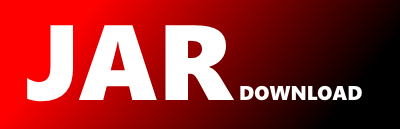
com.pulumi.aws.eks.kotlin.inputs.ClusterOutpostConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.eks.kotlin.inputs
import com.pulumi.aws.eks.inputs.ClusterOutpostConfigArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property controlPlaneInstanceType The Amazon EC2 instance type that you want to use for your local Amazon EKS cluster on Outposts. The instance type that you specify is used for all Kubernetes control plane instances. The instance type can't be changed after cluster creation. Choose an instance type based on the number of nodes that your cluster will have. If your cluster will have:
* * 1–20 nodes, then we recommend specifying a large instance type.
* * 21–100 nodes, then we recommend specifying an xlarge instance type.
* * 101–250 nodes, then we recommend specifying a 2xlarge instance type.
* For a list of the available Amazon EC2 instance types, see Compute and storage in AWS Outposts rack features The control plane is not automatically scaled by Amazon EKS.
* @property controlPlanePlacement An object representing the placement configuration for all the control plane instances of your local Amazon EKS cluster on AWS Outpost.
* The `control_plane_placement` configuration block supports the following arguments:
* @property outpostArns The ARN of the Outpost that you want to use for your local Amazon EKS cluster on Outposts. This argument is a list of arns, but only a single Outpost ARN is supported currently.
*/
public data class ClusterOutpostConfigArgs(
public val controlPlaneInstanceType: Output,
public val controlPlanePlacement: Output? = null,
public val outpostArns: Output>,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.eks.inputs.ClusterOutpostConfigArgs =
com.pulumi.aws.eks.inputs.ClusterOutpostConfigArgs.builder()
.controlPlaneInstanceType(controlPlaneInstanceType.applyValue({ args0 -> args0 }))
.controlPlanePlacement(
controlPlanePlacement?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.outpostArns(outpostArns.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [ClusterOutpostConfigArgs].
*/
@PulumiTagMarker
public class ClusterOutpostConfigArgsBuilder internal constructor() {
private var controlPlaneInstanceType: Output? = null
private var controlPlanePlacement: Output? = null
private var outpostArns: Output>? = null
/**
* @param value The Amazon EC2 instance type that you want to use for your local Amazon EKS cluster on Outposts. The instance type that you specify is used for all Kubernetes control plane instances. The instance type can't be changed after cluster creation. Choose an instance type based on the number of nodes that your cluster will have. If your cluster will have:
* * 1–20 nodes, then we recommend specifying a large instance type.
* * 21–100 nodes, then we recommend specifying an xlarge instance type.
* * 101–250 nodes, then we recommend specifying a 2xlarge instance type.
* For a list of the available Amazon EC2 instance types, see Compute and storage in AWS Outposts rack features The control plane is not automatically scaled by Amazon EKS.
*/
@JvmName("isavlkcmfxluulke")
public suspend fun controlPlaneInstanceType(`value`: Output) {
this.controlPlaneInstanceType = value
}
/**
* @param value An object representing the placement configuration for all the control plane instances of your local Amazon EKS cluster on AWS Outpost.
* The `control_plane_placement` configuration block supports the following arguments:
*/
@JvmName("wfntysjkxhssailt")
public suspend fun controlPlanePlacement(`value`: Output) {
this.controlPlanePlacement = value
}
/**
* @param value The ARN of the Outpost that you want to use for your local Amazon EKS cluster on Outposts. This argument is a list of arns, but only a single Outpost ARN is supported currently.
*/
@JvmName("fmcvfdgocahcwakn")
public suspend fun outpostArns(`value`: Output>) {
this.outpostArns = value
}
@JvmName("gcaiwnbnybmcnqip")
public suspend fun outpostArns(vararg values: Output) {
this.outpostArns = Output.all(values.asList())
}
/**
* @param values The ARN of the Outpost that you want to use for your local Amazon EKS cluster on Outposts. This argument is a list of arns, but only a single Outpost ARN is supported currently.
*/
@JvmName("quqqgxiecmrormgf")
public suspend fun outpostArns(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy