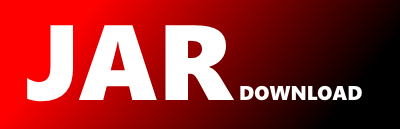
com.pulumi.aws.emr.kotlin.inputs.ClusterEc2AttributesArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.emr.kotlin.inputs
import com.pulumi.aws.emr.inputs.ClusterEc2AttributesArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property additionalMasterSecurityGroups String containing a comma separated list of additional Amazon EC2 security group IDs for the master node.
* @property additionalSlaveSecurityGroups String containing a comma separated list of additional Amazon EC2 security group IDs for the slave nodes as a comma separated string.
* @property emrManagedMasterSecurityGroup Identifier of the Amazon EC2 EMR-Managed security group for the master node.
* @property emrManagedSlaveSecurityGroup Identifier of the Amazon EC2 EMR-Managed security group for the slave nodes.
* @property instanceProfile Instance Profile for EC2 instances of the cluster assume this role.
* @property keyName Amazon EC2 key pair that can be used to ssh to the master node as the user called `hadoop`.
* @property serviceAccessSecurityGroup Identifier of the Amazon EC2 service-access security group - required when the cluster runs on a private subnet.
* @property subnetId VPC subnet id where you want the job flow to launch. Cannot specify the `cc1.4xlarge` instance type for nodes of a job flow launched in an Amazon VPC.
* @property subnetIds List of VPC subnet id-s where you want the job flow to launch. Amazon EMR identifies the best Availability Zone to launch instances according to your fleet specifications.
* > **NOTE on EMR-Managed security groups:** These security groups will have any missing inbound or outbound access rules added and maintained by AWS, to ensure proper communication between instances in a cluster. The EMR service will maintain these rules for groups provided in `emr_managed_master_security_group` and `emr_managed_slave_security_group`; attempts to remove the required rules may succeed, only for the EMR service to re-add them in a matter of minutes. This may cause this provider to fail to destroy an environment that contains an EMR cluster, because the EMR service does not revoke rules added on deletion, leaving a cyclic dependency between the security groups that prevents their deletion. To avoid this, use the `revoke_rules_on_delete` optional attribute for any Security Group used in `emr_managed_master_security_group` and `emr_managed_slave_security_group`. See [Amazon EMR-Managed Security Groups](http://docs.aws.amazon.com/emr/latest/ManagementGuide/emr-man-sec-groups.html) for more information about the EMR-managed security group rules.
*/
public data class ClusterEc2AttributesArgs(
public val additionalMasterSecurityGroups: Output? = null,
public val additionalSlaveSecurityGroups: Output? = null,
public val emrManagedMasterSecurityGroup: Output? = null,
public val emrManagedSlaveSecurityGroup: Output? = null,
public val instanceProfile: Output,
public val keyName: Output? = null,
public val serviceAccessSecurityGroup: Output? = null,
public val subnetId: Output? = null,
public val subnetIds: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.emr.inputs.ClusterEc2AttributesArgs =
com.pulumi.aws.emr.inputs.ClusterEc2AttributesArgs.builder()
.additionalMasterSecurityGroups(additionalMasterSecurityGroups?.applyValue({ args0 -> args0 }))
.additionalSlaveSecurityGroups(additionalSlaveSecurityGroups?.applyValue({ args0 -> args0 }))
.emrManagedMasterSecurityGroup(emrManagedMasterSecurityGroup?.applyValue({ args0 -> args0 }))
.emrManagedSlaveSecurityGroup(emrManagedSlaveSecurityGroup?.applyValue({ args0 -> args0 }))
.instanceProfile(instanceProfile.applyValue({ args0 -> args0 }))
.keyName(keyName?.applyValue({ args0 -> args0 }))
.serviceAccessSecurityGroup(serviceAccessSecurityGroup?.applyValue({ args0 -> args0 }))
.subnetId(subnetId?.applyValue({ args0 -> args0 }))
.subnetIds(subnetIds?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [ClusterEc2AttributesArgs].
*/
@PulumiTagMarker
public class ClusterEc2AttributesArgsBuilder internal constructor() {
private var additionalMasterSecurityGroups: Output? = null
private var additionalSlaveSecurityGroups: Output? = null
private var emrManagedMasterSecurityGroup: Output? = null
private var emrManagedSlaveSecurityGroup: Output? = null
private var instanceProfile: Output? = null
private var keyName: Output? = null
private var serviceAccessSecurityGroup: Output? = null
private var subnetId: Output? = null
private var subnetIds: Output>? = null
/**
* @param value String containing a comma separated list of additional Amazon EC2 security group IDs for the master node.
*/
@JvmName("uuotiisflgmclkgr")
public suspend fun additionalMasterSecurityGroups(`value`: Output) {
this.additionalMasterSecurityGroups = value
}
/**
* @param value String containing a comma separated list of additional Amazon EC2 security group IDs for the slave nodes as a comma separated string.
*/
@JvmName("uqtxcmewqvipywjv")
public suspend fun additionalSlaveSecurityGroups(`value`: Output) {
this.additionalSlaveSecurityGroups = value
}
/**
* @param value Identifier of the Amazon EC2 EMR-Managed security group for the master node.
*/
@JvmName("gqtqnyxbppslwcmf")
public suspend fun emrManagedMasterSecurityGroup(`value`: Output) {
this.emrManagedMasterSecurityGroup = value
}
/**
* @param value Identifier of the Amazon EC2 EMR-Managed security group for the slave nodes.
*/
@JvmName("cvrrtcpnpltiilde")
public suspend fun emrManagedSlaveSecurityGroup(`value`: Output) {
this.emrManagedSlaveSecurityGroup = value
}
/**
* @param value Instance Profile for EC2 instances of the cluster assume this role.
*/
@JvmName("gifwqctrfgtlmaho")
public suspend fun instanceProfile(`value`: Output) {
this.instanceProfile = value
}
/**
* @param value Amazon EC2 key pair that can be used to ssh to the master node as the user called `hadoop`.
*/
@JvmName("mrguqjyqnexpauna")
public suspend fun keyName(`value`: Output) {
this.keyName = value
}
/**
* @param value Identifier of the Amazon EC2 service-access security group - required when the cluster runs on a private subnet.
*/
@JvmName("hsjluamvdfyqbmwa")
public suspend fun serviceAccessSecurityGroup(`value`: Output) {
this.serviceAccessSecurityGroup = value
}
/**
* @param value VPC subnet id where you want the job flow to launch. Cannot specify the `cc1.4xlarge` instance type for nodes of a job flow launched in an Amazon VPC.
*/
@JvmName("eekpvfutfjjekayh")
public suspend fun subnetId(`value`: Output) {
this.subnetId = value
}
/**
* @param value List of VPC subnet id-s where you want the job flow to launch. Amazon EMR identifies the best Availability Zone to launch instances according to your fleet specifications.
* > **NOTE on EMR-Managed security groups:** These security groups will have any missing inbound or outbound access rules added and maintained by AWS, to ensure proper communication between instances in a cluster. The EMR service will maintain these rules for groups provided in `emr_managed_master_security_group` and `emr_managed_slave_security_group`; attempts to remove the required rules may succeed, only for the EMR service to re-add them in a matter of minutes. This may cause this provider to fail to destroy an environment that contains an EMR cluster, because the EMR service does not revoke rules added on deletion, leaving a cyclic dependency between the security groups that prevents their deletion. To avoid this, use the `revoke_rules_on_delete` optional attribute for any Security Group used in `emr_managed_master_security_group` and `emr_managed_slave_security_group`. See [Amazon EMR-Managed Security Groups](http://docs.aws.amazon.com/emr/latest/ManagementGuide/emr-man-sec-groups.html) for more information about the EMR-managed security group rules.
*/
@JvmName("ekdwcgndncfryuvo")
public suspend fun subnetIds(`value`: Output>) {
this.subnetIds = value
}
@JvmName("deulansvuhwgyhga")
public suspend fun subnetIds(vararg values: Output) {
this.subnetIds = Output.all(values.asList())
}
/**
* @param values List of VPC subnet id-s where you want the job flow to launch. Amazon EMR identifies the best Availability Zone to launch instances according to your fleet specifications.
* > **NOTE on EMR-Managed security groups:** These security groups will have any missing inbound or outbound access rules added and maintained by AWS, to ensure proper communication between instances in a cluster. The EMR service will maintain these rules for groups provided in `emr_managed_master_security_group` and `emr_managed_slave_security_group`; attempts to remove the required rules may succeed, only for the EMR service to re-add them in a matter of minutes. This may cause this provider to fail to destroy an environment that contains an EMR cluster, because the EMR service does not revoke rules added on deletion, leaving a cyclic dependency between the security groups that prevents their deletion. To avoid this, use the `revoke_rules_on_delete` optional attribute for any Security Group used in `emr_managed_master_security_group` and `emr_managed_slave_security_group`. See [Amazon EMR-Managed Security Groups](http://docs.aws.amazon.com/emr/latest/ManagementGuide/emr-man-sec-groups.html) for more information about the EMR-managed security group rules.
*/
@JvmName("ucmispojxalbvklo")
public suspend fun subnetIds(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy