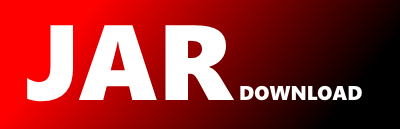
com.pulumi.aws.elastictranscoder.kotlin.outputs.PresetVideo.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.elastictranscoder.kotlin.outputs
import kotlin.String
import kotlin.Suppress
/**
*
* @property aspectRatio The display aspect ratio of the video in the output file. Valid values are: `auto`, `1:1`, `4:3`, `3:2`, `16:9`. (Note; to better control resolution and aspect ratio of output videos, we recommend that you use the values `max_width`, `max_height`, `sizing_policy`, `padding_policy`, and `display_aspect_ratio` instead of `resolution` and `aspect_ratio`.)
* @property bitRate The bit rate of the video stream in the output file, in kilobits/second. You can configure variable bit rate or constant bit rate encoding.
* @property codec The video codec for the output file. Valid values are `gif`, `H.264`, `mpeg2`, `vp8`, and `vp9`.
* @property displayAspectRatio The value that Elastic Transcoder adds to the metadata in the output file. If you set DisplayAspectRatio to auto, Elastic Transcoder chooses an aspect ratio that ensures square pixels. If you specify another option, Elastic Transcoder sets that value in the output file.
* @property fixedGop Whether to use a fixed value for Video:FixedGOP. Not applicable for containers of type gif. Valid values are true and false. Also known as, Fixed Number of Frames Between Keyframes.
* @property frameRate The frames per second for the video stream in the output file. The following values are valid: `auto`, `10`, `15`, `23.97`, `24`, `25`, `29.97`, `30`, `50`, `60`.
* @property keyframesMaxDist The maximum number of frames between key frames. Not applicable for containers of type gif.
* @property maxFrameRate If you specify auto for FrameRate, Elastic Transcoder uses the frame rate of the input video for the frame rate of the output video, up to the maximum frame rate. If you do not specify a MaxFrameRate, Elastic Transcoder will use a default of 30.
* @property maxHeight The maximum height of the output video in pixels. If you specify auto, Elastic Transcoder uses 1080 (Full HD) as the default value. If you specify a numeric value, enter an even integer between 96 and 3072, inclusive.
* @property maxWidth The maximum width of the output video in pixels. If you specify auto, Elastic Transcoder uses 1920 (Full HD) as the default value. If you specify a numeric value, enter an even integer between 128 and 4096, inclusive.
* @property paddingPolicy When you set PaddingPolicy to Pad, Elastic Transcoder might add black bars to the top and bottom and/or left and right sides of the output video to make the total size of the output video match the values that you specified for `max_width` and `max_height`.
* @property resolution The width and height of the video in the output file, in pixels. Valid values are `auto` and `widthxheight`. (see note for `aspect_ratio`)
* @property sizingPolicy A value that controls scaling of the output video. Valid values are: `Fit`, `Fill`, `Stretch`, `Keep`, `ShrinkToFit`, `ShrinkToFill`.
*/
public data class PresetVideo(
public val aspectRatio: String? = null,
public val bitRate: String? = null,
public val codec: String? = null,
public val displayAspectRatio: String? = null,
public val fixedGop: String? = null,
public val frameRate: String? = null,
public val keyframesMaxDist: String? = null,
public val maxFrameRate: String? = null,
public val maxHeight: String? = null,
public val maxWidth: String? = null,
public val paddingPolicy: String? = null,
public val resolution: String? = null,
public val sizingPolicy: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.elastictranscoder.outputs.PresetVideo): PresetVideo = PresetVideo(
aspectRatio = javaType.aspectRatio().map({ args0 -> args0 }).orElse(null),
bitRate = javaType.bitRate().map({ args0 -> args0 }).orElse(null),
codec = javaType.codec().map({ args0 -> args0 }).orElse(null),
displayAspectRatio = javaType.displayAspectRatio().map({ args0 -> args0 }).orElse(null),
fixedGop = javaType.fixedGop().map({ args0 -> args0 }).orElse(null),
frameRate = javaType.frameRate().map({ args0 -> args0 }).orElse(null),
keyframesMaxDist = javaType.keyframesMaxDist().map({ args0 -> args0 }).orElse(null),
maxFrameRate = javaType.maxFrameRate().map({ args0 -> args0 }).orElse(null),
maxHeight = javaType.maxHeight().map({ args0 -> args0 }).orElse(null),
maxWidth = javaType.maxWidth().map({ args0 -> args0 }).orElse(null),
paddingPolicy = javaType.paddingPolicy().map({ args0 -> args0 }).orElse(null),
resolution = javaType.resolution().map({ args0 -> args0 }).orElse(null),
sizingPolicy = javaType.sizingPolicy().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy