo.Id,
* AvailabilityZone = "us-west-2a",
* CidrBlock = "10.0.1.0/24",
* });
* var alpha = new Aws.Efs.MountTarget("alpha", new()
* {
* FileSystemId = fooAwsEfsFileSystem.Id,
* SubnetId = alphaSubnet.Id,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ec2"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/efs"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* foo, err := ec2.NewVpc(ctx, "foo", &ec2.VpcArgs{
* CidrBlock: pulumi.String("10.0.0.0/16"),
* })
* if err != nil {
* return err
* }
* alphaSubnet, err := ec2.NewSubnet(ctx, "alpha", &ec2.SubnetArgs{
* VpcId: foo.ID(),
* AvailabilityZone: pulumi.String("us-west-2a"),
* CidrBlock: pulumi.String("10.0.1.0/24"),
* })
* if err != nil {
* return err
* }
* _, err = efs.NewMountTarget(ctx, "alpha", &efs.MountTargetArgs{
* FileSystemId: pulumi.Any(fooAwsEfsFileSystem.Id),
* SubnetId: alphaSubnet.ID(),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.Vpc;
* import com.pulumi.aws.ec2.VpcArgs;
* import com.pulumi.aws.ec2.Subnet;
* import com.pulumi.aws.ec2.SubnetArgs;
* import com.pulumi.aws.efs.MountTarget;
* import com.pulumi.aws.efs.MountTargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var foo = new Vpc("foo", VpcArgs.builder()
* .cidrBlock("10.0.0.0/16")
* .build());
* var alphaSubnet = new Subnet("alphaSubnet", SubnetArgs.builder()
* .vpcId(foo.id())
* .availabilityZone("us-west-2a")
* .cidrBlock("10.0.1.0/24")
* .build());
* var alpha = new MountTarget("alpha", MountTargetArgs.builder()
* .fileSystemId(fooAwsEfsFileSystem.id())
* .subnetId(alphaSubnet.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* alpha:
* type: aws:efs:MountTarget
* properties:
* fileSystemId: ${fooAwsEfsFileSystem.id}
* subnetId: ${alphaSubnet.id}
* foo:
* type: aws:ec2:Vpc
* properties:
* cidrBlock: 10.0.0.0/16
* alphaSubnet:
* type: aws:ec2:Subnet
* name: alpha
* properties:
* vpcId: ${foo.id}
* availabilityZone: us-west-2a
* cidrBlock: 10.0.1.0/24
* ```
*
* ## Import
* Using `pulumi import`, import the EFS mount targets using the `id`. For example:
* ```sh
* $ pulumi import aws:efs/mountTarget:MountTarget alpha fsmt-52a643fb
* ```
* @property fileSystemId The ID of the file system for which the mount target is intended.
* @property ipAddress The address (within the address range of the specified subnet) at
* which the file system may be mounted via the mount target.
* @property securityGroups A list of up to 5 VPC security group IDs (that must
* be for the same VPC as subnet specified) in effect for the mount target.
* @property subnetId The ID of the subnet to add the mount target in.
*/
public data class MountTargetArgs(
public val fileSystemId: Output? = null,
public val ipAddress: Output? = null,
public val securityGroups: Output>? = null,
public val subnetId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.efs.MountTargetArgs =
com.pulumi.aws.efs.MountTargetArgs.builder()
.fileSystemId(fileSystemId?.applyValue({ args0 -> args0 }))
.ipAddress(ipAddress?.applyValue({ args0 -> args0 }))
.securityGroups(securityGroups?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.subnetId(subnetId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [MountTargetArgs].
*/
@PulumiTagMarker
public class MountTargetArgsBuilder internal constructor() {
private var fileSystemId: Output? = null
private var ipAddress: Output? = null
private var securityGroups: Output>? = null
private var subnetId: Output? = null
/**
* @param value The ID of the file system for which the mount target is intended.
*/
@JvmName("gfdkmpyrsoljfdgu")
public suspend fun fileSystemId(`value`: Output) {
this.fileSystemId = value
}
/**
* @param value The address (within the address range of the specified subnet) at
* which the file system may be mounted via the mount target.
*/
@JvmName("laeghbvcjbfounhb")
public suspend fun ipAddress(`value`: Output) {
this.ipAddress = value
}
/**
* @param value A list of up to 5 VPC security group IDs (that must
* be for the same VPC as subnet specified) in effect for the mount target.
*/
@JvmName("mdegkoqeovgqarfp")
public suspend fun securityGroups(`value`: Output>) {
this.securityGroups = value
}
@JvmName("pgvrhkqvypcpnwkp")
public suspend fun securityGroups(vararg values: Output) {
this.securityGroups = Output.all(values.asList())
}
/**
* @param values A list of up to 5 VPC security group IDs (that must
* be for the same VPC as subnet specified) in effect for the mount target.
*/
@JvmName("denegaqegnlotfcb")
public suspend fun securityGroups(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy