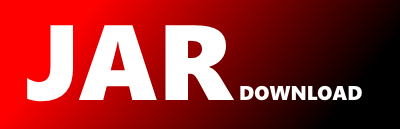
com.pulumi.aws.emr.kotlin.inputs.ManagedScalingPolicyComputeLimitArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.emr.kotlin.inputs
import com.pulumi.aws.emr.inputs.ManagedScalingPolicyComputeLimitArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property maximumCapacityUnits The upper boundary of EC2 units. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. Managed scaling activities are not allowed beyond this boundary. The limit only applies to the core and task nodes. The master node cannot be scaled after initial configuration.
* @property maximumCoreCapacityUnits The upper boundary of EC2 units for core node type in a cluster. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. The core units are not allowed to scale beyond this boundary. The parameter is used to split capacity allocation between core and task nodes.
* @property maximumOndemandCapacityUnits The upper boundary of On-Demand EC2 units. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. The On-Demand units are not allowed to scale beyond this boundary. The parameter is used to split capacity allocation between On-Demand and Spot instances.
* @property minimumCapacityUnits The lower boundary of EC2 units. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. Managed scaling activities are not allowed beyond this boundary. The limit only applies to the core and task nodes. The master node cannot be scaled after initial configuration.
* @property unitType The unit type used for specifying a managed scaling policy. Valid Values: `InstanceFleetUnits` | `Instances` | `VCPU`
*/
public data class ManagedScalingPolicyComputeLimitArgs(
public val maximumCapacityUnits: Output,
public val maximumCoreCapacityUnits: Output? = null,
public val maximumOndemandCapacityUnits: Output? = null,
public val minimumCapacityUnits: Output,
public val unitType: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.emr.inputs.ManagedScalingPolicyComputeLimitArgs =
com.pulumi.aws.emr.inputs.ManagedScalingPolicyComputeLimitArgs.builder()
.maximumCapacityUnits(maximumCapacityUnits.applyValue({ args0 -> args0 }))
.maximumCoreCapacityUnits(maximumCoreCapacityUnits?.applyValue({ args0 -> args0 }))
.maximumOndemandCapacityUnits(maximumOndemandCapacityUnits?.applyValue({ args0 -> args0 }))
.minimumCapacityUnits(minimumCapacityUnits.applyValue({ args0 -> args0 }))
.unitType(unitType.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ManagedScalingPolicyComputeLimitArgs].
*/
@PulumiTagMarker
public class ManagedScalingPolicyComputeLimitArgsBuilder internal constructor() {
private var maximumCapacityUnits: Output? = null
private var maximumCoreCapacityUnits: Output? = null
private var maximumOndemandCapacityUnits: Output? = null
private var minimumCapacityUnits: Output? = null
private var unitType: Output? = null
/**
* @param value The upper boundary of EC2 units. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. Managed scaling activities are not allowed beyond this boundary. The limit only applies to the core and task nodes. The master node cannot be scaled after initial configuration.
*/
@JvmName("wqxfmbvytcnibsgh")
public suspend fun maximumCapacityUnits(`value`: Output) {
this.maximumCapacityUnits = value
}
/**
* @param value The upper boundary of EC2 units for core node type in a cluster. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. The core units are not allowed to scale beyond this boundary. The parameter is used to split capacity allocation between core and task nodes.
*/
@JvmName("ysfhjhksxedlivom")
public suspend fun maximumCoreCapacityUnits(`value`: Output) {
this.maximumCoreCapacityUnits = value
}
/**
* @param value The upper boundary of On-Demand EC2 units. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. The On-Demand units are not allowed to scale beyond this boundary. The parameter is used to split capacity allocation between On-Demand and Spot instances.
*/
@JvmName("scfrdvowppfjkntd")
public suspend fun maximumOndemandCapacityUnits(`value`: Output) {
this.maximumOndemandCapacityUnits = value
}
/**
* @param value The lower boundary of EC2 units. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. Managed scaling activities are not allowed beyond this boundary. The limit only applies to the core and task nodes. The master node cannot be scaled after initial configuration.
*/
@JvmName("knjciagrjmedtjdj")
public suspend fun minimumCapacityUnits(`value`: Output) {
this.minimumCapacityUnits = value
}
/**
* @param value The unit type used for specifying a managed scaling policy. Valid Values: `InstanceFleetUnits` | `Instances` | `VCPU`
*/
@JvmName("bnhghvmfotcjrmbr")
public suspend fun unitType(`value`: Output) {
this.unitType = value
}
/**
* @param value The upper boundary of EC2 units. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. Managed scaling activities are not allowed beyond this boundary. The limit only applies to the core and task nodes. The master node cannot be scaled after initial configuration.
*/
@JvmName("jidalorcemruxvdo")
public suspend fun maximumCapacityUnits(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.maximumCapacityUnits = mapped
}
/**
* @param value The upper boundary of EC2 units for core node type in a cluster. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. The core units are not allowed to scale beyond this boundary. The parameter is used to split capacity allocation between core and task nodes.
*/
@JvmName("gpdagqxaplgtytfu")
public suspend fun maximumCoreCapacityUnits(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maximumCoreCapacityUnits = mapped
}
/**
* @param value The upper boundary of On-Demand EC2 units. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. The On-Demand units are not allowed to scale beyond this boundary. The parameter is used to split capacity allocation between On-Demand and Spot instances.
*/
@JvmName("nngkkawaklbuprhn")
public suspend fun maximumOndemandCapacityUnits(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maximumOndemandCapacityUnits = mapped
}
/**
* @param value The lower boundary of EC2 units. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. Managed scaling activities are not allowed beyond this boundary. The limit only applies to the core and task nodes. The master node cannot be scaled after initial configuration.
*/
@JvmName("rwxuofkcylltaoyg")
public suspend fun minimumCapacityUnits(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.minimumCapacityUnits = mapped
}
/**
* @param value The unit type used for specifying a managed scaling policy. Valid Values: `InstanceFleetUnits` | `Instances` | `VCPU`
*/
@JvmName("bbmwntsupskqgnau")
public suspend fun unitType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.unitType = mapped
}
internal fun build(): ManagedScalingPolicyComputeLimitArgs = ManagedScalingPolicyComputeLimitArgs(
maximumCapacityUnits = maximumCapacityUnits ?: throw
PulumiNullFieldException("maximumCapacityUnits"),
maximumCoreCapacityUnits = maximumCoreCapacityUnits,
maximumOndemandCapacityUnits = maximumOndemandCapacityUnits,
minimumCapacityUnits = minimumCapacityUnits ?: throw
PulumiNullFieldException("minimumCapacityUnits"),
unitType = unitType ?: throw PulumiNullFieldException("unitType"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy