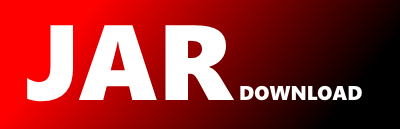
com.pulumi.aws.elasticache.kotlin.inputs.GetUserPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.elasticache.kotlin.inputs
import com.pulumi.aws.elasticache.inputs.GetUserPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getUser.
* @property accessString String for what access a user possesses within the associated ElastiCache replication groups or clusters.
* @property authenticationModes
* @property engine
* @property noPasswordRequired
* @property passwords
* @property userId Identifier for the user.
* @property userName User name of the user.
*/
public data class GetUserPlainArgs(
public val accessString: String? = null,
public val authenticationModes: List? = null,
public val engine: String? = null,
public val noPasswordRequired: Boolean? = null,
public val passwords: List? = null,
public val userId: String,
public val userName: String? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.elasticache.inputs.GetUserPlainArgs =
com.pulumi.aws.elasticache.inputs.GetUserPlainArgs.builder()
.accessString(accessString?.let({ args0 -> args0 }))
.authenticationModes(
authenticationModes?.let({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.engine(engine?.let({ args0 -> args0 }))
.noPasswordRequired(noPasswordRequired?.let({ args0 -> args0 }))
.passwords(passwords?.let({ args0 -> args0.map({ args0 -> args0 }) }))
.userId(userId.let({ args0 -> args0 }))
.userName(userName?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetUserPlainArgs].
*/
@PulumiTagMarker
public class GetUserPlainArgsBuilder internal constructor() {
private var accessString: String? = null
private var authenticationModes: List? = null
private var engine: String? = null
private var noPasswordRequired: Boolean? = null
private var passwords: List? = null
private var userId: String? = null
private var userName: String? = null
/**
* @param value String for what access a user possesses within the associated ElastiCache replication groups or clusters.
*/
@JvmName("mbdierepyxlxtejo")
public suspend fun accessString(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.accessString = mapped
}
/**
* @param value
*/
@JvmName("fdbjwiubxwojsbov")
public suspend fun authenticationModes(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.authenticationModes = mapped
}
/**
* @param argument
*/
@JvmName("ebpcjjqcsvingwcb")
public suspend fun authenticationModes(argument: List Unit>) {
val toBeMapped = argument.toList().map {
GetUserAuthenticationModeBuilder().applySuspend {
it()
}.build()
}
val mapped = toBeMapped
this.authenticationModes = mapped
}
/**
* @param argument
*/
@JvmName("grtlgulxyjwfgvmw")
public suspend fun authenticationModes(vararg argument: suspend GetUserAuthenticationModeBuilder.() -> Unit) {
val toBeMapped = argument.toList().map {
GetUserAuthenticationModeBuilder().applySuspend {
it()
}.build()
}
val mapped = toBeMapped
this.authenticationModes = mapped
}
/**
* @param argument
*/
@JvmName("ubmtaeguuswjqmqy")
public suspend fun authenticationModes(argument: suspend GetUserAuthenticationModeBuilder.() -> Unit) {
val toBeMapped = listOf(GetUserAuthenticationModeBuilder().applySuspend { argument() }.build())
val mapped = toBeMapped
this.authenticationModes = mapped
}
/**
* @param values
*/
@JvmName("tkfsxpuexyfapeuo")
public suspend fun authenticationModes(vararg values: GetUserAuthenticationMode) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.authenticationModes = mapped
}
/**
* @param value
*/
@JvmName("ksulgggbdculmebp")
public suspend fun engine(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.engine = mapped
}
/**
* @param value
*/
@JvmName("opkcowkegmmqsrcf")
public suspend fun noPasswordRequired(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.noPasswordRequired = mapped
}
/**
* @param value
*/
@JvmName("jjecfiitxxuospql")
public suspend fun passwords(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.passwords = mapped
}
/**
* @param values
*/
@JvmName("ohrpbfrcntejfpws")
public suspend fun passwords(vararg values: String) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.passwords = mapped
}
/**
* @param value Identifier for the user.
*/
@JvmName("dhsswxulgdalrbat")
public suspend fun userId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.userId = mapped
}
/**
* @param value User name of the user.
*/
@JvmName("rlpdcjnhxxeobmyh")
public suspend fun userName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.userName = mapped
}
internal fun build(): GetUserPlainArgs = GetUserPlainArgs(
accessString = accessString,
authenticationModes = authenticationModes,
engine = engine,
noPasswordRequired = noPasswordRequired,
passwords = passwords,
userId = userId ?: throw PulumiNullFieldException("userId"),
userName = userName,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy