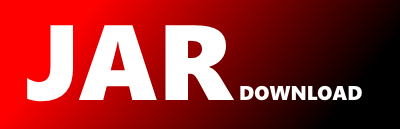
com.pulumi.aws.elastictranscoder.kotlin.inputs.PresetVideoArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.elastictranscoder.kotlin.inputs
import com.pulumi.aws.elastictranscoder.inputs.PresetVideoArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property aspectRatio The display aspect ratio of the video in the output file. Valid values are: `auto`, `1:1`, `4:3`, `3:2`, `16:9`. (Note; to better control resolution and aspect ratio of output videos, we recommend that you use the values `max_width`, `max_height`, `sizing_policy`, `padding_policy`, and `display_aspect_ratio` instead of `resolution` and `aspect_ratio`.)
* @property bitRate The bit rate of the video stream in the output file, in kilobits/second. You can configure variable bit rate or constant bit rate encoding.
* @property codec The video codec for the output file. Valid values are `gif`, `H.264`, `mpeg2`, `vp8`, and `vp9`.
* @property displayAspectRatio The value that Elastic Transcoder adds to the metadata in the output file. If you set DisplayAspectRatio to auto, Elastic Transcoder chooses an aspect ratio that ensures square pixels. If you specify another option, Elastic Transcoder sets that value in the output file.
* @property fixedGop Whether to use a fixed value for Video:FixedGOP. Not applicable for containers of type gif. Valid values are true and false. Also known as, Fixed Number of Frames Between Keyframes.
* @property frameRate The frames per second for the video stream in the output file. The following values are valid: `auto`, `10`, `15`, `23.97`, `24`, `25`, `29.97`, `30`, `50`, `60`.
* @property keyframesMaxDist The maximum number of frames between key frames. Not applicable for containers of type gif.
* @property maxFrameRate If you specify auto for FrameRate, Elastic Transcoder uses the frame rate of the input video for the frame rate of the output video, up to the maximum frame rate. If you do not specify a MaxFrameRate, Elastic Transcoder will use a default of 30.
* @property maxHeight The maximum height of the output video in pixels. If you specify auto, Elastic Transcoder uses 1080 (Full HD) as the default value. If you specify a numeric value, enter an even integer between 96 and 3072, inclusive.
* @property maxWidth The maximum width of the output video in pixels. If you specify auto, Elastic Transcoder uses 1920 (Full HD) as the default value. If you specify a numeric value, enter an even integer between 128 and 4096, inclusive.
* @property paddingPolicy When you set PaddingPolicy to Pad, Elastic Transcoder might add black bars to the top and bottom and/or left and right sides of the output video to make the total size of the output video match the values that you specified for `max_width` and `max_height`.
* @property resolution The width and height of the video in the output file, in pixels. Valid values are `auto` and `widthxheight`. (see note for `aspect_ratio`)
* @property sizingPolicy A value that controls scaling of the output video. Valid values are: `Fit`, `Fill`, `Stretch`, `Keep`, `ShrinkToFit`, `ShrinkToFill`.
*/
public data class PresetVideoArgs(
public val aspectRatio: Output? = null,
public val bitRate: Output? = null,
public val codec: Output? = null,
public val displayAspectRatio: Output? = null,
public val fixedGop: Output? = null,
public val frameRate: Output? = null,
public val keyframesMaxDist: Output? = null,
public val maxFrameRate: Output? = null,
public val maxHeight: Output? = null,
public val maxWidth: Output? = null,
public val paddingPolicy: Output? = null,
public val resolution: Output? = null,
public val sizingPolicy: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.elastictranscoder.inputs.PresetVideoArgs =
com.pulumi.aws.elastictranscoder.inputs.PresetVideoArgs.builder()
.aspectRatio(aspectRatio?.applyValue({ args0 -> args0 }))
.bitRate(bitRate?.applyValue({ args0 -> args0 }))
.codec(codec?.applyValue({ args0 -> args0 }))
.displayAspectRatio(displayAspectRatio?.applyValue({ args0 -> args0 }))
.fixedGop(fixedGop?.applyValue({ args0 -> args0 }))
.frameRate(frameRate?.applyValue({ args0 -> args0 }))
.keyframesMaxDist(keyframesMaxDist?.applyValue({ args0 -> args0 }))
.maxFrameRate(maxFrameRate?.applyValue({ args0 -> args0 }))
.maxHeight(maxHeight?.applyValue({ args0 -> args0 }))
.maxWidth(maxWidth?.applyValue({ args0 -> args0 }))
.paddingPolicy(paddingPolicy?.applyValue({ args0 -> args0 }))
.resolution(resolution?.applyValue({ args0 -> args0 }))
.sizingPolicy(sizingPolicy?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PresetVideoArgs].
*/
@PulumiTagMarker
public class PresetVideoArgsBuilder internal constructor() {
private var aspectRatio: Output? = null
private var bitRate: Output? = null
private var codec: Output? = null
private var displayAspectRatio: Output? = null
private var fixedGop: Output? = null
private var frameRate: Output? = null
private var keyframesMaxDist: Output? = null
private var maxFrameRate: Output? = null
private var maxHeight: Output? = null
private var maxWidth: Output? = null
private var paddingPolicy: Output? = null
private var resolution: Output? = null
private var sizingPolicy: Output? = null
/**
* @param value The display aspect ratio of the video in the output file. Valid values are: `auto`, `1:1`, `4:3`, `3:2`, `16:9`. (Note; to better control resolution and aspect ratio of output videos, we recommend that you use the values `max_width`, `max_height`, `sizing_policy`, `padding_policy`, and `display_aspect_ratio` instead of `resolution` and `aspect_ratio`.)
*/
@JvmName("jcovwgrbkeiactnk")
public suspend fun aspectRatio(`value`: Output) {
this.aspectRatio = value
}
/**
* @param value The bit rate of the video stream in the output file, in kilobits/second. You can configure variable bit rate or constant bit rate encoding.
*/
@JvmName("wbuixgfiaooxxepn")
public suspend fun bitRate(`value`: Output) {
this.bitRate = value
}
/**
* @param value The video codec for the output file. Valid values are `gif`, `H.264`, `mpeg2`, `vp8`, and `vp9`.
*/
@JvmName("hepbkigsvyajhutc")
public suspend fun codec(`value`: Output) {
this.codec = value
}
/**
* @param value The value that Elastic Transcoder adds to the metadata in the output file. If you set DisplayAspectRatio to auto, Elastic Transcoder chooses an aspect ratio that ensures square pixels. If you specify another option, Elastic Transcoder sets that value in the output file.
*/
@JvmName("aqkbxjlkcrnqqvoq")
public suspend fun displayAspectRatio(`value`: Output) {
this.displayAspectRatio = value
}
/**
* @param value Whether to use a fixed value for Video:FixedGOP. Not applicable for containers of type gif. Valid values are true and false. Also known as, Fixed Number of Frames Between Keyframes.
*/
@JvmName("ywvkqrctpacjfwhv")
public suspend fun fixedGop(`value`: Output) {
this.fixedGop = value
}
/**
* @param value The frames per second for the video stream in the output file. The following values are valid: `auto`, `10`, `15`, `23.97`, `24`, `25`, `29.97`, `30`, `50`, `60`.
*/
@JvmName("nyslgxsbasntosun")
public suspend fun frameRate(`value`: Output) {
this.frameRate = value
}
/**
* @param value The maximum number of frames between key frames. Not applicable for containers of type gif.
*/
@JvmName("cpttwbmmugasbdrb")
public suspend fun keyframesMaxDist(`value`: Output) {
this.keyframesMaxDist = value
}
/**
* @param value If you specify auto for FrameRate, Elastic Transcoder uses the frame rate of the input video for the frame rate of the output video, up to the maximum frame rate. If you do not specify a MaxFrameRate, Elastic Transcoder will use a default of 30.
*/
@JvmName("rfquximbbpugrtnl")
public suspend fun maxFrameRate(`value`: Output) {
this.maxFrameRate = value
}
/**
* @param value The maximum height of the output video in pixels. If you specify auto, Elastic Transcoder uses 1080 (Full HD) as the default value. If you specify a numeric value, enter an even integer between 96 and 3072, inclusive.
*/
@JvmName("fqdrxtedklhdrnoa")
public suspend fun maxHeight(`value`: Output) {
this.maxHeight = value
}
/**
* @param value The maximum width of the output video in pixels. If you specify auto, Elastic Transcoder uses 1920 (Full HD) as the default value. If you specify a numeric value, enter an even integer between 128 and 4096, inclusive.
*/
@JvmName("iynuoibkxdoaqtre")
public suspend fun maxWidth(`value`: Output) {
this.maxWidth = value
}
/**
* @param value When you set PaddingPolicy to Pad, Elastic Transcoder might add black bars to the top and bottom and/or left and right sides of the output video to make the total size of the output video match the values that you specified for `max_width` and `max_height`.
*/
@JvmName("losacqnlmpdqofjd")
public suspend fun paddingPolicy(`value`: Output) {
this.paddingPolicy = value
}
/**
* @param value The width and height of the video in the output file, in pixels. Valid values are `auto` and `widthxheight`. (see note for `aspect_ratio`)
*/
@JvmName("ebbdlxacxskrfwkv")
public suspend fun resolution(`value`: Output) {
this.resolution = value
}
/**
* @param value A value that controls scaling of the output video. Valid values are: `Fit`, `Fill`, `Stretch`, `Keep`, `ShrinkToFit`, `ShrinkToFill`.
*/
@JvmName("mpgotlejpwpcdiot")
public suspend fun sizingPolicy(`value`: Output) {
this.sizingPolicy = value
}
/**
* @param value The display aspect ratio of the video in the output file. Valid values are: `auto`, `1:1`, `4:3`, `3:2`, `16:9`. (Note; to better control resolution and aspect ratio of output videos, we recommend that you use the values `max_width`, `max_height`, `sizing_policy`, `padding_policy`, and `display_aspect_ratio` instead of `resolution` and `aspect_ratio`.)
*/
@JvmName("xipyxwiultkcivic")
public suspend fun aspectRatio(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.aspectRatio = mapped
}
/**
* @param value The bit rate of the video stream in the output file, in kilobits/second. You can configure variable bit rate or constant bit rate encoding.
*/
@JvmName("hogrkwmmrsyslcfj")
public suspend fun bitRate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bitRate = mapped
}
/**
* @param value The video codec for the output file. Valid values are `gif`, `H.264`, `mpeg2`, `vp8`, and `vp9`.
*/
@JvmName("forjjftubgqfwhue")
public suspend fun codec(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.codec = mapped
}
/**
* @param value The value that Elastic Transcoder adds to the metadata in the output file. If you set DisplayAspectRatio to auto, Elastic Transcoder chooses an aspect ratio that ensures square pixels. If you specify another option, Elastic Transcoder sets that value in the output file.
*/
@JvmName("cljuefqirrsendfb")
public suspend fun displayAspectRatio(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.displayAspectRatio = mapped
}
/**
* @param value Whether to use a fixed value for Video:FixedGOP. Not applicable for containers of type gif. Valid values are true and false. Also known as, Fixed Number of Frames Between Keyframes.
*/
@JvmName("hfndsnbleaawncag")
public suspend fun fixedGop(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fixedGop = mapped
}
/**
* @param value The frames per second for the video stream in the output file. The following values are valid: `auto`, `10`, `15`, `23.97`, `24`, `25`, `29.97`, `30`, `50`, `60`.
*/
@JvmName("oskrpqstyoxtromr")
public suspend fun frameRate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.frameRate = mapped
}
/**
* @param value The maximum number of frames between key frames. Not applicable for containers of type gif.
*/
@JvmName("xfbjvsibnduvvecg")
public suspend fun keyframesMaxDist(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keyframesMaxDist = mapped
}
/**
* @param value If you specify auto for FrameRate, Elastic Transcoder uses the frame rate of the input video for the frame rate of the output video, up to the maximum frame rate. If you do not specify a MaxFrameRate, Elastic Transcoder will use a default of 30.
*/
@JvmName("xhmeknpgcjorlcog")
public suspend fun maxFrameRate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxFrameRate = mapped
}
/**
* @param value The maximum height of the output video in pixels. If you specify auto, Elastic Transcoder uses 1080 (Full HD) as the default value. If you specify a numeric value, enter an even integer between 96 and 3072, inclusive.
*/
@JvmName("bovvycuhinbhucoy")
public suspend fun maxHeight(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxHeight = mapped
}
/**
* @param value The maximum width of the output video in pixels. If you specify auto, Elastic Transcoder uses 1920 (Full HD) as the default value. If you specify a numeric value, enter an even integer between 128 and 4096, inclusive.
*/
@JvmName("lccagcupamdwceib")
public suspend fun maxWidth(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxWidth = mapped
}
/**
* @param value When you set PaddingPolicy to Pad, Elastic Transcoder might add black bars to the top and bottom and/or left and right sides of the output video to make the total size of the output video match the values that you specified for `max_width` and `max_height`.
*/
@JvmName("chtjlqulcurguhnw")
public suspend fun paddingPolicy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.paddingPolicy = mapped
}
/**
* @param value The width and height of the video in the output file, in pixels. Valid values are `auto` and `widthxheight`. (see note for `aspect_ratio`)
*/
@JvmName("msrngjaimbogvfwu")
public suspend fun resolution(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resolution = mapped
}
/**
* @param value A value that controls scaling of the output video. Valid values are: `Fit`, `Fill`, `Stretch`, `Keep`, `ShrinkToFit`, `ShrinkToFill`.
*/
@JvmName("vbvahnaxgosqlfus")
public suspend fun sizingPolicy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sizingPolicy = mapped
}
internal fun build(): PresetVideoArgs = PresetVideoArgs(
aspectRatio = aspectRatio,
bitRate = bitRate,
codec = codec,
displayAspectRatio = displayAspectRatio,
fixedGop = fixedGop,
frameRate = frameRate,
keyframesMaxDist = keyframesMaxDist,
maxFrameRate = maxFrameRate,
maxHeight = maxHeight,
maxWidth = maxWidth,
paddingPolicy = paddingPolicy,
resolution = resolution,
sizingPolicy = sizingPolicy,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy