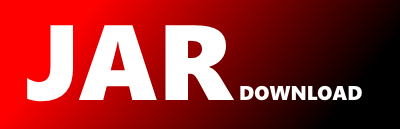
com.pulumi.aws.eks.kotlin.outputs.GetNodeGroupResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.eks.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getNodeGroup.
* @property amiType Type of Amazon Machine Image (AMI) associated with the EKS Node Group.
* @property arn ARN of the EKS Node Group.
* @property capacityType Type of capacity associated with the EKS Node Group. Valid values: `ON_DEMAND`, `SPOT`.
* @property clusterName
* @property diskSize Disk size in GiB for worker nodes.
* @property id The provider-assigned unique ID for this managed resource.
* @property instanceTypes Set of instance types associated with the EKS Node Group.
* @property labels Key-value map of Kubernetes labels. Only labels that are applied with the EKS API are managed by this argument. Other Kubernetes labels applied to the EKS Node Group will not be managed.
* @property launchTemplates Nested attribute containing information about the launch template used to create the EKS Node Group.
* @property nodeGroupName
* @property nodeRoleArn ARN of the IAM Role that provides permissions for the EKS Node Group.
* @property releaseVersion AMI version of the EKS Node Group.
* @property remoteAccesses Configuration block with remote access settings.
* @property resources List of objects containing information about underlying resources.
* @property scalingConfigs Configuration block with scaling settings.
* @property status Status of the EKS Node Group.
* @property subnetIds Identifiers of EC2 Subnets to associate with the EKS Node Group.
* @property tags Key-value map of resource tags.
* @property taints List of objects containing information about taints applied to the nodes in the EKS Node Group.
* @property version Kubernetes version.
*/
public data class GetNodeGroupResult(
public val amiType: String,
public val arn: String,
public val capacityType: String,
public val clusterName: String,
public val diskSize: Int,
public val id: String,
public val instanceTypes: List,
public val labels: Map,
public val launchTemplates: List,
public val nodeGroupName: String,
public val nodeRoleArn: String,
public val releaseVersion: String,
public val remoteAccesses: List,
public val resources: List,
public val scalingConfigs: List,
public val status: String,
public val subnetIds: List,
public val tags: Map,
public val taints: List,
public val version: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.eks.outputs.GetNodeGroupResult): GetNodeGroupResult = GetNodeGroupResult(
amiType = javaType.amiType(),
arn = javaType.arn(),
capacityType = javaType.capacityType(),
clusterName = javaType.clusterName(),
diskSize = javaType.diskSize(),
id = javaType.id(),
instanceTypes = javaType.instanceTypes().map({ args0 -> args0 }),
labels = javaType.labels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
launchTemplates = javaType.launchTemplates().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.eks.kotlin.outputs.GetNodeGroupLaunchTemplate.Companion.toKotlin(args0)
})
}),
nodeGroupName = javaType.nodeGroupName(),
nodeRoleArn = javaType.nodeRoleArn(),
releaseVersion = javaType.releaseVersion(),
remoteAccesses = javaType.remoteAccesses().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.eks.kotlin.outputs.GetNodeGroupRemoteAccess.Companion.toKotlin(args0)
})
}),
resources = javaType.resources().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.eks.kotlin.outputs.GetNodeGroupResource.Companion.toKotlin(args0)
})
}),
scalingConfigs = javaType.scalingConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.eks.kotlin.outputs.GetNodeGroupScalingConfig.Companion.toKotlin(args0)
})
}),
status = javaType.status(),
subnetIds = javaType.subnetIds().map({ args0 -> args0 }),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
taints = javaType.taints().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.eks.kotlin.outputs.GetNodeGroupTaint.Companion.toKotlin(args0)
})
}),
version = javaType.version(),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy