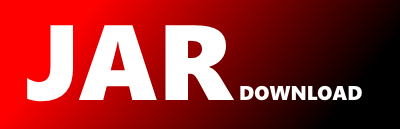
com.pulumi.aws.elb.kotlin.inputs.LoadBalancerHealthCheckArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.elb.kotlin.inputs
import com.pulumi.aws.elb.inputs.LoadBalancerHealthCheckArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property healthyThreshold The number of checks before the instance is declared healthy.
* @property interval The interval between checks.
* @property target The target of the check. Valid pattern is "${PROTOCOL}:${PORT}${PATH}", where PROTOCOL
* values are:
* * `HTTP`, `HTTPS` - PORT and PATH are required
* * `TCP`, `SSL` - PORT is required, PATH is not supported
* @property timeout The length of time before the check times out.
* @property unhealthyThreshold The number of checks before the instance is declared unhealthy.
*/
public data class LoadBalancerHealthCheckArgs(
public val healthyThreshold: Output,
public val interval: Output,
public val target: Output,
public val timeout: Output,
public val unhealthyThreshold: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.elb.inputs.LoadBalancerHealthCheckArgs =
com.pulumi.aws.elb.inputs.LoadBalancerHealthCheckArgs.builder()
.healthyThreshold(healthyThreshold.applyValue({ args0 -> args0 }))
.interval(interval.applyValue({ args0 -> args0 }))
.target(target.applyValue({ args0 -> args0 }))
.timeout(timeout.applyValue({ args0 -> args0 }))
.unhealthyThreshold(unhealthyThreshold.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LoadBalancerHealthCheckArgs].
*/
@PulumiTagMarker
public class LoadBalancerHealthCheckArgsBuilder internal constructor() {
private var healthyThreshold: Output? = null
private var interval: Output? = null
private var target: Output? = null
private var timeout: Output? = null
private var unhealthyThreshold: Output? = null
/**
* @param value The number of checks before the instance is declared healthy.
*/
@JvmName("iniokhqxpthnbpbs")
public suspend fun healthyThreshold(`value`: Output) {
this.healthyThreshold = value
}
/**
* @param value The interval between checks.
*/
@JvmName("cjanvisdnocspgjc")
public suspend fun interval(`value`: Output) {
this.interval = value
}
/**
* @param value The target of the check. Valid pattern is "${PROTOCOL}:${PORT}${PATH}", where PROTOCOL
* values are:
* * `HTTP`, `HTTPS` - PORT and PATH are required
* * `TCP`, `SSL` - PORT is required, PATH is not supported
*/
@JvmName("kpyyqoufdwdbsrlx")
public suspend fun target(`value`: Output) {
this.target = value
}
/**
* @param value The length of time before the check times out.
*/
@JvmName("citpnowseuywvcwg")
public suspend fun timeout(`value`: Output) {
this.timeout = value
}
/**
* @param value The number of checks before the instance is declared unhealthy.
*/
@JvmName("ieiiihkhkeixjinf")
public suspend fun unhealthyThreshold(`value`: Output) {
this.unhealthyThreshold = value
}
/**
* @param value The number of checks before the instance is declared healthy.
*/
@JvmName("bgkprowbaxpdsnlb")
public suspend fun healthyThreshold(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.healthyThreshold = mapped
}
/**
* @param value The interval between checks.
*/
@JvmName("xkubxfcdfbgnjxhv")
public suspend fun interval(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.interval = mapped
}
/**
* @param value The target of the check. Valid pattern is "${PROTOCOL}:${PORT}${PATH}", where PROTOCOL
* values are:
* * `HTTP`, `HTTPS` - PORT and PATH are required
* * `TCP`, `SSL` - PORT is required, PATH is not supported
*/
@JvmName("atowoobrhcdblyik")
public suspend fun target(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.target = mapped
}
/**
* @param value The length of time before the check times out.
*/
@JvmName("lbedlsnamhqthwou")
public suspend fun timeout(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.timeout = mapped
}
/**
* @param value The number of checks before the instance is declared unhealthy.
*/
@JvmName("vfkyyflrfpffhxmq")
public suspend fun unhealthyThreshold(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.unhealthyThreshold = mapped
}
internal fun build(): LoadBalancerHealthCheckArgs = LoadBalancerHealthCheckArgs(
healthyThreshold = healthyThreshold ?: throw PulumiNullFieldException("healthyThreshold"),
interval = interval ?: throw PulumiNullFieldException("interval"),
target = target ?: throw PulumiNullFieldException("target"),
timeout = timeout ?: throw PulumiNullFieldException("timeout"),
unhealthyThreshold = unhealthyThreshold ?: throw PulumiNullFieldException("unhealthyThreshold"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy