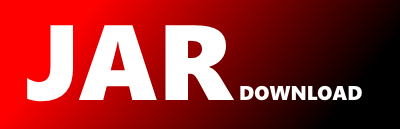
com.pulumi.aws.globalaccelerator.kotlin.EndpointGroupArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.globalaccelerator.kotlin
import com.pulumi.aws.globalaccelerator.EndpointGroupArgs.builder
import com.pulumi.aws.globalaccelerator.kotlin.inputs.EndpointGroupEndpointConfigurationArgs
import com.pulumi.aws.globalaccelerator.kotlin.inputs.EndpointGroupEndpointConfigurationArgsBuilder
import com.pulumi.aws.globalaccelerator.kotlin.inputs.EndpointGroupPortOverrideArgs
import com.pulumi.aws.globalaccelerator.kotlin.inputs.EndpointGroupPortOverrideArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Double
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Provides a Global Accelerator endpoint group.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.globalaccelerator.EndpointGroup("example", {
* listenerArn: exampleAwsGlobalacceleratorListener.id,
* endpointConfigurations: [{
* endpointId: exampleAwsLb.arn,
* weight: 100,
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.globalaccelerator.EndpointGroup("example",
* listener_arn=example_aws_globalaccelerator_listener["id"],
* endpoint_configurations=[{
* "endpoint_id": example_aws_lb["arn"],
* "weight": 100,
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.GlobalAccelerator.EndpointGroup("example", new()
* {
* ListenerArn = exampleAwsGlobalacceleratorListener.Id,
* EndpointConfigurations = new[]
* {
* new Aws.GlobalAccelerator.Inputs.EndpointGroupEndpointConfigurationArgs
* {
* EndpointId = exampleAwsLb.Arn,
* Weight = 100,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/globalaccelerator"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := globalaccelerator.NewEndpointGroup(ctx, "example", &globalaccelerator.EndpointGroupArgs{
* ListenerArn: pulumi.Any(exampleAwsGlobalacceleratorListener.Id),
* EndpointConfigurations: globalaccelerator.EndpointGroupEndpointConfigurationArray{
* &globalaccelerator.EndpointGroupEndpointConfigurationArgs{
* EndpointId: pulumi.Any(exampleAwsLb.Arn),
* Weight: pulumi.Int(100),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.globalaccelerator.EndpointGroup;
* import com.pulumi.aws.globalaccelerator.EndpointGroupArgs;
* import com.pulumi.aws.globalaccelerator.inputs.EndpointGroupEndpointConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new EndpointGroup("example", EndpointGroupArgs.builder()
* .listenerArn(exampleAwsGlobalacceleratorListener.id())
* .endpointConfigurations(EndpointGroupEndpointConfigurationArgs.builder()
* .endpointId(exampleAwsLb.arn())
* .weight(100)
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:globalaccelerator:EndpointGroup
* properties:
* listenerArn: ${exampleAwsGlobalacceleratorListener.id}
* endpointConfigurations:
* - endpointId: ${exampleAwsLb.arn}
* weight: 100
* ```
*
* ## Import
* Using `pulumi import`, import Global Accelerator endpoint groups using the `id`. For example:
* ```sh
* $ pulumi import aws:globalaccelerator/endpointGroup:EndpointGroup example arn:aws:globalaccelerator::111111111111:accelerator/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx/listener/xxxxxxx/endpoint-group/xxxxxxxx
* ```
* @property endpointConfigurations The list of endpoint objects. Fields documented below.
* @property endpointGroupRegion The name of the AWS Region where the endpoint group is located.
* @property healthCheckIntervalSeconds The time—10 seconds or 30 seconds—between each health check for an endpoint. The default value is 30.
* @property healthCheckPath If the protocol is HTTP/S, then this specifies the path that is the destination for health check targets. The default value is slash (`/`). the provider will only perform drift detection of its value when present in a configuration.
* @property healthCheckPort The port that AWS Global Accelerator uses to check the health of endpoints that are part of this endpoint group. The default port is the listener port that this endpoint group is associated with. If listener port is a list of ports, Global Accelerator uses the first port in the list.
* the provider will only perform drift detection of its value when present in a configuration.
* @property healthCheckProtocol The protocol that AWS Global Accelerator uses to check the health of endpoints that are part of this endpoint group. The default value is TCP.
* @property listenerArn The Amazon Resource Name (ARN) of the listener.
* @property portOverrides Override specific listener ports used to route traffic to endpoints that are part of this endpoint group. Fields documented below.
* @property thresholdCount The number of consecutive health checks required to set the state of a healthy endpoint to unhealthy, or to set an unhealthy endpoint to healthy. The default value is 3.
* @property trafficDialPercentage The percentage of traffic to send to an AWS Region. Additional traffic is distributed to other endpoint groups for this listener. The default value is 100.
*/
public data class EndpointGroupArgs(
public val endpointConfigurations: Output>? = null,
public val endpointGroupRegion: Output? = null,
public val healthCheckIntervalSeconds: Output? = null,
public val healthCheckPath: Output? = null,
public val healthCheckPort: Output? = null,
public val healthCheckProtocol: Output? = null,
public val listenerArn: Output? = null,
public val portOverrides: Output>? = null,
public val thresholdCount: Output? = null,
public val trafficDialPercentage: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.globalaccelerator.EndpointGroupArgs =
com.pulumi.aws.globalaccelerator.EndpointGroupArgs.builder()
.endpointConfigurations(
endpointConfigurations?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.endpointGroupRegion(endpointGroupRegion?.applyValue({ args0 -> args0 }))
.healthCheckIntervalSeconds(healthCheckIntervalSeconds?.applyValue({ args0 -> args0 }))
.healthCheckPath(healthCheckPath?.applyValue({ args0 -> args0 }))
.healthCheckPort(healthCheckPort?.applyValue({ args0 -> args0 }))
.healthCheckProtocol(healthCheckProtocol?.applyValue({ args0 -> args0 }))
.listenerArn(listenerArn?.applyValue({ args0 -> args0 }))
.portOverrides(
portOverrides?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.thresholdCount(thresholdCount?.applyValue({ args0 -> args0 }))
.trafficDialPercentage(trafficDialPercentage?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [EndpointGroupArgs].
*/
@PulumiTagMarker
public class EndpointGroupArgsBuilder internal constructor() {
private var endpointConfigurations: Output>? = null
private var endpointGroupRegion: Output? = null
private var healthCheckIntervalSeconds: Output? = null
private var healthCheckPath: Output? = null
private var healthCheckPort: Output? = null
private var healthCheckProtocol: Output? = null
private var listenerArn: Output? = null
private var portOverrides: Output>? = null
private var thresholdCount: Output? = null
private var trafficDialPercentage: Output? = null
/**
* @param value The list of endpoint objects. Fields documented below.
*/
@JvmName("qrviwbesquanokvt")
public suspend fun endpointConfigurations(`value`: Output>) {
this.endpointConfigurations = value
}
@JvmName("joclasbtkppkliqy")
public suspend fun endpointConfigurations(vararg values: Output) {
this.endpointConfigurations = Output.all(values.asList())
}
/**
* @param values The list of endpoint objects. Fields documented below.
*/
@JvmName("vhpnlbchavaqabmj")
public suspend fun endpointConfigurations(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy