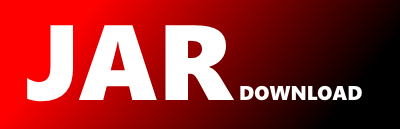
com.pulumi.aws.fsx.kotlin.WindowsFileSystem.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.fsx.kotlin
import com.pulumi.aws.fsx.kotlin.outputs.WindowsFileSystemAuditLogConfiguration
import com.pulumi.aws.fsx.kotlin.outputs.WindowsFileSystemDiskIopsConfiguration
import com.pulumi.aws.fsx.kotlin.outputs.WindowsFileSystemSelfManagedActiveDirectory
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.aws.fsx.kotlin.outputs.WindowsFileSystemAuditLogConfiguration.Companion.toKotlin as windowsFileSystemAuditLogConfigurationToKotlin
import com.pulumi.aws.fsx.kotlin.outputs.WindowsFileSystemDiskIopsConfiguration.Companion.toKotlin as windowsFileSystemDiskIopsConfigurationToKotlin
import com.pulumi.aws.fsx.kotlin.outputs.WindowsFileSystemSelfManagedActiveDirectory.Companion.toKotlin as windowsFileSystemSelfManagedActiveDirectoryToKotlin
/**
* Builder for [WindowsFileSystem].
*/
@PulumiTagMarker
public class WindowsFileSystemResourceBuilder internal constructor() {
public var name: String? = null
public var args: WindowsFileSystemArgs = WindowsFileSystemArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend WindowsFileSystemArgsBuilder.() -> Unit) {
val builder = WindowsFileSystemArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): WindowsFileSystem {
val builtJavaResource = com.pulumi.aws.fsx.WindowsFileSystem(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return WindowsFileSystem(builtJavaResource)
}
}
/**
* Manages a FSx Windows File System. See the [FSx Windows Guide](https://docs.aws.amazon.com/fsx/latest/WindowsGuide/what-is.html) for more information.
* > **NOTE:** Either the `active_directory_id` argument or `self_managed_active_directory` configuration block must be specified.
* ## Example Usage
* ### Using AWS Directory Service
* Additional information for using AWS Directory Service with Windows File Systems can be found in the [FSx Windows Guide](https://docs.aws.amazon.com/fsx/latest/WindowsGuide/fsx-aws-managed-ad.html).
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.fsx.WindowsFileSystem("example", {
* activeDirectoryId: exampleAwsDirectoryServiceDirectory.id,
* kmsKeyId: exampleAwsKmsKey.arn,
* storageCapacity: 300,
* subnetIds: [exampleAwsSubnet.id],
* throughputCapacity: 1024,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.fsx.WindowsFileSystem("example",
* active_directory_id=example_aws_directory_service_directory["id"],
* kms_key_id=example_aws_kms_key["arn"],
* storage_capacity=300,
* subnet_ids=[example_aws_subnet["id"]],
* throughput_capacity=1024)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Fsx.WindowsFileSystem("example", new()
* {
* ActiveDirectoryId = exampleAwsDirectoryServiceDirectory.Id,
* KmsKeyId = exampleAwsKmsKey.Arn,
* StorageCapacity = 300,
* SubnetIds = new[]
* {
* exampleAwsSubnet.Id,
* },
* ThroughputCapacity = 1024,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/fsx"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := fsx.NewWindowsFileSystem(ctx, "example", &fsx.WindowsFileSystemArgs{
* ActiveDirectoryId: pulumi.Any(exampleAwsDirectoryServiceDirectory.Id),
* KmsKeyId: pulumi.Any(exampleAwsKmsKey.Arn),
* StorageCapacity: pulumi.Int(300),
* SubnetIds: pulumi.StringArray{
* exampleAwsSubnet.Id,
* },
* ThroughputCapacity: pulumi.Int(1024),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.fsx.WindowsFileSystem;
* import com.pulumi.aws.fsx.WindowsFileSystemArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new WindowsFileSystem("example", WindowsFileSystemArgs.builder()
* .activeDirectoryId(exampleAwsDirectoryServiceDirectory.id())
* .kmsKeyId(exampleAwsKmsKey.arn())
* .storageCapacity(300)
* .subnetIds(exampleAwsSubnet.id())
* .throughputCapacity(1024)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:fsx:WindowsFileSystem
* properties:
* activeDirectoryId: ${exampleAwsDirectoryServiceDirectory.id}
* kmsKeyId: ${exampleAwsKmsKey.arn}
* storageCapacity: 300
* subnetIds:
* - ${exampleAwsSubnet.id}
* throughputCapacity: 1024
* ```
*
* ### Using a Self-Managed Microsoft Active Directory
* Additional information for using AWS Directory Service with Windows File Systems can be found in the [FSx Windows Guide](https://docs.aws.amazon.com/fsx/latest/WindowsGuide/self-managed-AD.html).
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.fsx.WindowsFileSystem("example", {
* kmsKeyId: exampleAwsKmsKey.arn,
* storageCapacity: 300,
* subnetIds: [exampleAwsSubnet.id],
* throughputCapacity: 1024,
* selfManagedActiveDirectory: {
* dnsIps: [
* "10.0.0.111",
* "10.0.0.222",
* ],
* domainName: "corp.example.com",
* password: "avoid-plaintext-passwords",
* username: "Admin",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.fsx.WindowsFileSystem("example",
* kms_key_id=example_aws_kms_key["arn"],
* storage_capacity=300,
* subnet_ids=[example_aws_subnet["id"]],
* throughput_capacity=1024,
* self_managed_active_directory={
* "dns_ips": [
* "10.0.0.111",
* "10.0.0.222",
* ],
* "domain_name": "corp.example.com",
* "password": "avoid-plaintext-passwords",
* "username": "Admin",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Fsx.WindowsFileSystem("example", new()
* {
* KmsKeyId = exampleAwsKmsKey.Arn,
* StorageCapacity = 300,
* SubnetIds = new[]
* {
* exampleAwsSubnet.Id,
* },
* ThroughputCapacity = 1024,
* SelfManagedActiveDirectory = new Aws.Fsx.Inputs.WindowsFileSystemSelfManagedActiveDirectoryArgs
* {
* DnsIps = new[]
* {
* "10.0.0.111",
* "10.0.0.222",
* },
* DomainName = "corp.example.com",
* Password = "avoid-plaintext-passwords",
* Username = "Admin",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/fsx"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := fsx.NewWindowsFileSystem(ctx, "example", &fsx.WindowsFileSystemArgs{
* KmsKeyId: pulumi.Any(exampleAwsKmsKey.Arn),
* StorageCapacity: pulumi.Int(300),
* SubnetIds: pulumi.StringArray{
* exampleAwsSubnet.Id,
* },
* ThroughputCapacity: pulumi.Int(1024),
* SelfManagedActiveDirectory: &fsx.WindowsFileSystemSelfManagedActiveDirectoryArgs{
* DnsIps: pulumi.StringArray{
* pulumi.String("10.0.0.111"),
* pulumi.String("10.0.0.222"),
* },
* DomainName: pulumi.String("corp.example.com"),
* Password: pulumi.String("avoid-plaintext-passwords"),
* Username: pulumi.String("Admin"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.fsx.WindowsFileSystem;
* import com.pulumi.aws.fsx.WindowsFileSystemArgs;
* import com.pulumi.aws.fsx.inputs.WindowsFileSystemSelfManagedActiveDirectoryArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new WindowsFileSystem("example", WindowsFileSystemArgs.builder()
* .kmsKeyId(exampleAwsKmsKey.arn())
* .storageCapacity(300)
* .subnetIds(exampleAwsSubnet.id())
* .throughputCapacity(1024)
* .selfManagedActiveDirectory(WindowsFileSystemSelfManagedActiveDirectoryArgs.builder()
* .dnsIps(
* "10.0.0.111",
* "10.0.0.222")
* .domainName("corp.example.com")
* .password("avoid-plaintext-passwords")
* .username("Admin")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:fsx:WindowsFileSystem
* properties:
* kmsKeyId: ${exampleAwsKmsKey.arn}
* storageCapacity: 300
* subnetIds:
* - ${exampleAwsSubnet.id}
* throughputCapacity: 1024
* selfManagedActiveDirectory:
* dnsIps:
* - 10.0.0.111
* - 10.0.0.222
* domainName: corp.example.com
* password: avoid-plaintext-passwords
* username: Admin
* ```
*
* ## Import
* Using `pulumi import`, import FSx File Systems using the `id`. For example:
* ```sh
* $ pulumi import aws:fsx/windowsFileSystem:WindowsFileSystem example fs-543ab12b1ca672f33
* ```
* Certain resource arguments, like `security_group_ids` and the `self_managed_active_directory` configuation block `password`, do not have a FSx API method for reading the information after creation. If these arguments are set in the Pulumi program on an imported resource, Pulumi will always show a difference. To workaround this behavior, either omit the argument from the Pulumi program or use `ignore_changes` to hide the difference. For example:
*/
public class WindowsFileSystem internal constructor(
override val javaResource: com.pulumi.aws.fsx.WindowsFileSystem,
) : KotlinCustomResource(javaResource, WindowsFileSystemMapper) {
/**
* The ID for an existing Microsoft Active Directory instance that the file system should join when it's created. Cannot be specified with `self_managed_active_directory`.
*/
public val activeDirectoryId: Output?
get() = javaResource.activeDirectoryId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* An array DNS alias names that you want to associate with the Amazon FSx file system. For more information, see [Working with DNS Aliases](https://docs.aws.amazon.com/fsx/latest/WindowsGuide/managing-dns-aliases.html)
*/
public val aliases: Output>?
get() = javaResource.aliases().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
/**
* Amazon Resource Name of the file system.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* The configuration that Amazon FSx for Windows File Server uses to audit and log user accesses of files, folders, and file shares on the Amazon FSx for Windows File Server file system. See `audit_log_configuration` Block for details.
*/
public val auditLogConfiguration: Output
get() = javaResource.auditLogConfiguration().applyValue({ args0 ->
args0.let({ args0 ->
windowsFileSystemAuditLogConfigurationToKotlin(args0)
})
})
/**
* The number of days to retain automatic backups. Minimum of `0` and maximum of `90`. Defaults to `7`. Set to `0` to disable.
*/
public val automaticBackupRetentionDays: Output?
get() = javaResource.automaticBackupRetentionDays().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The ID of the source backup to create the filesystem from.
*/
public val backupId: Output?
get() = javaResource.backupId().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* A boolean flag indicating whether tags on the file system should be copied to backups. Defaults to `false`.
*/
public val copyTagsToBackups: Output?
get() = javaResource.copyTagsToBackups().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The preferred time (in `HH:MM` format) to take daily automatic backups, in the UTC time zone.
*/
public val dailyAutomaticBackupStartTime: Output
get() = javaResource.dailyAutomaticBackupStartTime().applyValue({ args0 -> args0 })
/**
* Specifies the file system deployment type, valid values are `MULTI_AZ_1`, `SINGLE_AZ_1` and `SINGLE_AZ_2`. Default value is `SINGLE_AZ_1`.
*/
public val deploymentType: Output?
get() = javaResource.deploymentType().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The SSD IOPS configuration for the Amazon FSx for Windows File Server file system. See `disk_iops_configuration` Block for details.
*/
public val diskIopsConfiguration: Output
get() = javaResource.diskIopsConfiguration().applyValue({ args0 ->
args0.let({ args0 ->
windowsFileSystemDiskIopsConfigurationToKotlin(args0)
})
})
/**
* DNS name for the file system, e.g., `fs-12345678.corp.example.com` (domain name matching the Active Directory domain name)
*/
public val dnsName: Output
get() = javaResource.dnsName().applyValue({ args0 -> args0 })
/**
* A map of tags to apply to the file system's final backup.
*/
public val finalBackupTags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy