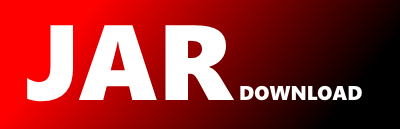
com.pulumi.aws.elasticbeanstalk.kotlin.ConfigurationTemplateArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.elasticbeanstalk.kotlin
import com.pulumi.aws.elasticbeanstalk.ConfigurationTemplateArgs.builder
import com.pulumi.aws.elasticbeanstalk.kotlin.inputs.ConfigurationTemplateSettingArgs
import com.pulumi.aws.elasticbeanstalk.kotlin.inputs.ConfigurationTemplateSettingArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Provides an Elastic Beanstalk Configuration Template, which are associated with
* a specific application and are used to deploy different versions of the
* application with the same configuration settings.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const tftest = new aws.elasticbeanstalk.Application("tftest", {
* name: "tf-test-name",
* description: "tf-test-desc",
* });
* const myTemplate = new aws.elasticbeanstalk.ConfigurationTemplate("my_template", {
* name: "tf-test-template-config",
* application: tftest.name,
* solutionStackName: "64bit Amazon Linux 2015.09 v2.0.8 running Go 1.4",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* tftest = aws.elasticbeanstalk.Application("tftest",
* name="tf-test-name",
* description="tf-test-desc")
* my_template = aws.elasticbeanstalk.ConfigurationTemplate("my_template",
* name="tf-test-template-config",
* application=tftest.name,
* solution_stack_name="64bit Amazon Linux 2015.09 v2.0.8 running Go 1.4")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var tftest = new Aws.ElasticBeanstalk.Application("tftest", new()
* {
* Name = "tf-test-name",
* Description = "tf-test-desc",
* });
* var myTemplate = new Aws.ElasticBeanstalk.ConfigurationTemplate("my_template", new()
* {
* Name = "tf-test-template-config",
* Application = tftest.Name,
* SolutionStackName = "64bit Amazon Linux 2015.09 v2.0.8 running Go 1.4",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/elasticbeanstalk"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* tftest, err := elasticbeanstalk.NewApplication(ctx, "tftest", &elasticbeanstalk.ApplicationArgs{
* Name: pulumi.String("tf-test-name"),
* Description: pulumi.String("tf-test-desc"),
* })
* if err != nil {
* return err
* }
* _, err = elasticbeanstalk.NewConfigurationTemplate(ctx, "my_template", &elasticbeanstalk.ConfigurationTemplateArgs{
* Name: pulumi.String("tf-test-template-config"),
* Application: tftest.Name,
* SolutionStackName: pulumi.String("64bit Amazon Linux 2015.09 v2.0.8 running Go 1.4"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.elasticbeanstalk.Application;
* import com.pulumi.aws.elasticbeanstalk.ApplicationArgs;
* import com.pulumi.aws.elasticbeanstalk.ConfigurationTemplate;
* import com.pulumi.aws.elasticbeanstalk.ConfigurationTemplateArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var tftest = new Application("tftest", ApplicationArgs.builder()
* .name("tf-test-name")
* .description("tf-test-desc")
* .build());
* var myTemplate = new ConfigurationTemplate("myTemplate", ConfigurationTemplateArgs.builder()
* .name("tf-test-template-config")
* .application(tftest.name())
* .solutionStackName("64bit Amazon Linux 2015.09 v2.0.8 running Go 1.4")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* tftest:
* type: aws:elasticbeanstalk:Application
* properties:
* name: tf-test-name
* description: tf-test-desc
* myTemplate:
* type: aws:elasticbeanstalk:ConfigurationTemplate
* name: my_template
* properties:
* name: tf-test-template-config
* application: ${tftest.name}
* solutionStackName: 64bit Amazon Linux 2015.09 v2.0.8 running Go 1.4
* ```
*
* ## Option Settings
* The `setting` field supports the following format:
* * `namespace` - unique namespace identifying the option's associated AWS resource
* * `name` - name of the configuration option
* * `value` - value for the configuration option
* * `resource` - (Optional) resource name for [scheduled action](https://docs.aws.amazon.com/elasticbeanstalk/latest/dg/command-options-general.html#command-options-general-autoscalingscheduledaction)
* @property application name of the application to associate with this configuration template
* @property description Short description of the Template
* @property environmentId The ID of the environment used with this configuration template
* @property name A unique name for this Template.
* @property settings Option settings to configure the new Environment. These
* override specific values that are set as defaults. The format is detailed
* below in Option Settings
* @property solutionStackName A solution stack to base your Template
* off of. Example stacks can be found in the [Amazon API documentation][1]
*/
public data class ConfigurationTemplateArgs(
public val application: Output? = null,
public val description: Output? = null,
public val environmentId: Output? = null,
public val name: Output? = null,
public val settings: Output>? = null,
public val solutionStackName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.elasticbeanstalk.ConfigurationTemplateArgs =
com.pulumi.aws.elasticbeanstalk.ConfigurationTemplateArgs.builder()
.application(application?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.environmentId(environmentId?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.settings(
settings?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.solutionStackName(solutionStackName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ConfigurationTemplateArgs].
*/
@PulumiTagMarker
public class ConfigurationTemplateArgsBuilder internal constructor() {
private var application: Output? = null
private var description: Output? = null
private var environmentId: Output? = null
private var name: Output? = null
private var settings: Output>? = null
private var solutionStackName: Output? = null
/**
* @param value name of the application to associate with this configuration template
*/
@JvmName("wdnhcnlqqvwscdaw")
public suspend fun application(`value`: Output) {
this.application = value
}
/**
* @param value Short description of the Template
*/
@JvmName("urpyhkdvfgpoyrnv")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The ID of the environment used with this configuration template
*/
@JvmName("tqtovlubyaqkujxh")
public suspend fun environmentId(`value`: Output) {
this.environmentId = value
}
/**
* @param value A unique name for this Template.
*/
@JvmName("jupnatbqeneoqmod")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Option settings to configure the new Environment. These
* override specific values that are set as defaults. The format is detailed
* below in Option Settings
*/
@JvmName("mwluscgsrmmsfwby")
public suspend fun settings(`value`: Output>) {
this.settings = value
}
@JvmName("xuwdiryyerihdafl")
public suspend fun settings(vararg values: Output) {
this.settings = Output.all(values.asList())
}
/**
* @param values Option settings to configure the new Environment. These
* override specific values that are set as defaults. The format is detailed
* below in Option Settings
*/
@JvmName("svaucplgbnyxlufv")
public suspend fun settings(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy