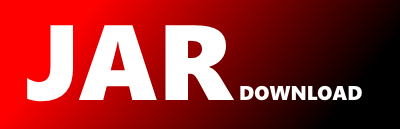
com.pulumi.aws.fms.kotlin.PolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.fms.kotlin
import com.pulumi.aws.fms.PolicyArgs.builder
import com.pulumi.aws.fms.kotlin.inputs.PolicyExcludeMapArgs
import com.pulumi.aws.fms.kotlin.inputs.PolicyExcludeMapArgsBuilder
import com.pulumi.aws.fms.kotlin.inputs.PolicyIncludeMapArgs
import com.pulumi.aws.fms.kotlin.inputs.PolicyIncludeMapArgsBuilder
import com.pulumi.aws.fms.kotlin.inputs.PolicySecurityServicePolicyDataArgs
import com.pulumi.aws.fms.kotlin.inputs.PolicySecurityServicePolicyDataArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Provides a resource to create an AWS Firewall Manager policy. You need to be using AWS organizations and have enabled the Firewall Manager administrator account.
* > **NOTE:** Due to limitations with testing, we provide it as best effort. If you find it useful, and have the ability to help test or notice issues, consider reaching out to us on GitHub.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const exampleRuleGroup = new aws.wafregional.RuleGroup("example", {
* metricName: "WAFRuleGroupExample",
* name: "WAF-Rule-Group-Example",
* });
* const example = new aws.fms.Policy("example", {
* name: "FMS-Policy-Example",
* excludeResourceTags: false,
* remediationEnabled: false,
* resourceType: "AWS::ElasticLoadBalancingV2::LoadBalancer",
* securityServicePolicyData: {
* type: "WAF",
* managedServiceData: pulumi.jsonStringify({
* type: "WAF",
* ruleGroups: [{
* id: exampleRuleGroup.id,
* overrideAction: {
* type: "COUNT",
* },
* }],
* defaultAction: {
* type: "BLOCK",
* },
* overrideCustomerWebACLAssociation: false,
* }),
* },
* tags: {
* Name: "example-fms-policy",
* },
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_aws as aws
* example_rule_group = aws.wafregional.RuleGroup("example",
* metric_name="WAFRuleGroupExample",
* name="WAF-Rule-Group-Example")
* example = aws.fms.Policy("example",
* name="FMS-Policy-Example",
* exclude_resource_tags=False,
* remediation_enabled=False,
* resource_type="AWS::ElasticLoadBalancingV2::LoadBalancer",
* security_service_policy_data={
* "type": "WAF",
* "managed_service_data": pulumi.Output.json_dumps({
* "type": "WAF",
* "ruleGroups": [{
* "id": example_rule_group.id,
* "overrideAction": {
* "type": "COUNT",
* },
* }],
* "defaultAction": {
* "type": "BLOCK",
* },
* "overrideCustomerWebACLAssociation": False,
* }),
* },
* tags={
* "Name": "example-fms-policy",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var exampleRuleGroup = new Aws.WafRegional.RuleGroup("example", new()
* {
* MetricName = "WAFRuleGroupExample",
* Name = "WAF-Rule-Group-Example",
* });
* var example = new Aws.Fms.Policy("example", new()
* {
* Name = "FMS-Policy-Example",
* ExcludeResourceTags = false,
* RemediationEnabled = false,
* ResourceType = "AWS::ElasticLoadBalancingV2::LoadBalancer",
* SecurityServicePolicyData = new Aws.Fms.Inputs.PolicySecurityServicePolicyDataArgs
* {
* Type = "WAF",
* ManagedServiceData = Output.JsonSerialize(Output.Create(new Dictionary
* {
* ["type"] = "WAF",
* ["ruleGroups"] = new[]
* {
* new Dictionary
* {
* ["id"] = exampleRuleGroup.Id,
* ["overrideAction"] = new Dictionary
* {
* ["type"] = "COUNT",
* },
* },
* },
* ["defaultAction"] = new Dictionary
* {
* ["type"] = "BLOCK",
* },
* ["overrideCustomerWebACLAssociation"] = false,
* })),
* },
* Tags =
* {
* { "Name", "example-fms-policy" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/fms"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/wafregional"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* exampleRuleGroup, err := wafregional.NewRuleGroup(ctx, "example", &wafregional.RuleGroupArgs{
* MetricName: pulumi.String("WAFRuleGroupExample"),
* Name: pulumi.String("WAF-Rule-Group-Example"),
* })
* if err != nil {
* return err
* }
* _, err = fms.NewPolicy(ctx, "example", &fms.PolicyArgs{
* Name: pulumi.String("FMS-Policy-Example"),
* ExcludeResourceTags: pulumi.Bool(false),
* RemediationEnabled: pulumi.Bool(false),
* ResourceType: pulumi.String("AWS::ElasticLoadBalancingV2::LoadBalancer"),
* SecurityServicePolicyData: &fms.PolicySecurityServicePolicyDataArgs{
* Type: pulumi.String("WAF"),
* ManagedServiceData: exampleRuleGroup.ID().ApplyT(func(id string) (pulumi.String, error) {
* var _zero pulumi.String
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "type": "WAF",
* "ruleGroups": []map[string]interface{}{
* map[string]interface{}{
* "id": id,
* "overrideAction": map[string]interface{}{
* "type": "COUNT",
* },
* },
* },
* "defaultAction": map[string]interface{}{
* "type": "BLOCK",
* },
* "overrideCustomerWebACLAssociation": false,
* })
* if err != nil {
* return _zero, err
* }
* json0 := string(tmpJSON0)
* return pulumi.String(json0), nil
* }).(pulumi.StringOutput),
* },
* Tags: pulumi.StringMap{
* "Name": pulumi.String("example-fms-policy"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.wafregional.RuleGroup;
* import com.pulumi.aws.wafregional.RuleGroupArgs;
* import com.pulumi.aws.fms.Policy;
* import com.pulumi.aws.fms.PolicyArgs;
* import com.pulumi.aws.fms.inputs.PolicySecurityServicePolicyDataArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var exampleRuleGroup = new RuleGroup("exampleRuleGroup", RuleGroupArgs.builder()
* .metricName("WAFRuleGroupExample")
* .name("WAF-Rule-Group-Example")
* .build());
* var example = new Policy("example", PolicyArgs.builder()
* .name("FMS-Policy-Example")
* .excludeResourceTags(false)
* .remediationEnabled(false)
* .resourceType("AWS::ElasticLoadBalancingV2::LoadBalancer")
* .securityServicePolicyData(PolicySecurityServicePolicyDataArgs.builder()
* .type("WAF")
* .managedServiceData(exampleRuleGroup.id().applyValue(id -> serializeJson(
* jsonObject(
* jsonProperty("type", "WAF"),
* jsonProperty("ruleGroups", jsonArray(jsonObject(
* jsonProperty("id", id),
* jsonProperty("overrideAction", jsonObject(
* jsonProperty("type", "COUNT")
* ))
* ))),
* jsonProperty("defaultAction", jsonObject(
* jsonProperty("type", "BLOCK")
* )),
* jsonProperty("overrideCustomerWebACLAssociation", false)
* ))))
* .build())
* .tags(Map.of("Name", "example-fms-policy"))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:fms:Policy
* properties:
* name: FMS-Policy-Example
* excludeResourceTags: false
* remediationEnabled: false
* resourceType: AWS::ElasticLoadBalancingV2::LoadBalancer
* securityServicePolicyData:
* type: WAF
* managedServiceData:
* fn::toJSON:
* type: WAF
* ruleGroups:
* - id: ${exampleRuleGroup.id}
* overrideAction:
* type: COUNT
* defaultAction:
* type: BLOCK
* overrideCustomerWebACLAssociation: false
* tags:
* Name: example-fms-policy
* exampleRuleGroup:
* type: aws:wafregional:RuleGroup
* name: example
* properties:
* metricName: WAFRuleGroupExample
* name: WAF-Rule-Group-Example
* ```
*
* ## Import
* Using `pulumi import`, import Firewall Manager policies using the policy ID. For example:
* ```sh
* $ pulumi import aws:fms/policy:Policy example 5be49585-a7e3-4c49-dde1-a179fe4a619a
* ```
* @property deleteAllPolicyResources If true, the request will also perform a clean-up process. Defaults to `true`. More information can be found here [AWS Firewall Manager delete policy](https://docs.aws.amazon.com/fms/2018-01-01/APIReference/API_DeletePolicy.html)
* @property deleteUnusedFmManagedResources If true, Firewall Manager will automatically remove protections from resources that leave the policy scope. Defaults to `false`. More information can be found here [AWS Firewall Manager policy contents](https://docs.aws.amazon.com/fms/2018-01-01/APIReference/API_Policy.html)
* @property description The description of the AWS Network Firewall firewall policy.
* @property excludeMap A map of lists of accounts and OU's to exclude from the policy.
* @property excludeResourceTags A boolean value, if true the tags that are specified in the `resource_tags` are not protected by this policy. If set to false and resource_tags are populated, resources that contain tags will be protected by this policy.
* @property includeMap A map of lists of accounts and OU's to include in the policy.
* @property name The friendly name of the AWS Firewall Manager Policy.
* @property remediationEnabled A boolean value, indicates if the policy should automatically applied to resources that already exist in the account.
* @property resourceSetIds
* @property resourceTags A map of resource tags, that if present will filter protections on resources based on the exclude_resource_tags.
* @property resourceType A resource type to protect. Conflicts with `resource_type_list`. See the [FMS API Reference](https://docs.aws.amazon.com/fms/2018-01-01/APIReference/API_Policy.html#fms-Type-Policy-ResourceType) for more information about supported values.
* @property resourceTypeLists A list of resource types to protect. Conflicts with `resource_type`. See the [FMS API Reference](https://docs.aws.amazon.com/fms/2018-01-01/APIReference/API_Policy.html#fms-Type-Policy-ResourceType) for more information about supported values. Lists with only one element are not supported, instead use `resource_type`.
* @property securityServicePolicyData The objects to include in Security Service Policy Data. Documented below.
* @property tags Key-value mapping of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level
*/
public data class PolicyArgs(
public val deleteAllPolicyResources: Output? = null,
public val deleteUnusedFmManagedResources: Output? = null,
public val description: Output? = null,
public val excludeMap: Output? = null,
public val excludeResourceTags: Output? = null,
public val includeMap: Output? = null,
public val name: Output? = null,
public val remediationEnabled: Output? = null,
public val resourceSetIds: Output>? = null,
public val resourceTags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy