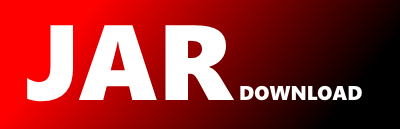
com.pulumi.aws.elasticache.kotlin.ReservedCacheNodeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.elasticache.kotlin
import com.pulumi.aws.elasticache.ReservedCacheNodeArgs.builder
import com.pulumi.aws.elasticache.kotlin.inputs.ReservedCacheNodeTimeoutsArgs
import com.pulumi.aws.elasticache.kotlin.inputs.ReservedCacheNodeTimeoutsArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Double
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Manages an ElastiCache Reserved Cache Node.
* > **NOTE:** Once created, a reservation is valid for the `duration` of the provided `offering_id` and cannot be deleted. Performing a `destroy` will only remove the resource from state. For more information see [ElastiCache Reserved Nodes Documentation](https://aws.amazon.com/elasticache/reserved-cache-nodes/) and [PurchaseReservedCacheNodesOffering](https://docs.aws.amazon.com/AmazonElastiCache/latest/APIReference/API_PurchaseReservedCacheNodesOffering.html).
* > **NOTE:** Due to the expense of testing this resource, we provide it as best effort. If you find it useful, and have the ability to help test or notice issues, consider reaching out to us on GitHub.
* ## Example Usage
*
* ```yaml
* resources:
* exampleReservedCacheNode:
* type: aws:elasticache:ReservedCacheNode
* name: example
* properties:
* reservedCacheNodesOfferingId: ${example.offeringId}
* id: optionalCustomReservationID
* cacheNodeCount: 3
* variables:
* example:
* fn::invoke:
* Function: aws:elasticache:getReservedCacheNodeOffering
* Arguments:
* cacheNodeType: cache.t4g.small
* duration: P1Y
* offeringType: No Upfront
* productDescription: redis
* ```
*
* ## Import
* Using `pulumi import`, import ElastiCache Reserved Cache Node using the `id`. For example:
* ```sh
* $ pulumi import aws:elasticache/reservedCacheNode:ReservedCacheNode example CustomReservationID
* ```
* @property cacheNodeCount Number of cache node instances to reserve.
* Default value is `1`.
* @property reservedCacheNodesOfferingId ID of the reserved cache node offering to purchase.
* To determine an `reserved_cache_nodes_offering_id`, see the `aws.elasticache.getReservedCacheNodeOffering` data source.
* The following arguments are optional:
* @property tags Map of tags to assign to the reservation. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
* @property timeouts
*/
public data class ReservedCacheNodeArgs(
public val cacheNodeCount: Output? = null,
public val reservedCacheNodesOfferingId: Output? = null,
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy