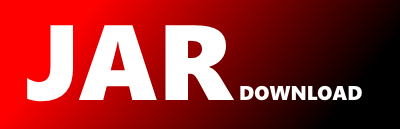
com.pulumi.aws.elasticache.kotlin.inputs.GetReservedCacheNodeOfferingPlainArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.elasticache.kotlin.inputs
import com.pulumi.aws.elasticache.inputs.GetReservedCacheNodeOfferingPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getReservedCacheNodeOffering.
* @property cacheNodeType Node type for the reserved cache node.
* See AWS documentation for information on [supported node types for Redis](https://docs.aws.amazon.com/AmazonElastiCache/latest/red-ug/CacheNodes.SupportedTypes.html) and [guidance on selecting node types for Redis](https://docs.aws.amazon.com/AmazonElastiCache/latest/red-ug/nodes-select-size.html).
* See AWS documentation for information on [supported node types for Memcached](https://docs.aws.amazon.com/AmazonElastiCache/latest/mem-ug/CacheNodes.SupportedTypes.html) and [guidance on selecting node types for Memcached](https://docs.aws.amazon.com/AmazonElastiCache/latest/mem-ug/nodes-select-size.html).
* @property duration Duration of the reservation in RFC3339 duration format.
* Valid values are `P1Y` (one year) and `P3Y` (three years).
* @property offeringType Offering type of this reserved cache node.
* For the latest generation of nodes (e.g. M5, R5, T4 and newer) valid values are `No Upfront`, `Partial Upfront`, and `All Upfront`.
* For other current generation nodes (i.e. T2, M3, M4, R3, or R4) the only valid value is `Heavy Utilization`.
* For previous generation modes (i.e. T1, M1, M2, or C1) valid values are `Heavy Utilization`, `Medium Utilization`, and `Light Utilization`.
* @property productDescription Engine type for the reserved cache node.
* Valid values are `redis`, `valkey` and `memcached`.
*/
public data class GetReservedCacheNodeOfferingPlainArgs(
public val cacheNodeType: String,
public val duration: String,
public val offeringType: String,
public val productDescription: String,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.elasticache.inputs.GetReservedCacheNodeOfferingPlainArgs =
com.pulumi.aws.elasticache.inputs.GetReservedCacheNodeOfferingPlainArgs.builder()
.cacheNodeType(cacheNodeType.let({ args0 -> args0 }))
.duration(duration.let({ args0 -> args0 }))
.offeringType(offeringType.let({ args0 -> args0 }))
.productDescription(productDescription.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetReservedCacheNodeOfferingPlainArgs].
*/
@PulumiTagMarker
public class GetReservedCacheNodeOfferingPlainArgsBuilder internal constructor() {
private var cacheNodeType: String? = null
private var duration: String? = null
private var offeringType: String? = null
private var productDescription: String? = null
/**
* @param value Node type for the reserved cache node.
* See AWS documentation for information on [supported node types for Redis](https://docs.aws.amazon.com/AmazonElastiCache/latest/red-ug/CacheNodes.SupportedTypes.html) and [guidance on selecting node types for Redis](https://docs.aws.amazon.com/AmazonElastiCache/latest/red-ug/nodes-select-size.html).
* See AWS documentation for information on [supported node types for Memcached](https://docs.aws.amazon.com/AmazonElastiCache/latest/mem-ug/CacheNodes.SupportedTypes.html) and [guidance on selecting node types for Memcached](https://docs.aws.amazon.com/AmazonElastiCache/latest/mem-ug/nodes-select-size.html).
*/
@JvmName("plqwkskifmwgnqpa")
public suspend fun cacheNodeType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.cacheNodeType = mapped
}
/**
* @param value Duration of the reservation in RFC3339 duration format.
* Valid values are `P1Y` (one year) and `P3Y` (three years).
*/
@JvmName("eutsyhinstnyeheu")
public suspend fun duration(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.duration = mapped
}
/**
* @param value Offering type of this reserved cache node.
* For the latest generation of nodes (e.g. M5, R5, T4 and newer) valid values are `No Upfront`, `Partial Upfront`, and `All Upfront`.
* For other current generation nodes (i.e. T2, M3, M4, R3, or R4) the only valid value is `Heavy Utilization`.
* For previous generation modes (i.e. T1, M1, M2, or C1) valid values are `Heavy Utilization`, `Medium Utilization`, and `Light Utilization`.
*/
@JvmName("huihmormcfjxilbv")
public suspend fun offeringType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.offeringType = mapped
}
/**
* @param value Engine type for the reserved cache node.
* Valid values are `redis`, `valkey` and `memcached`.
*/
@JvmName("mgwgcmecmwthduex")
public suspend fun productDescription(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.productDescription = mapped
}
internal fun build(): GetReservedCacheNodeOfferingPlainArgs =
GetReservedCacheNodeOfferingPlainArgs(
cacheNodeType = cacheNodeType ?: throw PulumiNullFieldException("cacheNodeType"),
duration = duration ?: throw PulumiNullFieldException("duration"),
offeringType = offeringType ?: throw PulumiNullFieldException("offeringType"),
productDescription = productDescription ?: throw PulumiNullFieldException("productDescription"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy