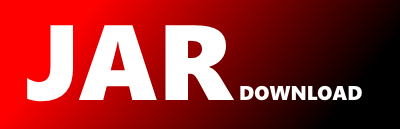
com.pulumi.aws.eks.kotlin.inputs.ClusterKubernetesNetworkConfigArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.eks.kotlin.inputs
import com.pulumi.aws.eks.inputs.ClusterKubernetesNetworkConfigArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property elasticLoadBalancing Configuration block with elastic load balancing configuration for the cluster. Detailed below.
* @property ipFamily The IP family used to assign Kubernetes pod and service addresses. Valid values are `ipv4` (default) and `ipv6`. You can only specify an IP family when you create a cluster, changing this value will force a new cluster to be created.
* @property serviceIpv4Cidr The CIDR block to assign Kubernetes pod and service IP addresses from. If you don't specify a block, Kubernetes assigns addresses from either the 10.100.0.0/16 or 172.20.0.0/16 CIDR blocks. We recommend that you specify a block that does not overlap with resources in other networks that are peered or connected to your VPC. You can only specify a custom CIDR block when you create a cluster, changing this value will force a new cluster to be created. The block must meet the following requirements:
* * Within one of the following private IP address blocks: 10.0.0.0/8, 172.16.0.0/12, or 192.168.0.0/16.
* * Doesn't overlap with any CIDR block assigned to the VPC that you selected for VPC.
* * Between /24 and /12.
* @property serviceIpv6Cidr The CIDR block that Kubernetes pod and service IP addresses are assigned from if you specified `ipv6` for `ip_family` when you created the cluster. Kubernetes assigns service addresses from the unique local address range (fc00::/7) because you can't specify a custom IPv6 CIDR block when you create the cluster.
*/
public data class ClusterKubernetesNetworkConfigArgs(
public val elasticLoadBalancing: Output? =
null,
public val ipFamily: Output? = null,
public val serviceIpv4Cidr: Output? = null,
public val serviceIpv6Cidr: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.eks.inputs.ClusterKubernetesNetworkConfigArgs =
com.pulumi.aws.eks.inputs.ClusterKubernetesNetworkConfigArgs.builder()
.elasticLoadBalancing(
elasticLoadBalancing?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.ipFamily(ipFamily?.applyValue({ args0 -> args0 }))
.serviceIpv4Cidr(serviceIpv4Cidr?.applyValue({ args0 -> args0 }))
.serviceIpv6Cidr(serviceIpv6Cidr?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ClusterKubernetesNetworkConfigArgs].
*/
@PulumiTagMarker
public class ClusterKubernetesNetworkConfigArgsBuilder internal constructor() {
private var elasticLoadBalancing: Output? =
null
private var ipFamily: Output? = null
private var serviceIpv4Cidr: Output? = null
private var serviceIpv6Cidr: Output? = null
/**
* @param value Configuration block with elastic load balancing configuration for the cluster. Detailed below.
*/
@JvmName("cxclygcrcsnkqapj")
public suspend fun elasticLoadBalancing(`value`: Output) {
this.elasticLoadBalancing = value
}
/**
* @param value The IP family used to assign Kubernetes pod and service addresses. Valid values are `ipv4` (default) and `ipv6`. You can only specify an IP family when you create a cluster, changing this value will force a new cluster to be created.
*/
@JvmName("ybhcyijlwikiwlth")
public suspend fun ipFamily(`value`: Output) {
this.ipFamily = value
}
/**
* @param value The CIDR block to assign Kubernetes pod and service IP addresses from. If you don't specify a block, Kubernetes assigns addresses from either the 10.100.0.0/16 or 172.20.0.0/16 CIDR blocks. We recommend that you specify a block that does not overlap with resources in other networks that are peered or connected to your VPC. You can only specify a custom CIDR block when you create a cluster, changing this value will force a new cluster to be created. The block must meet the following requirements:
* * Within one of the following private IP address blocks: 10.0.0.0/8, 172.16.0.0/12, or 192.168.0.0/16.
* * Doesn't overlap with any CIDR block assigned to the VPC that you selected for VPC.
* * Between /24 and /12.
*/
@JvmName("ttmihauxfkhijqwi")
public suspend fun serviceIpv4Cidr(`value`: Output) {
this.serviceIpv4Cidr = value
}
/**
* @param value The CIDR block that Kubernetes pod and service IP addresses are assigned from if you specified `ipv6` for `ip_family` when you created the cluster. Kubernetes assigns service addresses from the unique local address range (fc00::/7) because you can't specify a custom IPv6 CIDR block when you create the cluster.
*/
@JvmName("jglwkjrryjlowhhy")
public suspend fun serviceIpv6Cidr(`value`: Output) {
this.serviceIpv6Cidr = value
}
/**
* @param value Configuration block with elastic load balancing configuration for the cluster. Detailed below.
*/
@JvmName("obdjmdmocweccsly")
public suspend fun elasticLoadBalancing(`value`: ClusterKubernetesNetworkConfigElasticLoadBalancingArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.elasticLoadBalancing = mapped
}
/**
* @param argument Configuration block with elastic load balancing configuration for the cluster. Detailed below.
*/
@JvmName("whaeliqshxdjslck")
public suspend fun elasticLoadBalancing(argument: suspend ClusterKubernetesNetworkConfigElasticLoadBalancingArgsBuilder.() -> Unit) {
val toBeMapped = ClusterKubernetesNetworkConfigElasticLoadBalancingArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.elasticLoadBalancing = mapped
}
/**
* @param value The IP family used to assign Kubernetes pod and service addresses. Valid values are `ipv4` (default) and `ipv6`. You can only specify an IP family when you create a cluster, changing this value will force a new cluster to be created.
*/
@JvmName("niunbkjkbpjliixo")
public suspend fun ipFamily(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ipFamily = mapped
}
/**
* @param value The CIDR block to assign Kubernetes pod and service IP addresses from. If you don't specify a block, Kubernetes assigns addresses from either the 10.100.0.0/16 or 172.20.0.0/16 CIDR blocks. We recommend that you specify a block that does not overlap with resources in other networks that are peered or connected to your VPC. You can only specify a custom CIDR block when you create a cluster, changing this value will force a new cluster to be created. The block must meet the following requirements:
* * Within one of the following private IP address blocks: 10.0.0.0/8, 172.16.0.0/12, or 192.168.0.0/16.
* * Doesn't overlap with any CIDR block assigned to the VPC that you selected for VPC.
* * Between /24 and /12.
*/
@JvmName("jwhcuablypxwrqlw")
public suspend fun serviceIpv4Cidr(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceIpv4Cidr = mapped
}
/**
* @param value The CIDR block that Kubernetes pod and service IP addresses are assigned from if you specified `ipv6` for `ip_family` when you created the cluster. Kubernetes assigns service addresses from the unique local address range (fc00::/7) because you can't specify a custom IPv6 CIDR block when you create the cluster.
*/
@JvmName("jngvmsnvefpdmvic")
public suspend fun serviceIpv6Cidr(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceIpv6Cidr = mapped
}
internal fun build(): ClusterKubernetesNetworkConfigArgs = ClusterKubernetesNetworkConfigArgs(
elasticLoadBalancing = elasticLoadBalancing,
ipFamily = ipFamily,
serviceIpv4Cidr = serviceIpv4Cidr,
serviceIpv6Cidr = serviceIpv6Cidr,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy