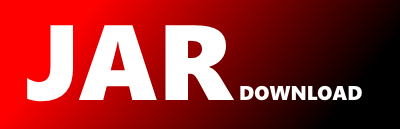
com.pulumi.aws.eks.kotlin.inputs.ClusterComputeConfigArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.eks.kotlin.inputs
import com.pulumi.aws.eks.inputs.ClusterComputeConfigArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property enabled Request to enable or disable the compute capability on your EKS Auto Mode cluster. If the compute capability is enabled, EKS Auto Mode will create and delete EC2 Managed Instances in your Amazon Web Services account.
* @property nodePools Configuration for node pools that defines the compute resources for your EKS Auto Mode cluster. Valid options are `general-purpose` and `system`.
* @property nodeRoleArn The ARN of the IAM Role EKS will assign to EC2 Managed Instances in your EKS Auto Mode cluster. This value cannot be changed after the compute capability of EKS Auto Mode is enabled..
*/
public data class ClusterComputeConfigArgs(
public val enabled: Output? = null,
public val nodePools: Output>? = null,
public val nodeRoleArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.eks.inputs.ClusterComputeConfigArgs =
com.pulumi.aws.eks.inputs.ClusterComputeConfigArgs.builder()
.enabled(enabled?.applyValue({ args0 -> args0 }))
.nodePools(nodePools?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.nodeRoleArn(nodeRoleArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ClusterComputeConfigArgs].
*/
@PulumiTagMarker
public class ClusterComputeConfigArgsBuilder internal constructor() {
private var enabled: Output? = null
private var nodePools: Output>? = null
private var nodeRoleArn: Output? = null
/**
* @param value Request to enable or disable the compute capability on your EKS Auto Mode cluster. If the compute capability is enabled, EKS Auto Mode will create and delete EC2 Managed Instances in your Amazon Web Services account.
*/
@JvmName("nvqpgxvqlornrubh")
public suspend fun enabled(`value`: Output) {
this.enabled = value
}
/**
* @param value Configuration for node pools that defines the compute resources for your EKS Auto Mode cluster. Valid options are `general-purpose` and `system`.
*/
@JvmName("qyfrpkspklprcjqw")
public suspend fun nodePools(`value`: Output>) {
this.nodePools = value
}
@JvmName("gbavokssurjhumsl")
public suspend fun nodePools(vararg values: Output) {
this.nodePools = Output.all(values.asList())
}
/**
* @param values Configuration for node pools that defines the compute resources for your EKS Auto Mode cluster. Valid options are `general-purpose` and `system`.
*/
@JvmName("mjykvdvicurkcefy")
public suspend fun nodePools(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy