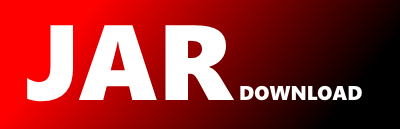
com.pulumi.aws.elasticache.kotlin.outputs.GetReplicationGroupResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.elasticache.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
* A collection of values returned by getReplicationGroup.
* @property arn ARN of the created ElastiCache Replication Group.
* @property authTokenEnabled Whether an AuthToken (password) is enabled.
* @property automaticFailoverEnabled A flag whether a read-only replica will be automatically promoted to read/write primary if the existing primary fails.
* @property clusterMode Whether cluster mode is enabled or disabled.
* @property configurationEndpointAddress The configuration endpoint address to allow host discovery.
* @property description Description of the replication group.
* @property id The provider-assigned unique ID for this managed resource.
* @property logDeliveryConfigurations Redis [SLOWLOG](https://redis.io/commands/slowlog) or Redis [Engine Log](https://docs.aws.amazon.com/AmazonElastiCache/latest/red-ug/Log_Delivery.html#Log_contents-engine-log) delivery settings.
* @property memberClusters Identifiers of all the nodes that are part of this replication group.
* @property multiAzEnabled Whether Multi-AZ Support is enabled for the replication group.
* @property nodeType The cluster node type.
* @property numCacheClusters The number of cache clusters that the replication group has.
* @property numNodeGroups Number of node groups (shards) for the replication group.
* @property port The port number on which the configuration endpoint will accept connections.
* @property primaryEndpointAddress The endpoint of the primary node in this node group (shard).
* @property readerEndpointAddress The endpoint of the reader node in this node group (shard).
* @property replicasPerNodeGroup Number of replica nodes in each node group.
* @property replicationGroupId
* @property snapshotRetentionLimit The number of days for which ElastiCache retains automatic cache cluster snapshots before deleting them.
* @property snapshotWindow Daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group (shard).
*/
public data class GetReplicationGroupResult(
public val arn: String,
public val authTokenEnabled: Boolean,
public val automaticFailoverEnabled: Boolean,
public val clusterMode: String,
public val configurationEndpointAddress: String,
public val description: String,
public val id: String,
public val logDeliveryConfigurations: List,
public val memberClusters: List,
public val multiAzEnabled: Boolean,
public val nodeType: String,
public val numCacheClusters: Int,
public val numNodeGroups: Int,
public val port: Int,
public val primaryEndpointAddress: String,
public val readerEndpointAddress: String,
public val replicasPerNodeGroup: Int,
public val replicationGroupId: String,
public val snapshotRetentionLimit: Int,
public val snapshotWindow: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.elasticache.outputs.GetReplicationGroupResult): GetReplicationGroupResult = GetReplicationGroupResult(
arn = javaType.arn(),
authTokenEnabled = javaType.authTokenEnabled(),
automaticFailoverEnabled = javaType.automaticFailoverEnabled(),
clusterMode = javaType.clusterMode(),
configurationEndpointAddress = javaType.configurationEndpointAddress(),
description = javaType.description(),
id = javaType.id(),
logDeliveryConfigurations = javaType.logDeliveryConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.elasticache.kotlin.outputs.GetReplicationGroupLogDeliveryConfiguration.Companion.toKotlin(args0)
})
}),
memberClusters = javaType.memberClusters().map({ args0 -> args0 }),
multiAzEnabled = javaType.multiAzEnabled(),
nodeType = javaType.nodeType(),
numCacheClusters = javaType.numCacheClusters(),
numNodeGroups = javaType.numNodeGroups(),
port = javaType.port(),
primaryEndpointAddress = javaType.primaryEndpointAddress(),
readerEndpointAddress = javaType.readerEndpointAddress(),
replicasPerNodeGroup = javaType.replicasPerNodeGroup(),
replicationGroupId = javaType.replicationGroupId(),
snapshotRetentionLimit = javaType.snapshotRetentionLimit(),
snapshotWindow = javaType.snapshotWindow(),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy