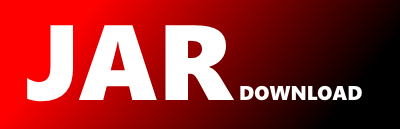
com.pulumi.aws.emr.kotlin.StudioSessionMappingArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.emr.kotlin
import com.pulumi.aws.emr.StudioSessionMappingArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Provides an Elastic MapReduce Studio Session Mapping.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.emr.StudioSessionMapping("example", {
* studioId: exampleAwsEmrStudio.id,
* identityType: "USER",
* identityId: "example",
* sessionPolicyArn: exampleAwsIamPolicy.arn,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.emr.StudioSessionMapping("example",
* studio_id=example_aws_emr_studio["id"],
* identity_type="USER",
* identity_id="example",
* session_policy_arn=example_aws_iam_policy["arn"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Emr.StudioSessionMapping("example", new()
* {
* StudioId = exampleAwsEmrStudio.Id,
* IdentityType = "USER",
* IdentityId = "example",
* SessionPolicyArn = exampleAwsIamPolicy.Arn,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/emr"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := emr.NewStudioSessionMapping(ctx, "example", &emr.StudioSessionMappingArgs{
* StudioId: pulumi.Any(exampleAwsEmrStudio.Id),
* IdentityType: pulumi.String("USER"),
* IdentityId: pulumi.String("example"),
* SessionPolicyArn: pulumi.Any(exampleAwsIamPolicy.Arn),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.emr.StudioSessionMapping;
* import com.pulumi.aws.emr.StudioSessionMappingArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new StudioSessionMapping("example", StudioSessionMappingArgs.builder()
* .studioId(exampleAwsEmrStudio.id())
* .identityType("USER")
* .identityId("example")
* .sessionPolicyArn(exampleAwsIamPolicy.arn())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:emr:StudioSessionMapping
* properties:
* studioId: ${exampleAwsEmrStudio.id}
* identityType: USER
* identityId: example
* sessionPolicyArn: ${exampleAwsIamPolicy.arn}
* ```
*
* ## Import
* Using `pulumi import`, import EMR studio session mappings using `studio-id:identity-type:identity-id`. For example:
* ```sh
* $ pulumi import aws:emr/studioSessionMapping:StudioSessionMapping example es-xxxxx:USER:xxxxx-xxx-xxx
* ```
* @property identityId The globally unique identifier (GUID) of the user or group from the Amazon Web Services SSO Identity Store.
* @property identityName The name of the user or group from the Amazon Web Services SSO Identity Store.
* @property identityType Specifies whether the identity to map to the Amazon EMR Studio is a `USER` or a `GROUP`.
* @property sessionPolicyArn The Amazon Resource Name (ARN) for the session policy that will be applied to the user or group. You should specify the ARN for the session policy that you want to apply, not the ARN of your user role.
* @property studioId The ID of the Amazon EMR Studio to which the user or group will be mapped.
*/
public data class StudioSessionMappingArgs(
public val identityId: Output? = null,
public val identityName: Output? = null,
public val identityType: Output? = null,
public val sessionPolicyArn: Output? = null,
public val studioId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.emr.StudioSessionMappingArgs =
com.pulumi.aws.emr.StudioSessionMappingArgs.builder()
.identityId(identityId?.applyValue({ args0 -> args0 }))
.identityName(identityName?.applyValue({ args0 -> args0 }))
.identityType(identityType?.applyValue({ args0 -> args0 }))
.sessionPolicyArn(sessionPolicyArn?.applyValue({ args0 -> args0 }))
.studioId(studioId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [StudioSessionMappingArgs].
*/
@PulumiTagMarker
public class StudioSessionMappingArgsBuilder internal constructor() {
private var identityId: Output? = null
private var identityName: Output? = null
private var identityType: Output? = null
private var sessionPolicyArn: Output? = null
private var studioId: Output? = null
/**
* @param value The globally unique identifier (GUID) of the user or group from the Amazon Web Services SSO Identity Store.
*/
@JvmName("mpielrooptovcsqf")
public suspend fun identityId(`value`: Output) {
this.identityId = value
}
/**
* @param value The name of the user or group from the Amazon Web Services SSO Identity Store.
*/
@JvmName("okbcgbhiegjsnhrd")
public suspend fun identityName(`value`: Output) {
this.identityName = value
}
/**
* @param value Specifies whether the identity to map to the Amazon EMR Studio is a `USER` or a `GROUP`.
*/
@JvmName("twpxbgdyhqkehqkn")
public suspend fun identityType(`value`: Output) {
this.identityType = value
}
/**
* @param value The Amazon Resource Name (ARN) for the session policy that will be applied to the user or group. You should specify the ARN for the session policy that you want to apply, not the ARN of your user role.
*/
@JvmName("vllsdhmvydsbsxvh")
public suspend fun sessionPolicyArn(`value`: Output) {
this.sessionPolicyArn = value
}
/**
* @param value The ID of the Amazon EMR Studio to which the user or group will be mapped.
*/
@JvmName("ofknlcasljljbhen")
public suspend fun studioId(`value`: Output) {
this.studioId = value
}
/**
* @param value The globally unique identifier (GUID) of the user or group from the Amazon Web Services SSO Identity Store.
*/
@JvmName("kexxfrtfnibgcsdg")
public suspend fun identityId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.identityId = mapped
}
/**
* @param value The name of the user or group from the Amazon Web Services SSO Identity Store.
*/
@JvmName("pwddeojefahbgwue")
public suspend fun identityName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.identityName = mapped
}
/**
* @param value Specifies whether the identity to map to the Amazon EMR Studio is a `USER` or a `GROUP`.
*/
@JvmName("eyangucaonohdchs")
public suspend fun identityType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.identityType = mapped
}
/**
* @param value The Amazon Resource Name (ARN) for the session policy that will be applied to the user or group. You should specify the ARN for the session policy that you want to apply, not the ARN of your user role.
*/
@JvmName("bqniyxjsyuvvqoeb")
public suspend fun sessionPolicyArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sessionPolicyArn = mapped
}
/**
* @param value The ID of the Amazon EMR Studio to which the user or group will be mapped.
*/
@JvmName("unliatwnsluhjbgy")
public suspend fun studioId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.studioId = mapped
}
internal fun build(): StudioSessionMappingArgs = StudioSessionMappingArgs(
identityId = identityId,
identityName = identityName,
identityType = identityType,
sessionPolicyArn = sessionPolicyArn,
studioId = studioId,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy