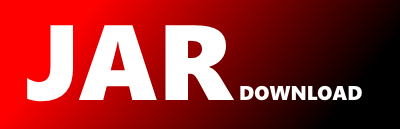
com.pulumi.aws.emr.kotlin.inputs.ClusterKerberosAttributesArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.emr.kotlin.inputs
import com.pulumi.aws.emr.inputs.ClusterKerberosAttributesArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property adDomainJoinPassword Active Directory password for `ad_domain_join_user`. This provider cannot perform drift detection of this configuration.
* @property adDomainJoinUser Required only when establishing a cross-realm trust with an Active Directory domain. A user with sufficient privileges to join resources to the domain. This provider cannot perform drift detection of this configuration.
* @property crossRealmTrustPrincipalPassword Required only when establishing a cross-realm trust with a KDC in a different realm. The cross-realm principal password, which must be identical across realms. This provider cannot perform drift detection of this configuration.
* @property kdcAdminPassword Password used within the cluster for the kadmin service on the cluster-dedicated KDC, which maintains Kerberos principals, password policies, and keytabs for the cluster. This provider cannot perform drift detection of this configuration.
* @property realm Name of the Kerberos realm to which all nodes in a cluster belong. For example, `EC2.INTERNAL`
*/
public data class ClusterKerberosAttributesArgs(
public val adDomainJoinPassword: Output? = null,
public val adDomainJoinUser: Output? = null,
public val crossRealmTrustPrincipalPassword: Output? = null,
public val kdcAdminPassword: Output,
public val realm: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.emr.inputs.ClusterKerberosAttributesArgs =
com.pulumi.aws.emr.inputs.ClusterKerberosAttributesArgs.builder()
.adDomainJoinPassword(adDomainJoinPassword?.applyValue({ args0 -> args0 }))
.adDomainJoinUser(adDomainJoinUser?.applyValue({ args0 -> args0 }))
.crossRealmTrustPrincipalPassword(crossRealmTrustPrincipalPassword?.applyValue({ args0 -> args0 }))
.kdcAdminPassword(kdcAdminPassword.applyValue({ args0 -> args0 }))
.realm(realm.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ClusterKerberosAttributesArgs].
*/
@PulumiTagMarker
public class ClusterKerberosAttributesArgsBuilder internal constructor() {
private var adDomainJoinPassword: Output? = null
private var adDomainJoinUser: Output? = null
private var crossRealmTrustPrincipalPassword: Output? = null
private var kdcAdminPassword: Output? = null
private var realm: Output? = null
/**
* @param value Active Directory password for `ad_domain_join_user`. This provider cannot perform drift detection of this configuration.
*/
@JvmName("vqkgsioocyempydj")
public suspend fun adDomainJoinPassword(`value`: Output) {
this.adDomainJoinPassword = value
}
/**
* @param value Required only when establishing a cross-realm trust with an Active Directory domain. A user with sufficient privileges to join resources to the domain. This provider cannot perform drift detection of this configuration.
*/
@JvmName("assspxoplweywgdf")
public suspend fun adDomainJoinUser(`value`: Output) {
this.adDomainJoinUser = value
}
/**
* @param value Required only when establishing a cross-realm trust with a KDC in a different realm. The cross-realm principal password, which must be identical across realms. This provider cannot perform drift detection of this configuration.
*/
@JvmName("kotsskomllpdgjua")
public suspend fun crossRealmTrustPrincipalPassword(`value`: Output) {
this.crossRealmTrustPrincipalPassword = value
}
/**
* @param value Password used within the cluster for the kadmin service on the cluster-dedicated KDC, which maintains Kerberos principals, password policies, and keytabs for the cluster. This provider cannot perform drift detection of this configuration.
*/
@JvmName("mouxxahtkllyqplj")
public suspend fun kdcAdminPassword(`value`: Output) {
this.kdcAdminPassword = value
}
/**
* @param value Name of the Kerberos realm to which all nodes in a cluster belong. For example, `EC2.INTERNAL`
*/
@JvmName("oweiiumydgbtvkyl")
public suspend fun realm(`value`: Output) {
this.realm = value
}
/**
* @param value Active Directory password for `ad_domain_join_user`. This provider cannot perform drift detection of this configuration.
*/
@JvmName("khnfrdynpeyiryyd")
public suspend fun adDomainJoinPassword(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.adDomainJoinPassword = mapped
}
/**
* @param value Required only when establishing a cross-realm trust with an Active Directory domain. A user with sufficient privileges to join resources to the domain. This provider cannot perform drift detection of this configuration.
*/
@JvmName("nijixqcaqooirvgw")
public suspend fun adDomainJoinUser(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.adDomainJoinUser = mapped
}
/**
* @param value Required only when establishing a cross-realm trust with a KDC in a different realm. The cross-realm principal password, which must be identical across realms. This provider cannot perform drift detection of this configuration.
*/
@JvmName("tcvipxxjirecriyg")
public suspend fun crossRealmTrustPrincipalPassword(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.crossRealmTrustPrincipalPassword = mapped
}
/**
* @param value Password used within the cluster for the kadmin service on the cluster-dedicated KDC, which maintains Kerberos principals, password policies, and keytabs for the cluster. This provider cannot perform drift detection of this configuration.
*/
@JvmName("qjdjbclgbtyiltpi")
public suspend fun kdcAdminPassword(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.kdcAdminPassword = mapped
}
/**
* @param value Name of the Kerberos realm to which all nodes in a cluster belong. For example, `EC2.INTERNAL`
*/
@JvmName("agslferldmoilurt")
public suspend fun realm(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.realm = mapped
}
internal fun build(): ClusterKerberosAttributesArgs = ClusterKerberosAttributesArgs(
adDomainJoinPassword = adDomainJoinPassword,
adDomainJoinUser = adDomainJoinUser,
crossRealmTrustPrincipalPassword = crossRealmTrustPrincipalPassword,
kdcAdminPassword = kdcAdminPassword ?: throw PulumiNullFieldException("kdcAdminPassword"),
realm = realm ?: throw PulumiNullFieldException("realm"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy