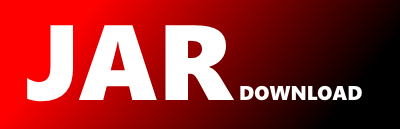
com.pulumi.aws.fms.kotlin.inputs.PolicyIncludeMapArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.fms.kotlin.inputs
import com.pulumi.aws.fms.inputs.PolicyIncludeMapArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property accounts A list of AWS Organization member Accounts that you want to include for this AWS FMS Policy.
* @property orgunits A list of IDs of the AWS Organizational Units that you want to include for this AWS FMS Policy. Specifying an OU is the equivalent of specifying all accounts in the OU and in any of its child OUs, including any child OUs and accounts that are added at a later time.
* You can specify inclusions or exclusions, but not both. If you specify an `include_map`, AWS Firewall Manager applies the policy to all accounts specified by the `include_map`, and does not evaluate any `exclude_map` specifications. If you do not specify an `include_map`, then Firewall Manager applies the policy to all accounts except for those specified by the `exclude_map`.
*/
public data class PolicyIncludeMapArgs(
public val accounts: Output>? = null,
public val orgunits: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.fms.inputs.PolicyIncludeMapArgs =
com.pulumi.aws.fms.inputs.PolicyIncludeMapArgs.builder()
.accounts(accounts?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.orgunits(orgunits?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [PolicyIncludeMapArgs].
*/
@PulumiTagMarker
public class PolicyIncludeMapArgsBuilder internal constructor() {
private var accounts: Output>? = null
private var orgunits: Output>? = null
/**
* @param value A list of AWS Organization member Accounts that you want to include for this AWS FMS Policy.
*/
@JvmName("uhhtrivinqswcbud")
public suspend fun accounts(`value`: Output>) {
this.accounts = value
}
@JvmName("uhibslpdwlyeywgp")
public suspend fun accounts(vararg values: Output) {
this.accounts = Output.all(values.asList())
}
/**
* @param values A list of AWS Organization member Accounts that you want to include for this AWS FMS Policy.
*/
@JvmName("pthplakpnlmkbbmi")
public suspend fun accounts(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy