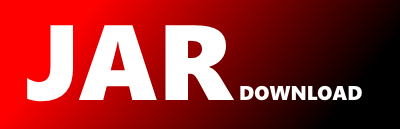
com.pulumi.aws.glue.kotlin.CrawlerArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.glue.kotlin
import com.pulumi.aws.glue.CrawlerArgs.builder
import com.pulumi.aws.glue.kotlin.inputs.CrawlerCatalogTargetArgs
import com.pulumi.aws.glue.kotlin.inputs.CrawlerCatalogTargetArgsBuilder
import com.pulumi.aws.glue.kotlin.inputs.CrawlerDeltaTargetArgs
import com.pulumi.aws.glue.kotlin.inputs.CrawlerDeltaTargetArgsBuilder
import com.pulumi.aws.glue.kotlin.inputs.CrawlerDynamodbTargetArgs
import com.pulumi.aws.glue.kotlin.inputs.CrawlerDynamodbTargetArgsBuilder
import com.pulumi.aws.glue.kotlin.inputs.CrawlerHudiTargetArgs
import com.pulumi.aws.glue.kotlin.inputs.CrawlerHudiTargetArgsBuilder
import com.pulumi.aws.glue.kotlin.inputs.CrawlerIcebergTargetArgs
import com.pulumi.aws.glue.kotlin.inputs.CrawlerIcebergTargetArgsBuilder
import com.pulumi.aws.glue.kotlin.inputs.CrawlerJdbcTargetArgs
import com.pulumi.aws.glue.kotlin.inputs.CrawlerJdbcTargetArgsBuilder
import com.pulumi.aws.glue.kotlin.inputs.CrawlerLakeFormationConfigurationArgs
import com.pulumi.aws.glue.kotlin.inputs.CrawlerLakeFormationConfigurationArgsBuilder
import com.pulumi.aws.glue.kotlin.inputs.CrawlerLineageConfigurationArgs
import com.pulumi.aws.glue.kotlin.inputs.CrawlerLineageConfigurationArgsBuilder
import com.pulumi.aws.glue.kotlin.inputs.CrawlerMongodbTargetArgs
import com.pulumi.aws.glue.kotlin.inputs.CrawlerMongodbTargetArgsBuilder
import com.pulumi.aws.glue.kotlin.inputs.CrawlerRecrawlPolicyArgs
import com.pulumi.aws.glue.kotlin.inputs.CrawlerRecrawlPolicyArgsBuilder
import com.pulumi.aws.glue.kotlin.inputs.CrawlerS3TargetArgs
import com.pulumi.aws.glue.kotlin.inputs.CrawlerS3TargetArgsBuilder
import com.pulumi.aws.glue.kotlin.inputs.CrawlerSchemaChangePolicyArgs
import com.pulumi.aws.glue.kotlin.inputs.CrawlerSchemaChangePolicyArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Manages a Glue Crawler. More information can be found in the [AWS Glue Developer Guide](https://docs.aws.amazon.com/glue/latest/dg/add-crawler.html)
* ## Example Usage
* ### DynamoDB Target Example
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.glue.Crawler("example", {
* databaseName: exampleAwsGlueCatalogDatabase.name,
* name: "example",
* role: exampleAwsIamRole.arn,
* dynamodbTargets: [{
* path: "table-name",
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.glue.Crawler("example",
* database_name=example_aws_glue_catalog_database["name"],
* name="example",
* role=example_aws_iam_role["arn"],
* dynamodb_targets=[{
* "path": "table-name",
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Glue.Crawler("example", new()
* {
* DatabaseName = exampleAwsGlueCatalogDatabase.Name,
* Name = "example",
* Role = exampleAwsIamRole.Arn,
* DynamodbTargets = new[]
* {
* new Aws.Glue.Inputs.CrawlerDynamodbTargetArgs
* {
* Path = "table-name",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/glue"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := glue.NewCrawler(ctx, "example", &glue.CrawlerArgs{
* DatabaseName: pulumi.Any(exampleAwsGlueCatalogDatabase.Name),
* Name: pulumi.String("example"),
* Role: pulumi.Any(exampleAwsIamRole.Arn),
* DynamodbTargets: glue.CrawlerDynamodbTargetArray{
* &glue.CrawlerDynamodbTargetArgs{
* Path: pulumi.String("table-name"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glue.Crawler;
* import com.pulumi.aws.glue.CrawlerArgs;
* import com.pulumi.aws.glue.inputs.CrawlerDynamodbTargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Crawler("example", CrawlerArgs.builder()
* .databaseName(exampleAwsGlueCatalogDatabase.name())
* .name("example")
* .role(exampleAwsIamRole.arn())
* .dynamodbTargets(CrawlerDynamodbTargetArgs.builder()
* .path("table-name")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:glue:Crawler
* properties:
* databaseName: ${exampleAwsGlueCatalogDatabase.name}
* name: example
* role: ${exampleAwsIamRole.arn}
* dynamodbTargets:
* - path: table-name
* ```
*
* ### JDBC Target Example
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.glue.Crawler("example", {
* databaseName: exampleAwsGlueCatalogDatabase.name,
* name: "example",
* role: exampleAwsIamRole.arn,
* jdbcTargets: [{
* connectionName: exampleAwsGlueConnection.name,
* path: "database-name/%",
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.glue.Crawler("example",
* database_name=example_aws_glue_catalog_database["name"],
* name="example",
* role=example_aws_iam_role["arn"],
* jdbc_targets=[{
* "connection_name": example_aws_glue_connection["name"],
* "path": "database-name/%",
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Glue.Crawler("example", new()
* {
* DatabaseName = exampleAwsGlueCatalogDatabase.Name,
* Name = "example",
* Role = exampleAwsIamRole.Arn,
* JdbcTargets = new[]
* {
* new Aws.Glue.Inputs.CrawlerJdbcTargetArgs
* {
* ConnectionName = exampleAwsGlueConnection.Name,
* Path = "database-name/%",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/glue"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := glue.NewCrawler(ctx, "example", &glue.CrawlerArgs{
* DatabaseName: pulumi.Any(exampleAwsGlueCatalogDatabase.Name),
* Name: pulumi.String("example"),
* Role: pulumi.Any(exampleAwsIamRole.Arn),
* JdbcTargets: glue.CrawlerJdbcTargetArray{
* &glue.CrawlerJdbcTargetArgs{
* ConnectionName: pulumi.Any(exampleAwsGlueConnection.Name),
* Path: pulumi.String("database-name/%"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glue.Crawler;
* import com.pulumi.aws.glue.CrawlerArgs;
* import com.pulumi.aws.glue.inputs.CrawlerJdbcTargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Crawler("example", CrawlerArgs.builder()
* .databaseName(exampleAwsGlueCatalogDatabase.name())
* .name("example")
* .role(exampleAwsIamRole.arn())
* .jdbcTargets(CrawlerJdbcTargetArgs.builder()
* .connectionName(exampleAwsGlueConnection.name())
* .path("database-name/%")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:glue:Crawler
* properties:
* databaseName: ${exampleAwsGlueCatalogDatabase.name}
* name: example
* role: ${exampleAwsIamRole.arn}
* jdbcTargets:
* - connectionName: ${exampleAwsGlueConnection.name}
* path: database-name/%
* ```
*
* ### S3 Target Example
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.glue.Crawler("example", {
* databaseName: exampleAwsGlueCatalogDatabase.name,
* name: "example",
* role: exampleAwsIamRole.arn,
* s3Targets: [{
* path: `s3://${exampleAwsS3Bucket.bucket}`,
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.glue.Crawler("example",
* database_name=example_aws_glue_catalog_database["name"],
* name="example",
* role=example_aws_iam_role["arn"],
* s3_targets=[{
* "path": f"s3://{example_aws_s3_bucket['bucket']}",
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Glue.Crawler("example", new()
* {
* DatabaseName = exampleAwsGlueCatalogDatabase.Name,
* Name = "example",
* Role = exampleAwsIamRole.Arn,
* S3Targets = new[]
* {
* new Aws.Glue.Inputs.CrawlerS3TargetArgs
* {
* Path = $"s3://{exampleAwsS3Bucket.Bucket}",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/glue"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := glue.NewCrawler(ctx, "example", &glue.CrawlerArgs{
* DatabaseName: pulumi.Any(exampleAwsGlueCatalogDatabase.Name),
* Name: pulumi.String("example"),
* Role: pulumi.Any(exampleAwsIamRole.Arn),
* S3Targets: glue.CrawlerS3TargetArray{
* &glue.CrawlerS3TargetArgs{
* Path: pulumi.Sprintf("s3://%v", exampleAwsS3Bucket.Bucket),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glue.Crawler;
* import com.pulumi.aws.glue.CrawlerArgs;
* import com.pulumi.aws.glue.inputs.CrawlerS3TargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Crawler("example", CrawlerArgs.builder()
* .databaseName(exampleAwsGlueCatalogDatabase.name())
* .name("example")
* .role(exampleAwsIamRole.arn())
* .s3Targets(CrawlerS3TargetArgs.builder()
* .path(String.format("s3://%s", exampleAwsS3Bucket.bucket()))
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:glue:Crawler
* properties:
* databaseName: ${exampleAwsGlueCatalogDatabase.name}
* name: example
* role: ${exampleAwsIamRole.arn}
* s3Targets:
* - path: s3://${exampleAwsS3Bucket.bucket}
* ```
*
* ### Catalog Target Example
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.glue.Crawler("example", {
* databaseName: exampleAwsGlueCatalogDatabase.name,
* name: "example",
* role: exampleAwsIamRole.arn,
* catalogTargets: [{
* databaseName: exampleAwsGlueCatalogDatabase.name,
* tables: [exampleAwsGlueCatalogTable.name],
* }],
* schemaChangePolicy: {
* deleteBehavior: "LOG",
* },
* configuration: `{
* "Version":1.0,
* "Grouping": {
* "TableGroupingPolicy": "CombineCompatibleSchemas"
* }
* }
* `,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.glue.Crawler("example",
* database_name=example_aws_glue_catalog_database["name"],
* name="example",
* role=example_aws_iam_role["arn"],
* catalog_targets=[{
* "database_name": example_aws_glue_catalog_database["name"],
* "tables": [example_aws_glue_catalog_table["name"]],
* }],
* schema_change_policy={
* "delete_behavior": "LOG",
* },
* configuration="""{
* "Version":1.0,
* "Grouping": {
* "TableGroupingPolicy": "CombineCompatibleSchemas"
* }
* }
* """)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Glue.Crawler("example", new()
* {
* DatabaseName = exampleAwsGlueCatalogDatabase.Name,
* Name = "example",
* Role = exampleAwsIamRole.Arn,
* CatalogTargets = new[]
* {
* new Aws.Glue.Inputs.CrawlerCatalogTargetArgs
* {
* DatabaseName = exampleAwsGlueCatalogDatabase.Name,
* Tables = new[]
* {
* exampleAwsGlueCatalogTable.Name,
* },
* },
* },
* SchemaChangePolicy = new Aws.Glue.Inputs.CrawlerSchemaChangePolicyArgs
* {
* DeleteBehavior = "LOG",
* },
* Configuration = @"{
* ""Version"":1.0,
* ""Grouping"": {
* ""TableGroupingPolicy"": ""CombineCompatibleSchemas""
* }
* }
* ",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/glue"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := glue.NewCrawler(ctx, "example", &glue.CrawlerArgs{
* DatabaseName: pulumi.Any(exampleAwsGlueCatalogDatabase.Name),
* Name: pulumi.String("example"),
* Role: pulumi.Any(exampleAwsIamRole.Arn),
* CatalogTargets: glue.CrawlerCatalogTargetArray{
* &glue.CrawlerCatalogTargetArgs{
* DatabaseName: pulumi.Any(exampleAwsGlueCatalogDatabase.Name),
* Tables: pulumi.StringArray{
* exampleAwsGlueCatalogTable.Name,
* },
* },
* },
* SchemaChangePolicy: &glue.CrawlerSchemaChangePolicyArgs{
* DeleteBehavior: pulumi.String("LOG"),
* },
* Configuration: pulumi.String(`{
* "Version":1.0,
* "Grouping": {
* "TableGroupingPolicy": "CombineCompatibleSchemas"
* }
* }
* `),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glue.Crawler;
* import com.pulumi.aws.glue.CrawlerArgs;
* import com.pulumi.aws.glue.inputs.CrawlerCatalogTargetArgs;
* import com.pulumi.aws.glue.inputs.CrawlerSchemaChangePolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Crawler("example", CrawlerArgs.builder()
* .databaseName(exampleAwsGlueCatalogDatabase.name())
* .name("example")
* .role(exampleAwsIamRole.arn())
* .catalogTargets(CrawlerCatalogTargetArgs.builder()
* .databaseName(exampleAwsGlueCatalogDatabase.name())
* .tables(exampleAwsGlueCatalogTable.name())
* .build())
* .schemaChangePolicy(CrawlerSchemaChangePolicyArgs.builder()
* .deleteBehavior("LOG")
* .build())
* .configuration("""
* {
* "Version":1.0,
* "Grouping": {
* "TableGroupingPolicy": "CombineCompatibleSchemas"
* }
* }
* """)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:glue:Crawler
* properties:
* databaseName: ${exampleAwsGlueCatalogDatabase.name}
* name: example
* role: ${exampleAwsIamRole.arn}
* catalogTargets:
* - databaseName: ${exampleAwsGlueCatalogDatabase.name}
* tables:
* - ${exampleAwsGlueCatalogTable.name}
* schemaChangePolicy:
* deleteBehavior: LOG
* configuration: |
* {
* "Version":1.0,
* "Grouping": {
* "TableGroupingPolicy": "CombineCompatibleSchemas"
* }
* }
* ```
*
* ### MongoDB Target Example
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.glue.Crawler("example", {
* databaseName: exampleAwsGlueCatalogDatabase.name,
* name: "example",
* role: exampleAwsIamRole.arn,
* mongodbTargets: [{
* connectionName: exampleAwsGlueConnection.name,
* path: "database-name/%",
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.glue.Crawler("example",
* database_name=example_aws_glue_catalog_database["name"],
* name="example",
* role=example_aws_iam_role["arn"],
* mongodb_targets=[{
* "connection_name": example_aws_glue_connection["name"],
* "path": "database-name/%",
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Glue.Crawler("example", new()
* {
* DatabaseName = exampleAwsGlueCatalogDatabase.Name,
* Name = "example",
* Role = exampleAwsIamRole.Arn,
* MongodbTargets = new[]
* {
* new Aws.Glue.Inputs.CrawlerMongodbTargetArgs
* {
* ConnectionName = exampleAwsGlueConnection.Name,
* Path = "database-name/%",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/glue"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := glue.NewCrawler(ctx, "example", &glue.CrawlerArgs{
* DatabaseName: pulumi.Any(exampleAwsGlueCatalogDatabase.Name),
* Name: pulumi.String("example"),
* Role: pulumi.Any(exampleAwsIamRole.Arn),
* MongodbTargets: glue.CrawlerMongodbTargetArray{
* &glue.CrawlerMongodbTargetArgs{
* ConnectionName: pulumi.Any(exampleAwsGlueConnection.Name),
* Path: pulumi.String("database-name/%"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glue.Crawler;
* import com.pulumi.aws.glue.CrawlerArgs;
* import com.pulumi.aws.glue.inputs.CrawlerMongodbTargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Crawler("example", CrawlerArgs.builder()
* .databaseName(exampleAwsGlueCatalogDatabase.name())
* .name("example")
* .role(exampleAwsIamRole.arn())
* .mongodbTargets(CrawlerMongodbTargetArgs.builder()
* .connectionName(exampleAwsGlueConnection.name())
* .path("database-name/%")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:glue:Crawler
* properties:
* databaseName: ${exampleAwsGlueCatalogDatabase.name}
* name: example
* role: ${exampleAwsIamRole.arn}
* mongodbTargets:
* - connectionName: ${exampleAwsGlueConnection.name}
* path: database-name/%
* ```
*
* ### Configuration Settings Example
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const eventsCrawler = new aws.glue.Crawler("events_crawler", {
* databaseName: glueDatabase.name,
* schedule: "cron(0 1 * * ? *)",
* name: `events_crawler_${environmentName}`,
* role: glueRole.arn,
* tags: tags,
* configuration: JSON.stringify({
* Grouping: {
* TableGroupingPolicy: "CombineCompatibleSchemas",
* },
* CrawlerOutput: {
* Partitions: {
* AddOrUpdateBehavior: "InheritFromTable",
* },
* },
* Version: 1,
* }),
* s3Targets: [{
* path: `s3://${dataLakeBucket.bucket}`,
* }],
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_aws as aws
* events_crawler = aws.glue.Crawler("events_crawler",
* database_name=glue_database["name"],
* schedule="cron(0 1 * * ? *)",
* name=f"events_crawler_{environment_name}",
* role=glue_role["arn"],
* tags=tags,
* configuration=json.dumps({
* "Grouping": {
* "TableGroupingPolicy": "CombineCompatibleSchemas",
* },
* "CrawlerOutput": {
* "Partitions": {
* "AddOrUpdateBehavior": "InheritFromTable",
* },
* },
* "Version": 1,
* }),
* s3_targets=[{
* "path": f"s3://{data_lake_bucket['bucket']}",
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var eventsCrawler = new Aws.Glue.Crawler("events_crawler", new()
* {
* DatabaseName = glueDatabase.Name,
* Schedule = "cron(0 1 * * ? *)",
* Name = $"events_crawler_{environmentName}",
* Role = glueRole.Arn,
* Tags = tags,
* Configuration = JsonSerializer.Serialize(new Dictionary
* {
* ["Grouping"] = new Dictionary
* {
* ["TableGroupingPolicy"] = "CombineCompatibleSchemas",
* },
* ["CrawlerOutput"] = new Dictionary
* {
* ["Partitions"] = new Dictionary
* {
* ["AddOrUpdateBehavior"] = "InheritFromTable",
* },
* },
* ["Version"] = 1,
* }),
* S3Targets = new[]
* {
* new Aws.Glue.Inputs.CrawlerS3TargetArgs
* {
* Path = $"s3://{dataLakeBucket.Bucket}",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "fmt"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/glue"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "Grouping": map[string]interface{}{
* "TableGroupingPolicy": "CombineCompatibleSchemas",
* },
* "CrawlerOutput": map[string]interface{}{
* "Partitions": map[string]interface{}{
* "AddOrUpdateBehavior": "InheritFromTable",
* },
* },
* "Version": 1,
* })
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* _, err = glue.NewCrawler(ctx, "events_crawler", &glue.CrawlerArgs{
* DatabaseName: pulumi.Any(glueDatabase.Name),
* Schedule: pulumi.String("cron(0 1 * * ? *)"),
* Name: pulumi.Sprintf("events_crawler_%v", environmentName),
* Role: pulumi.Any(glueRole.Arn),
* Tags: pulumi.Any(tags),
* Configuration: pulumi.String(json0),
* S3Targets: glue.CrawlerS3TargetArray{
* &glue.CrawlerS3TargetArgs{
* Path: pulumi.Sprintf("s3://%v", dataLakeBucket.Bucket),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glue.Crawler;
* import com.pulumi.aws.glue.CrawlerArgs;
* import com.pulumi.aws.glue.inputs.CrawlerS3TargetArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var eventsCrawler = new Crawler("eventsCrawler", CrawlerArgs.builder()
* .databaseName(glueDatabase.name())
* .schedule("cron(0 1 * * ? *)")
* .name(String.format("events_crawler_%s", environmentName))
* .role(glueRole.arn())
* .tags(tags)
* .configuration(serializeJson(
* jsonObject(
* jsonProperty("Grouping", jsonObject(
* jsonProperty("TableGroupingPolicy", "CombineCompatibleSchemas")
* )),
* jsonProperty("CrawlerOutput", jsonObject(
* jsonProperty("Partitions", jsonObject(
* jsonProperty("AddOrUpdateBehavior", "InheritFromTable")
* ))
* )),
* jsonProperty("Version", 1)
* )))
* .s3Targets(CrawlerS3TargetArgs.builder()
* .path(String.format("s3://%s", dataLakeBucket.bucket()))
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* eventsCrawler:
* type: aws:glue:Crawler
* name: events_crawler
* properties:
* databaseName: ${glueDatabase.name}
* schedule: cron(0 1 * * ? *)
* name: events_crawler_${environmentName}
* role: ${glueRole.arn}
* tags: ${tags}
* configuration:
* fn::toJSON:
* Grouping:
* TableGroupingPolicy: CombineCompatibleSchemas
* CrawlerOutput:
* Partitions:
* AddOrUpdateBehavior: InheritFromTable
* Version: 1
* s3Targets:
* - path: s3://${dataLakeBucket.bucket}
* ```
*
* ## Import
* Using `pulumi import`, import Glue Crawlers using `name`. For example:
* ```sh
* $ pulumi import aws:glue/crawler:Crawler MyJob MyJob
* ```
* @property catalogTargets List of nested AWS Glue Data Catalog target arguments. See Catalog Target below.
* @property classifiers List of custom classifiers. By default, all AWS classifiers are included in a crawl, but these custom classifiers always override the default classifiers for a given classification.
* @property configuration JSON string of configuration information. For more details see [Setting Crawler Configuration Options](https://docs.aws.amazon.com/glue/latest/dg/crawler-configuration.html).
* @property databaseName Glue database where results are written.
* @property deltaTargets List of nested Delta Lake target arguments. See Delta Target below.
* @property description Description of the crawler.
* @property dynamodbTargets List of nested DynamoDB target arguments. See Dynamodb Target below.
* @property hudiTargets List of nested Hudi target arguments. See Iceberg Target below.
* @property icebergTargets List of nested Iceberg target arguments. See Iceberg Target below.
* @property jdbcTargets List of nested JDBC target arguments. See JDBC Target below.
* @property lakeFormationConfiguration Specifies Lake Formation configuration settings for the crawler. See Lake Formation Configuration below.
* @property lineageConfiguration Specifies data lineage configuration settings for the crawler. See Lineage Configuration below.
* @property mongodbTargets List of nested MongoDB target arguments. See MongoDB Target below.
* @property name Name of the crawler.
* @property recrawlPolicy A policy that specifies whether to crawl the entire dataset again, or to crawl only folders that were added since the last crawler run.. See Recrawl Policy below.
* @property role The IAM role friendly name (including path without leading slash), or ARN of an IAM role, used by the crawler to access other resources.
* @property s3Targets List of nested Amazon S3 target arguments. See S3 Target below.
* @property schedule A cron expression used to specify the schedule. For more information, see [Time-Based Schedules for Jobs and Crawlers](https://docs.aws.amazon.com/glue/latest/dg/monitor-data-warehouse-schedule.html). For example, to run something every day at 12:15 UTC, you would specify: `cron(15 12 * * ? *)`.
* @property schemaChangePolicy Policy for the crawler's update and deletion behavior. See Schema Change Policy below.
* @property securityConfiguration The name of Security Configuration to be used by the crawler
* @property tablePrefix The table prefix used for catalog tables that are created.
* @property tags Key-value map of resource tags. .If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public data class CrawlerArgs(
public val catalogTargets: Output>? = null,
public val classifiers: Output>? = null,
public val configuration: Output? = null,
public val databaseName: Output? = null,
public val deltaTargets: Output>? = null,
public val description: Output? = null,
public val dynamodbTargets: Output>? = null,
public val hudiTargets: Output>? = null,
public val icebergTargets: Output>? = null,
public val jdbcTargets: Output>? = null,
public val lakeFormationConfiguration: Output? = null,
public val lineageConfiguration: Output? = null,
public val mongodbTargets: Output>? = null,
public val name: Output? = null,
public val recrawlPolicy: Output? = null,
public val role: Output? = null,
public val s3Targets: Output>? = null,
public val schedule: Output? = null,
public val schemaChangePolicy: Output? = null,
public val securityConfiguration: Output? = null,
public val tablePrefix: Output? = null,
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy