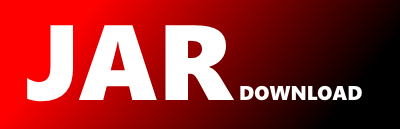
com.pulumi.aws.fsx.kotlin.inputs.OntapVolumeSnaplockConfigurationRetentionPeriodArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.fsx.kotlin.inputs
import com.pulumi.aws.fsx.inputs.OntapVolumeSnaplockConfigurationRetentionPeriodArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property defaultRetention The retention period assigned to a write once, read many (WORM) file by default if an explicit retention period is not set for an FSx for ONTAP SnapLock volume. The default retention period must be greater than or equal to the minimum retention period and less than or equal to the maximum retention period. See `default_retention` Block for details.
* @property maximumRetention The longest retention period that can be assigned to a WORM file on an FSx for ONTAP SnapLock volume. See `maximum_retention` Block for details.
* @property minimumRetention The shortest retention period that can be assigned to a WORM file on an FSx for ONTAP SnapLock volume. See `minimum_retention` Block for details.
*/
public data class OntapVolumeSnaplockConfigurationRetentionPeriodArgs(
public val defaultRetention: Output? = null,
public val maximumRetention: Output? = null,
public val minimumRetention: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.fsx.inputs.OntapVolumeSnaplockConfigurationRetentionPeriodArgs =
com.pulumi.aws.fsx.inputs.OntapVolumeSnaplockConfigurationRetentionPeriodArgs.builder()
.defaultRetention(defaultRetention?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.maximumRetention(maximumRetention?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.minimumRetention(
minimumRetention?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [OntapVolumeSnaplockConfigurationRetentionPeriodArgs].
*/
@PulumiTagMarker
public class OntapVolumeSnaplockConfigurationRetentionPeriodArgsBuilder internal constructor() {
private var defaultRetention:
Output? = null
private var maximumRetention:
Output? = null
private var minimumRetention:
Output? = null
/**
* @param value The retention period assigned to a write once, read many (WORM) file by default if an explicit retention period is not set for an FSx for ONTAP SnapLock volume. The default retention period must be greater than or equal to the minimum retention period and less than or equal to the maximum retention period. See `default_retention` Block for details.
*/
@JvmName("xhihmtxpjenxrhqq")
public suspend fun defaultRetention(`value`: Output) {
this.defaultRetention = value
}
/**
* @param value The longest retention period that can be assigned to a WORM file on an FSx for ONTAP SnapLock volume. See `maximum_retention` Block for details.
*/
@JvmName("utqfbpjktekhlnvv")
public suspend fun maximumRetention(`value`: Output) {
this.maximumRetention = value
}
/**
* @param value The shortest retention period that can be assigned to a WORM file on an FSx for ONTAP SnapLock volume. See `minimum_retention` Block for details.
*/
@JvmName("vqbndhwgcxqjfxfw")
public suspend fun minimumRetention(`value`: Output) {
this.minimumRetention = value
}
/**
* @param value The retention period assigned to a write once, read many (WORM) file by default if an explicit retention period is not set for an FSx for ONTAP SnapLock volume. The default retention period must be greater than or equal to the minimum retention period and less than or equal to the maximum retention period. See `default_retention` Block for details.
*/
@JvmName("jpviqlycguitrejl")
public suspend fun defaultRetention(`value`: OntapVolumeSnaplockConfigurationRetentionPeriodDefaultRetentionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultRetention = mapped
}
/**
* @param argument The retention period assigned to a write once, read many (WORM) file by default if an explicit retention period is not set for an FSx for ONTAP SnapLock volume. The default retention period must be greater than or equal to the minimum retention period and less than or equal to the maximum retention period. See `default_retention` Block for details.
*/
@JvmName("uhkkpjbmimvawxba")
public suspend fun defaultRetention(argument: suspend OntapVolumeSnaplockConfigurationRetentionPeriodDefaultRetentionArgsBuilder.() -> Unit) {
val toBeMapped =
OntapVolumeSnaplockConfigurationRetentionPeriodDefaultRetentionArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.defaultRetention = mapped
}
/**
* @param value The longest retention period that can be assigned to a WORM file on an FSx for ONTAP SnapLock volume. See `maximum_retention` Block for details.
*/
@JvmName("dimatadwhunebriq")
public suspend fun maximumRetention(`value`: OntapVolumeSnaplockConfigurationRetentionPeriodMaximumRetentionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maximumRetention = mapped
}
/**
* @param argument The longest retention period that can be assigned to a WORM file on an FSx for ONTAP SnapLock volume. See `maximum_retention` Block for details.
*/
@JvmName("bboqtlgwamteshmy")
public suspend fun maximumRetention(argument: suspend OntapVolumeSnaplockConfigurationRetentionPeriodMaximumRetentionArgsBuilder.() -> Unit) {
val toBeMapped =
OntapVolumeSnaplockConfigurationRetentionPeriodMaximumRetentionArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.maximumRetention = mapped
}
/**
* @param value The shortest retention period that can be assigned to a WORM file on an FSx for ONTAP SnapLock volume. See `minimum_retention` Block for details.
*/
@JvmName("dfyxhahsoojhxnca")
public suspend fun minimumRetention(`value`: OntapVolumeSnaplockConfigurationRetentionPeriodMinimumRetentionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minimumRetention = mapped
}
/**
* @param argument The shortest retention period that can be assigned to a WORM file on an FSx for ONTAP SnapLock volume. See `minimum_retention` Block for details.
*/
@JvmName("rqhfifmpikbknpdn")
public suspend fun minimumRetention(argument: suspend OntapVolumeSnaplockConfigurationRetentionPeriodMinimumRetentionArgsBuilder.() -> Unit) {
val toBeMapped =
OntapVolumeSnaplockConfigurationRetentionPeriodMinimumRetentionArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.minimumRetention = mapped
}
internal fun build(): OntapVolumeSnaplockConfigurationRetentionPeriodArgs =
OntapVolumeSnaplockConfigurationRetentionPeriodArgs(
defaultRetention = defaultRetention,
maximumRetention = maximumRetention,
minimumRetention = minimumRetention,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy