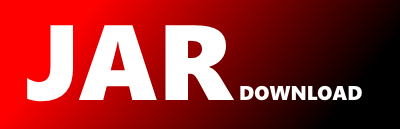
com.pulumi.aws.finspace.kotlin.KxDataview.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.finspace.kotlin
import com.pulumi.aws.finspace.kotlin.outputs.KxDataviewSegmentConfiguration
import com.pulumi.aws.finspace.kotlin.outputs.KxDataviewSegmentConfiguration.Companion.toKotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
/**
* Builder for [KxDataview].
*/
@PulumiTagMarker
public class KxDataviewResourceBuilder internal constructor() {
public var name: String? = null
public var args: KxDataviewArgs = KxDataviewArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend KxDataviewArgsBuilder.() -> Unit) {
val builder = KxDataviewArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): KxDataview {
val builtJavaResource = com.pulumi.aws.finspace.KxDataview(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return KxDataview(builtJavaResource)
}
}
/**
* Resource for managing an AWS FinSpace Kx Dataview.
* ## Example Usage
* ### Basic Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.finspace.KxDataview("example", {
* name: "my-tf-kx-dataview",
* environmentId: exampleAwsFinspaceKxEnvironment.id,
* databaseName: exampleAwsFinspaceKxDatabase.name,
* availabilityZoneId: "use1-az2",
* description: "Terraform managed Kx Dataview",
* azMode: "SINGLE",
* autoUpdate: true,
* segmentConfigurations: [{
* volumeName: exampleAwsFinspaceKxVolume.name,
* dbPaths: ["/*"],
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.finspace.KxDataview("example",
* name="my-tf-kx-dataview",
* environment_id=example_aws_finspace_kx_environment["id"],
* database_name=example_aws_finspace_kx_database["name"],
* availability_zone_id="use1-az2",
* description="Terraform managed Kx Dataview",
* az_mode="SINGLE",
* auto_update=True,
* segment_configurations=[{
* "volume_name": example_aws_finspace_kx_volume["name"],
* "db_paths": ["/*"],
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.FinSpace.KxDataview("example", new()
* {
* Name = "my-tf-kx-dataview",
* EnvironmentId = exampleAwsFinspaceKxEnvironment.Id,
* DatabaseName = exampleAwsFinspaceKxDatabase.Name,
* AvailabilityZoneId = "use1-az2",
* Description = "Terraform managed Kx Dataview",
* AzMode = "SINGLE",
* AutoUpdate = true,
* SegmentConfigurations = new[]
* {
* new Aws.FinSpace.Inputs.KxDataviewSegmentConfigurationArgs
* {
* VolumeName = exampleAwsFinspaceKxVolume.Name,
* DbPaths = new[]
* {
* "/*",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/finspace"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := finspace.NewKxDataview(ctx, "example", &finspace.KxDataviewArgs{
* Name: pulumi.String("my-tf-kx-dataview"),
* EnvironmentId: pulumi.Any(exampleAwsFinspaceKxEnvironment.Id),
* DatabaseName: pulumi.Any(exampleAwsFinspaceKxDatabase.Name),
* AvailabilityZoneId: pulumi.String("use1-az2"),
* Description: pulumi.String("Terraform managed Kx Dataview"),
* AzMode: pulumi.String("SINGLE"),
* AutoUpdate: pulumi.Bool(true),
* SegmentConfigurations: finspace.KxDataviewSegmentConfigurationArray{
* &finspace.KxDataviewSegmentConfigurationArgs{
* VolumeName: pulumi.Any(exampleAwsFinspaceKxVolume.Name),
* DbPaths: pulumi.StringArray{
* pulumi.String("/*"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.finspace.KxDataview;
* import com.pulumi.aws.finspace.KxDataviewArgs;
* import com.pulumi.aws.finspace.inputs.KxDataviewSegmentConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new KxDataview("example", KxDataviewArgs.builder()
* .name("my-tf-kx-dataview")
* .environmentId(exampleAwsFinspaceKxEnvironment.id())
* .databaseName(exampleAwsFinspaceKxDatabase.name())
* .availabilityZoneId("use1-az2")
* .description("Terraform managed Kx Dataview")
* .azMode("SINGLE")
* .autoUpdate(true)
* .segmentConfigurations(KxDataviewSegmentConfigurationArgs.builder()
* .volumeName(exampleAwsFinspaceKxVolume.name())
* .dbPaths("/*")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:finspace:KxDataview
* properties:
* name: my-tf-kx-dataview
* environmentId: ${exampleAwsFinspaceKxEnvironment.id}
* databaseName: ${exampleAwsFinspaceKxDatabase.name}
* availabilityZoneId: use1-az2
* description: Terraform managed Kx Dataview
* azMode: SINGLE
* autoUpdate: true
* segmentConfigurations:
* - volumeName: ${exampleAwsFinspaceKxVolume.name}
* dbPaths:
* - /*
* ```
*
* ## Import
* Using `pulumi import`, import an AWS FinSpace Kx Cluster using the `id` (environment ID and cluster name, comma-delimited). For example:
* ```sh
* $ pulumi import aws:finspace/kxDataview:KxDataview example n3ceo7wqxoxcti5tujqwzs,my-tf-kx-database,my-tf-kx-dataview
* ```
* */*/*/*/*/*/
*/
public class KxDataview internal constructor(
override val javaResource: com.pulumi.aws.finspace.KxDataview,
) : KotlinCustomResource(javaResource, KxDataviewMapper) {
/**
* Amazon Resource Name (ARN) identifier of the KX dataview.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* The option to specify whether you want to apply all the future additions and corrections automatically to the dataview, when you ingest new changesets. The default value is false.
*/
public val autoUpdate: Output
get() = javaResource.autoUpdate().applyValue({ args0 -> args0 })
/**
* The identifier of the availability zones. If attaching a volume, the volume must be in the same availability zone as the dataview that you are attaching to.
*/
public val availabilityZoneId: Output?
get() = javaResource.availabilityZoneId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The number of availability zones you want to assign per cluster. This can be one of the following:
* * `SINGLE` - Assigns one availability zone per cluster.
* * `MULTI` - Assigns all the availability zones per cluster.
*/
public val azMode: Output
get() = javaResource.azMode().applyValue({ args0 -> args0 })
/**
* A unique identifier of the changeset of the database that you want to use to ingest data.
*/
public val changesetId: Output?
get() = javaResource.changesetId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Timestamp at which the dataview was created in FinSpace. Value determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public val createdTimestamp: Output
get() = javaResource.createdTimestamp().applyValue({ args0 -> args0 })
/**
* The name of the database where you want to create a dataview.
*/
public val databaseName: Output
get() = javaResource.databaseName().applyValue({ args0 -> args0 })
/**
* A description for the dataview.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Unique identifier for the KX environment.
*/
public val environmentId: Output
get() = javaResource.environmentId().applyValue({ args0 -> args0 })
/**
* The last time that the dataview was updated in FinSpace. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public val lastModifiedTimestamp: Output
get() = javaResource.lastModifiedTimestamp().applyValue({ args0 -> args0 })
/**
* A unique identifier for the dataview.
* The following arguments are optional:
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The option to specify whether you want to make the dataview writable to perform database maintenance. The following are some considerations related to writable dataviews.
* * You cannot create partial writable dataviews. When you create writeable dataviews you must provide the entire database path. You cannot perform updates on a writeable dataview. Hence, `auto_update` must be set as `false` if `read_write` is `true` for a dataview.
* * You must also use a unique volume for creating a writeable dataview. So, if you choose a volume that is already in use by another dataview, the dataview creation fails.
* * Once you create a dataview as writeable, you cannot change it to read-only. So, you cannot update the `read_write` parameter later.
*/
public val readWrite: Output?
get() = javaResource.readWrite().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The configuration that contains the database path of the data that you want to place on each selected volume. Each segment must have a unique database path for each volume. If you do not explicitly specify any database path for a volume, they are accessible from the cluster through the default S3/object store segment. See segment_configurations below.
*/
public val segmentConfigurations: Output>?
get() = javaResource.segmentConfigurations().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0.let({ args0 -> toKotlin(args0) }) })
}).orElse(null)
})
public val status: Output
get() = javaResource.status().applyValue({ args0 -> args0 })
/**
* Key-value mapping of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy