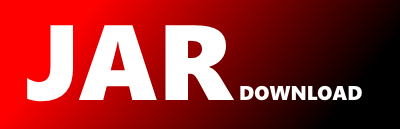
com.pulumi.aws.evidently.kotlin.inputs.LaunchScheduledSplitsConfigStepSegmentOverrideArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.evidently.kotlin.inputs
import com.pulumi.aws.evidently.inputs.LaunchScheduledSplitsConfigStepSegmentOverrideArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
*
* @property evaluationOrder Specifies a number indicating the order to use to evaluate segment overrides, if there are more than one. Segment overrides with lower numbers are evaluated first.
* @property segment The name or ARN of the segment to use.
* @property weights The traffic allocation percentages among the feature variations to assign to this segment. This is a set of key-value pairs. The keys are variation names. The values represent the amount of traffic to allocate to that variation for this segment. This is expressed in thousandths of a percent, so a weight of 50000 represents 50% of traffic.
*/
public data class LaunchScheduledSplitsConfigStepSegmentOverrideArgs(
public val evaluationOrder: Output,
public val segment: Output,
public val weights: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy