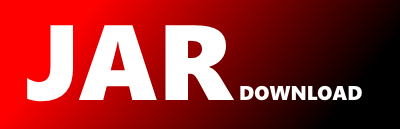
com.pulumi.aws.elasticbeanstalk.kotlin.inputs.ApplicationAppversionLifecycleArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.elasticbeanstalk.kotlin.inputs
import com.pulumi.aws.elasticbeanstalk.inputs.ApplicationAppversionLifecycleArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property deleteSourceFromS3 Set to `true` to delete a version's source bundle from S3 when the application version is deleted.
* @property maxAgeInDays The number of days to retain an application version ('max_age_in_days' and 'max_count' cannot be enabled simultaneously.).
* @property maxCount The maximum number of application versions to retain ('max_age_in_days' and 'max_count' cannot be enabled simultaneously.).
* @property serviceRole The ARN of an IAM service role under which the application version is deleted. Elastic Beanstalk must have permission to assume this role.
*/
public data class ApplicationAppversionLifecycleArgs(
public val deleteSourceFromS3: Output? = null,
public val maxAgeInDays: Output? = null,
public val maxCount: Output? = null,
public val serviceRole: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.elasticbeanstalk.inputs.ApplicationAppversionLifecycleArgs =
com.pulumi.aws.elasticbeanstalk.inputs.ApplicationAppversionLifecycleArgs.builder()
.deleteSourceFromS3(deleteSourceFromS3?.applyValue({ args0 -> args0 }))
.maxAgeInDays(maxAgeInDays?.applyValue({ args0 -> args0 }))
.maxCount(maxCount?.applyValue({ args0 -> args0 }))
.serviceRole(serviceRole.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ApplicationAppversionLifecycleArgs].
*/
@PulumiTagMarker
public class ApplicationAppversionLifecycleArgsBuilder internal constructor() {
private var deleteSourceFromS3: Output? = null
private var maxAgeInDays: Output? = null
private var maxCount: Output? = null
private var serviceRole: Output? = null
/**
* @param value Set to `true` to delete a version's source bundle from S3 when the application version is deleted.
*/
@JvmName("yhdsavwouifsplul")
public suspend fun deleteSourceFromS3(`value`: Output) {
this.deleteSourceFromS3 = value
}
/**
* @param value The number of days to retain an application version ('max_age_in_days' and 'max_count' cannot be enabled simultaneously.).
*/
@JvmName("caalciufpmheacdb")
public suspend fun maxAgeInDays(`value`: Output) {
this.maxAgeInDays = value
}
/**
* @param value The maximum number of application versions to retain ('max_age_in_days' and 'max_count' cannot be enabled simultaneously.).
*/
@JvmName("flqjuixqqsgiecxi")
public suspend fun maxCount(`value`: Output) {
this.maxCount = value
}
/**
* @param value The ARN of an IAM service role under which the application version is deleted. Elastic Beanstalk must have permission to assume this role.
*/
@JvmName("spyqrclqegaocwvd")
public suspend fun serviceRole(`value`: Output) {
this.serviceRole = value
}
/**
* @param value Set to `true` to delete a version's source bundle from S3 when the application version is deleted.
*/
@JvmName("yudbbkpsflvdjfuo")
public suspend fun deleteSourceFromS3(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deleteSourceFromS3 = mapped
}
/**
* @param value The number of days to retain an application version ('max_age_in_days' and 'max_count' cannot be enabled simultaneously.).
*/
@JvmName("fjjkjnnfinevuvuk")
public suspend fun maxAgeInDays(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxAgeInDays = mapped
}
/**
* @param value The maximum number of application versions to retain ('max_age_in_days' and 'max_count' cannot be enabled simultaneously.).
*/
@JvmName("dldntsrkoibwsnxx")
public suspend fun maxCount(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxCount = mapped
}
/**
* @param value The ARN of an IAM service role under which the application version is deleted. Elastic Beanstalk must have permission to assume this role.
*/
@JvmName("ilskpygrqejeapfc")
public suspend fun serviceRole(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.serviceRole = mapped
}
internal fun build(): ApplicationAppversionLifecycleArgs = ApplicationAppversionLifecycleArgs(
deleteSourceFromS3 = deleteSourceFromS3,
maxAgeInDays = maxAgeInDays,
maxCount = maxCount,
serviceRole = serviceRole ?: throw PulumiNullFieldException("serviceRole"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy