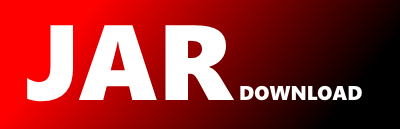
com.pulumi.aws.glue.kotlin.GlueFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.glue.kotlin
import com.pulumi.aws.glue.GlueFunctions.getCatalogTablePlain
import com.pulumi.aws.glue.GlueFunctions.getConnectionPlain
import com.pulumi.aws.glue.GlueFunctions.getDataCatalogEncryptionSettingsPlain
import com.pulumi.aws.glue.GlueFunctions.getRegistryPlain
import com.pulumi.aws.glue.GlueFunctions.getScriptPlain
import com.pulumi.aws.glue.kotlin.inputs.GetCatalogTablePlainArgs
import com.pulumi.aws.glue.kotlin.inputs.GetCatalogTablePlainArgsBuilder
import com.pulumi.aws.glue.kotlin.inputs.GetConnectionPlainArgs
import com.pulumi.aws.glue.kotlin.inputs.GetConnectionPlainArgsBuilder
import com.pulumi.aws.glue.kotlin.inputs.GetDataCatalogEncryptionSettingsPlainArgs
import com.pulumi.aws.glue.kotlin.inputs.GetDataCatalogEncryptionSettingsPlainArgsBuilder
import com.pulumi.aws.glue.kotlin.inputs.GetRegistryPlainArgs
import com.pulumi.aws.glue.kotlin.inputs.GetRegistryPlainArgsBuilder
import com.pulumi.aws.glue.kotlin.inputs.GetScriptDagEdge
import com.pulumi.aws.glue.kotlin.inputs.GetScriptDagNode
import com.pulumi.aws.glue.kotlin.inputs.GetScriptPlainArgs
import com.pulumi.aws.glue.kotlin.inputs.GetScriptPlainArgsBuilder
import com.pulumi.aws.glue.kotlin.outputs.GetCatalogTableResult
import com.pulumi.aws.glue.kotlin.outputs.GetConnectionResult
import com.pulumi.aws.glue.kotlin.outputs.GetDataCatalogEncryptionSettingsResult
import com.pulumi.aws.glue.kotlin.outputs.GetRegistryResult
import com.pulumi.aws.glue.kotlin.outputs.GetScriptResult
import kotlinx.coroutines.future.await
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.aws.glue.kotlin.outputs.GetCatalogTableResult.Companion.toKotlin as getCatalogTableResultToKotlin
import com.pulumi.aws.glue.kotlin.outputs.GetConnectionResult.Companion.toKotlin as getConnectionResultToKotlin
import com.pulumi.aws.glue.kotlin.outputs.GetDataCatalogEncryptionSettingsResult.Companion.toKotlin as getDataCatalogEncryptionSettingsResultToKotlin
import com.pulumi.aws.glue.kotlin.outputs.GetRegistryResult.Companion.toKotlin as getRegistryResultToKotlin
import com.pulumi.aws.glue.kotlin.outputs.GetScriptResult.Companion.toKotlin as getScriptResultToKotlin
public object GlueFunctions {
/**
* This data source can be used to fetch information about an AWS Glue Data Catalog Table.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = aws.glue.getCatalogTable({
* name: "MyCatalogTable",
* databaseName: "MyCatalogDatabase",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.glue.get_catalog_table(name="MyCatalogTable",
* database_name="MyCatalogDatabase")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = Aws.Glue.GetCatalogTable.Invoke(new()
* {
* Name = "MyCatalogTable",
* DatabaseName = "MyCatalogDatabase",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/glue"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := glue.LookupCatalogTable(ctx, &glue.LookupCatalogTableArgs{
* Name: "MyCatalogTable",
* DatabaseName: "MyCatalogDatabase",
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glue.GlueFunctions;
* import com.pulumi.aws.glue.inputs.GetCatalogTableArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = GlueFunctions.getCatalogTable(GetCatalogTableArgs.builder()
* .name("MyCatalogTable")
* .databaseName("MyCatalogDatabase")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: aws:glue:getCatalogTable
* Arguments:
* name: MyCatalogTable
* databaseName: MyCatalogDatabase
* ```
*
* @param argument A collection of arguments for invoking getCatalogTable.
* @return A collection of values returned by getCatalogTable.
*/
public suspend fun getCatalogTable(argument: GetCatalogTablePlainArgs): GetCatalogTableResult =
getCatalogTableResultToKotlin(getCatalogTablePlain(argument.toJava()).await())
/**
* @see [getCatalogTable].
* @param catalogId ID of the Glue Catalog and database where the table metadata resides. If omitted, this defaults to the current AWS Account ID.
* @param databaseName Name of the metadata database where the table metadata resides.
* @param name Name of the table.
* @param queryAsOfTime The time as of when to read the table contents. If not set, the most recent transaction commit time will be used. Cannot be specified along with `transaction_id`. Specified in RFC 3339 format, e.g. `2006-01-02T15:04:05Z07:00`.
* @param transactionId The transaction ID at which to read the table contents.
* @return A collection of values returned by getCatalogTable.
*/
public suspend fun getCatalogTable(
catalogId: String? = null,
databaseName: String,
name: String,
queryAsOfTime: String? = null,
transactionId: Int? = null,
): GetCatalogTableResult {
val argument = GetCatalogTablePlainArgs(
catalogId = catalogId,
databaseName = databaseName,
name = name,
queryAsOfTime = queryAsOfTime,
transactionId = transactionId,
)
return getCatalogTableResultToKotlin(getCatalogTablePlain(argument.toJava()).await())
}
/**
* @see [getCatalogTable].
* @param argument Builder for [com.pulumi.aws.glue.kotlin.inputs.GetCatalogTablePlainArgs].
* @return A collection of values returned by getCatalogTable.
*/
public suspend fun getCatalogTable(argument: suspend GetCatalogTablePlainArgsBuilder.() -> Unit): GetCatalogTableResult {
val builder = GetCatalogTablePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getCatalogTableResultToKotlin(getCatalogTablePlain(builtArgument.toJava()).await())
}
/**
* This data source can be used to fetch information about a specific Glue Connection.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = aws.glue.getConnection({
* id: "123456789123:connection",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.glue.get_connection(id="123456789123:connection")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = Aws.Glue.GetConnection.Invoke(new()
* {
* Id = "123456789123:connection",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/glue"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := glue.LookupConnection(ctx, &glue.LookupConnectionArgs{
* Id: "123456789123:connection",
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glue.GlueFunctions;
* import com.pulumi.aws.glue.inputs.GetConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = GlueFunctions.getConnection(GetConnectionArgs.builder()
* .id("123456789123:connection")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: aws:glue:getConnection
* Arguments:
* id: 123456789123:connection
* ```
*
* @param argument A collection of arguments for invoking getConnection.
* @return A collection of values returned by getConnection.
*/
public suspend fun getConnection(argument: GetConnectionPlainArgs): GetConnectionResult =
getConnectionResultToKotlin(getConnectionPlain(argument.toJava()).await())
/**
* @see [getConnection].
* @param id Concatenation of the catalog ID and connection name. For example, if your account ID is
* `123456789123` and the connection name is `conn` then the ID is `123456789123:conn`.
* @param tags Tags assigned to the resource
* @return A collection of values returned by getConnection.
*/
public suspend fun getConnection(id: String, tags: Map? = null): GetConnectionResult {
val argument = GetConnectionPlainArgs(
id = id,
tags = tags,
)
return getConnectionResultToKotlin(getConnectionPlain(argument.toJava()).await())
}
/**
* @see [getConnection].
* @param argument Builder for [com.pulumi.aws.glue.kotlin.inputs.GetConnectionPlainArgs].
* @return A collection of values returned by getConnection.
*/
public suspend fun getConnection(argument: suspend GetConnectionPlainArgsBuilder.() -> Unit): GetConnectionResult {
val builder = GetConnectionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getConnectionResultToKotlin(getConnectionPlain(builtArgument.toJava()).await())
}
/**
* This data source can be used to fetch information about AWS Glue Data Catalog Encryption Settings.
* ## Example Usage
*
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glue.GlueFunctions;
* import com.pulumi.aws.glue.inputs.GetDataCatalogEncryptionSettingsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = GlueFunctions.getDataCatalogEncryptionSettings(GetDataCatalogEncryptionSettingsArgs.builder()
* .id("123456789123")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: aws:glue:getDataCatalogEncryptionSettings
* Arguments:
* id: '123456789123'
* ```
*
* @param argument A collection of arguments for invoking getDataCatalogEncryptionSettings.
* @return A collection of values returned by getDataCatalogEncryptionSettings.
*/
public suspend fun getDataCatalogEncryptionSettings(argument: GetDataCatalogEncryptionSettingsPlainArgs): GetDataCatalogEncryptionSettingsResult =
getDataCatalogEncryptionSettingsResultToKotlin(getDataCatalogEncryptionSettingsPlain(argument.toJava()).await())
/**
* @see [getDataCatalogEncryptionSettings].
* @param catalogId ID of the Data Catalog. This is typically the AWS account ID.
* @return A collection of values returned by getDataCatalogEncryptionSettings.
*/
public suspend fun getDataCatalogEncryptionSettings(catalogId: String): GetDataCatalogEncryptionSettingsResult {
val argument = GetDataCatalogEncryptionSettingsPlainArgs(
catalogId = catalogId,
)
return getDataCatalogEncryptionSettingsResultToKotlin(getDataCatalogEncryptionSettingsPlain(argument.toJava()).await())
}
/**
* @see [getDataCatalogEncryptionSettings].
* @param argument Builder for [com.pulumi.aws.glue.kotlin.inputs.GetDataCatalogEncryptionSettingsPlainArgs].
* @return A collection of values returned by getDataCatalogEncryptionSettings.
*/
public suspend fun getDataCatalogEncryptionSettings(argument: suspend GetDataCatalogEncryptionSettingsPlainArgsBuilder.() -> Unit): GetDataCatalogEncryptionSettingsResult {
val builder = GetDataCatalogEncryptionSettingsPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getDataCatalogEncryptionSettingsResultToKotlin(getDataCatalogEncryptionSettingsPlain(builtArgument.toJava()).await())
}
/**
* Data source for managing an AWS Glue Registry.
* ## Example Usage
* ### Basic Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = aws.glue.getRegistry({
* name: "example",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.glue.get_registry(name="example")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = Aws.Glue.GetRegistry.Invoke(new()
* {
* Name = "example",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/glue"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := glue.LookupRegistry(ctx, &glue.LookupRegistryArgs{
* Name: "example",
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glue.GlueFunctions;
* import com.pulumi.aws.glue.inputs.GetRegistryArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = GlueFunctions.getRegistry(GetRegistryArgs.builder()
* .name("example")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: aws:glue:getRegistry
* Arguments:
* name: example
* ```
*
* @param argument A collection of arguments for invoking getRegistry.
* @return A collection of values returned by getRegistry.
*/
public suspend fun getRegistry(argument: GetRegistryPlainArgs): GetRegistryResult =
getRegistryResultToKotlin(getRegistryPlain(argument.toJava()).await())
/**
* @see [getRegistry].
* @param name Name of the Glue Registry.
* @return A collection of values returned by getRegistry.
*/
public suspend fun getRegistry(name: String): GetRegistryResult {
val argument = GetRegistryPlainArgs(
name = name,
)
return getRegistryResultToKotlin(getRegistryPlain(argument.toJava()).await())
}
/**
* @see [getRegistry].
* @param argument Builder for [com.pulumi.aws.glue.kotlin.inputs.GetRegistryPlainArgs].
* @return A collection of values returned by getRegistry.
*/
public suspend fun getRegistry(argument: suspend GetRegistryPlainArgsBuilder.() -> Unit): GetRegistryResult {
val builder = GetRegistryPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getRegistryResultToKotlin(getRegistryPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to generate a Glue script from a Directed Acyclic Graph (DAG).
* ## Example Usage
* ### Generate Python Script
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = aws.glue.getScript({
* language: "PYTHON",
* dagEdges: [
* {
* source: "datasource0",
* target: "applymapping1",
* },
* {
* source: "applymapping1",
* target: "selectfields2",
* },
* {
* source: "selectfields2",
* target: "resolvechoice3",
* },
* {
* source: "resolvechoice3",
* target: "datasink4",
* },
* ],
* dagNodes: [
* {
* id: "datasource0",
* nodeType: "DataSource",
* args: [
* {
* name: "database",
* value: `"${source.name}"`,
* },
* {
* name: "table_name",
* value: `"${sourceAwsGlueCatalogTable.name}"`,
* },
* ],
* },
* {
* id: "applymapping1",
* nodeType: "ApplyMapping",
* args: [{
* name: "mapping",
* value: "[(\"column1\", \"string\", \"column1\", \"string\")]",
* }],
* },
* {
* id: "selectfields2",
* nodeType: "SelectFields",
* args: [{
* name: "paths",
* value: "[\"column1\"]",
* }],
* },
* {
* id: "resolvechoice3",
* nodeType: "ResolveChoice",
* args: [
* {
* name: "choice",
* value: "\"MATCH_CATALOG\"",
* },
* {
* name: "database",
* value: `"${destination.name}"`,
* },
* {
* name: "table_name",
* value: `"${destinationAwsGlueCatalogTable.name}"`,
* },
* ],
* },
* {
* id: "datasink4",
* nodeType: "DataSink",
* args: [
* {
* name: "database",
* value: `"${destination.name}"`,
* },
* {
* name: "table_name",
* value: `"${destinationAwsGlueCatalogTable.name}"`,
* },
* ],
* },
* ],
* });
* export const pythonScript = example.then(example => example.pythonScript);
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.glue.get_script(language="PYTHON",
* dag_edges=[
* {
* "source": "datasource0",
* "target": "applymapping1",
* },
* {
* "source": "applymapping1",
* "target": "selectfields2",
* },
* {
* "source": "selectfields2",
* "target": "resolvechoice3",
* },
* {
* "source": "resolvechoice3",
* "target": "datasink4",
* },
* ],
* dag_nodes=[
* {
* "id": "datasource0",
* "node_type": "DataSource",
* "args": [
* {
* "name": "database",
* "value": f"\"{source['name']}\"",
* },
* {
* "name": "table_name",
* "value": f"\"{source_aws_glue_catalog_table['name']}\"",
* },
* ],
* },
* {
* "id": "applymapping1",
* "node_type": "ApplyMapping",
* "args": [{
* "name": "mapping",
* "value": "[(\"column1\", \"string\", \"column1\", \"string\")]",
* }],
* },
* {
* "id": "selectfields2",
* "node_type": "SelectFields",
* "args": [{
* "name": "paths",
* "value": "[\"column1\"]",
* }],
* },
* {
* "id": "resolvechoice3",
* "node_type": "ResolveChoice",
* "args": [
* {
* "name": "choice",
* "value": "\"MATCH_CATALOG\"",
* },
* {
* "name": "database",
* "value": f"\"{destination['name']}\"",
* },
* {
* "name": "table_name",
* "value": f"\"{destination_aws_glue_catalog_table['name']}\"",
* },
* ],
* },
* {
* "id": "datasink4",
* "node_type": "DataSink",
* "args": [
* {
* "name": "database",
* "value": f"\"{destination['name']}\"",
* },
* {
* "name": "table_name",
* "value": f"\"{destination_aws_glue_catalog_table['name']}\"",
* },
* ],
* },
* ])
* pulumi.export("pythonScript", example.python_script)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = Aws.Glue.GetScript.Invoke(new()
* {
* Language = "PYTHON",
* DagEdges = new[]
* {
* new Aws.Glue.Inputs.GetScriptDagEdgeInputArgs
* {
* Source = "datasource0",
* Target = "applymapping1",
* },
* new Aws.Glue.Inputs.GetScriptDagEdgeInputArgs
* {
* Source = "applymapping1",
* Target = "selectfields2",
* },
* new Aws.Glue.Inputs.GetScriptDagEdgeInputArgs
* {
* Source = "selectfields2",
* Target = "resolvechoice3",
* },
* new Aws.Glue.Inputs.GetScriptDagEdgeInputArgs
* {
* Source = "resolvechoice3",
* Target = "datasink4",
* },
* },
* DagNodes = new[]
* {
* new Aws.Glue.Inputs.GetScriptDagNodeInputArgs
* {
* Id = "datasource0",
* NodeType = "DataSource",
* Args = new[]
* {
* new Aws.Glue.Inputs.GetScriptDagNodeArgInputArgs
* {
* Name = "database",
* Value = $"\"{source.Name}\"",
* },
* new Aws.Glue.Inputs.GetScriptDagNodeArgInputArgs
* {
* Name = "table_name",
* Value = $"\"{sourceAwsGlueCatalogTable.Name}\"",
* },
* },
* },
* new Aws.Glue.Inputs.GetScriptDagNodeInputArgs
* {
* Id = "applymapping1",
* NodeType = "ApplyMapping",
* Args = new[]
* {
* new Aws.Glue.Inputs.GetScriptDagNodeArgInputArgs
* {
* Name = "mapping",
* Value = "[(\"column1\", \"string\", \"column1\", \"string\")]",
* },
* },
* },
* new Aws.Glue.Inputs.GetScriptDagNodeInputArgs
* {
* Id = "selectfields2",
* NodeType = "SelectFields",
* Args = new[]
* {
* new Aws.Glue.Inputs.GetScriptDagNodeArgInputArgs
* {
* Name = "paths",
* Value = "[\"column1\"]",
* },
* },
* },
* new Aws.Glue.Inputs.GetScriptDagNodeInputArgs
* {
* Id = "resolvechoice3",
* NodeType = "ResolveChoice",
* Args = new[]
* {
* new Aws.Glue.Inputs.GetScriptDagNodeArgInputArgs
* {
* Name = "choice",
* Value = "\"MATCH_CATALOG\"",
* },
* new Aws.Glue.Inputs.GetScriptDagNodeArgInputArgs
* {
* Name = "database",
* Value = $"\"{destination.Name}\"",
* },
* new Aws.Glue.Inputs.GetScriptDagNodeArgInputArgs
* {
* Name = "table_name",
* Value = $"\"{destinationAwsGlueCatalogTable.Name}\"",
* },
* },
* },
* new Aws.Glue.Inputs.GetScriptDagNodeInputArgs
* {
* Id = "datasink4",
* NodeType = "DataSink",
* Args = new[]
* {
* new Aws.Glue.Inputs.GetScriptDagNodeArgInputArgs
* {
* Name = "database",
* Value = $"\"{destination.Name}\"",
* },
* new Aws.Glue.Inputs.GetScriptDagNodeArgInputArgs
* {
* Name = "table_name",
* Value = $"\"{destinationAwsGlueCatalogTable.Name}\"",
* },
* },
* },
* },
* });
* return new Dictionary
* {
* ["pythonScript"] = example.Apply(getScriptResult => getScriptResult.PythonScript),
* };
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/glue"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := glue.GetScript(ctx, &glue.GetScriptArgs{
* Language: pulumi.StringRef("PYTHON"),
* DagEdges: []glue.GetScriptDagEdge{
* {
* Source: "datasource0",
* Target: "applymapping1",
* },
* {
* Source: "applymapping1",
* Target: "selectfields2",
* },
* {
* Source: "selectfields2",
* Target: "resolvechoice3",
* },
* {
* Source: "resolvechoice3",
* Target: "datasink4",
* },
* },
* DagNodes: []glue.GetScriptDagNode{
* {
* Id: "datasource0",
* NodeType: "DataSource",
* Args: []glue.GetScriptDagNodeArg{
* {
* Name: "database",
* Value: fmt.Sprintf("\"%v\"", source.Name),
* },
* {
* Name: "table_name",
* Value: fmt.Sprintf("\"%v\"", sourceAwsGlueCatalogTable.Name),
* },
* },
* },
* {
* Id: "applymapping1",
* NodeType: "ApplyMapping",
* Args: []glue.GetScriptDagNodeArg{
* {
* Name: "mapping",
* Value: "[(\"column1\", \"string\", \"column1\", \"string\")]",
* },
* },
* },
* {
* Id: "selectfields2",
* NodeType: "SelectFields",
* Args: []glue.GetScriptDagNodeArg{
* {
* Name: "paths",
* Value: "[\"column1\"]",
* },
* },
* },
* {
* Id: "resolvechoice3",
* NodeType: "ResolveChoice",
* Args: []glue.GetScriptDagNodeArg{
* {
* Name: "choice",
* Value: "\"MATCH_CATALOG\"",
* },
* {
* Name: "database",
* Value: fmt.Sprintf("\"%v\"", destination.Name),
* },
* {
* Name: "table_name",
* Value: fmt.Sprintf("\"%v\"", destinationAwsGlueCatalogTable.Name),
* },
* },
* },
* {
* Id: "datasink4",
* NodeType: "DataSink",
* Args: []glue.GetScriptDagNodeArg{
* {
* Name: "database",
* Value: fmt.Sprintf("\"%v\"", destination.Name),
* },
* {
* Name: "table_name",
* Value: fmt.Sprintf("\"%v\"", destinationAwsGlueCatalogTable.Name),
* },
* },
* },
* },
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("pythonScript", example.PythonScript)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glue.GlueFunctions;
* import com.pulumi.aws.glue.inputs.GetScriptArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = GlueFunctions.getScript(GetScriptArgs.builder()
* .language("PYTHON")
* .dagEdges(
* GetScriptDagEdgeArgs.builder()
* .source("datasource0")
* .target("applymapping1")
* .build(),
* GetScriptDagEdgeArgs.builder()
* .source("applymapping1")
* .target("selectfields2")
* .build(),
* GetScriptDagEdgeArgs.builder()
* .source("selectfields2")
* .target("resolvechoice3")
* .build(),
* GetScriptDagEdgeArgs.builder()
* .source("resolvechoice3")
* .target("datasink4")
* .build())
* .dagNodes(
* GetScriptDagNodeArgs.builder()
* .id("datasource0")
* .nodeType("DataSource")
* .args(
* GetScriptDagNodeArgArgs.builder()
* .name("database")
* .value(String.format("\"%s\"", source.name()))
* .build(),
* GetScriptDagNodeArgArgs.builder()
* .name("table_name")
* .value(String.format("\"%s\"", sourceAwsGlueCatalogTable.name()))
* .build())
* .build(),
* GetScriptDagNodeArgs.builder()
* .id("applymapping1")
* .nodeType("ApplyMapping")
* .args(GetScriptDagNodeArgArgs.builder()
* .name("mapping")
* .value("[(\"column1\", \"string\", \"column1\", \"string\")]")
* .build())
* .build(),
* GetScriptDagNodeArgs.builder()
* .id("selectfields2")
* .nodeType("SelectFields")
* .args(GetScriptDagNodeArgArgs.builder()
* .name("paths")
* .value("[\"column1\"]")
* .build())
* .build(),
* GetScriptDagNodeArgs.builder()
* .id("resolvechoice3")
* .nodeType("ResolveChoice")
* .args(
* GetScriptDagNodeArgArgs.builder()
* .name("choice")
* .value("\"MATCH_CATALOG\"")
* .build(),
* GetScriptDagNodeArgArgs.builder()
* .name("database")
* .value(String.format("\"%s\"", destination.name()))
* .build(),
* GetScriptDagNodeArgArgs.builder()
* .name("table_name")
* .value(String.format("\"%s\"", destinationAwsGlueCatalogTable.name()))
* .build())
* .build(),
* GetScriptDagNodeArgs.builder()
* .id("datasink4")
* .nodeType("DataSink")
* .args(
* GetScriptDagNodeArgArgs.builder()
* .name("database")
* .value(String.format("\"%s\"", destination.name()))
* .build(),
* GetScriptDagNodeArgArgs.builder()
* .name("table_name")
* .value(String.format("\"%s\"", destinationAwsGlueCatalogTable.name()))
* .build())
* .build())
* .build());
* ctx.export("pythonScript", example.applyValue(getScriptResult -> getScriptResult.pythonScript()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: aws:glue:getScript
* Arguments:
* language: PYTHON
* dagEdges:
* - source: datasource0
* target: applymapping1
* - source: applymapping1
* target: selectfields2
* - source: selectfields2
* target: resolvechoice3
* - source: resolvechoice3
* target: datasink4
* dagNodes:
* - id: datasource0
* nodeType: DataSource
* args:
* - name: database
* value: '"${source.name}"'
* - name: table_name
* value: '"${sourceAwsGlueCatalogTable.name}"'
* - id: applymapping1
* nodeType: ApplyMapping
* args:
* - name: mapping
* value: '[("column1", "string", "column1", "string")]'
* - id: selectfields2
* nodeType: SelectFields
* args:
* - name: paths
* value: '["column1"]'
* - id: resolvechoice3
* nodeType: ResolveChoice
* args:
* - name: choice
* value: '"MATCH_CATALOG"'
* - name: database
* value: '"${destination.name}"'
* - name: table_name
* value: '"${destinationAwsGlueCatalogTable.name}"'
* - id: datasink4
* nodeType: DataSink
* args:
* - name: database
* value: '"${destination.name}"'
* - name: table_name
* value: '"${destinationAwsGlueCatalogTable.name}"'
* outputs:
* pythonScript: ${example.pythonScript}
* ```
*
* ### Generate Scala Code
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = aws.glue.getScript({
* language: "SCALA",
* dagEdges: [
* {
* source: "datasource0",
* target: "applymapping1",
* },
* {
* source: "applymapping1",
* target: "selectfields2",
* },
* {
* source: "selectfields2",
* target: "resolvechoice3",
* },
* {
* source: "resolvechoice3",
* target: "datasink4",
* },
* ],
* dagNodes: [
* {
* id: "datasource0",
* nodeType: "DataSource",
* args: [
* {
* name: "database",
* value: `"${source.name}"`,
* },
* {
* name: "table_name",
* value: `"${sourceAwsGlueCatalogTable.name}"`,
* },
* ],
* },
* {
* id: "applymapping1",
* nodeType: "ApplyMapping",
* args: [{
* name: "mappings",
* value: "[(\"column1\", \"string\", \"column1\", \"string\")]",
* }],
* },
* {
* id: "selectfields2",
* nodeType: "SelectFields",
* args: [{
* name: "paths",
* value: "[\"column1\"]",
* }],
* },
* {
* id: "resolvechoice3",
* nodeType: "ResolveChoice",
* args: [
* {
* name: "choice",
* value: "\"MATCH_CATALOG\"",
* },
* {
* name: "database",
* value: `"${destination.name}"`,
* },
* {
* name: "table_name",
* value: `"${destinationAwsGlueCatalogTable.name}"`,
* },
* ],
* },
* {
* id: "datasink4",
* nodeType: "DataSink",
* args: [
* {
* name: "database",
* value: `"${destination.name}"`,
* },
* {
* name: "table_name",
* value: `"${destinationAwsGlueCatalogTable.name}"`,
* },
* ],
* },
* ],
* });
* export const scalaCode = example.then(example => example.scalaCode);
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.glue.get_script(language="SCALA",
* dag_edges=[
* {
* "source": "datasource0",
* "target": "applymapping1",
* },
* {
* "source": "applymapping1",
* "target": "selectfields2",
* },
* {
* "source": "selectfields2",
* "target": "resolvechoice3",
* },
* {
* "source": "resolvechoice3",
* "target": "datasink4",
* },
* ],
* dag_nodes=[
* {
* "id": "datasource0",
* "node_type": "DataSource",
* "args": [
* {
* "name": "database",
* "value": f"\"{source['name']}\"",
* },
* {
* "name": "table_name",
* "value": f"\"{source_aws_glue_catalog_table['name']}\"",
* },
* ],
* },
* {
* "id": "applymapping1",
* "node_type": "ApplyMapping",
* "args": [{
* "name": "mappings",
* "value": "[(\"column1\", \"string\", \"column1\", \"string\")]",
* }],
* },
* {
* "id": "selectfields2",
* "node_type": "SelectFields",
* "args": [{
* "name": "paths",
* "value": "[\"column1\"]",
* }],
* },
* {
* "id": "resolvechoice3",
* "node_type": "ResolveChoice",
* "args": [
* {
* "name": "choice",
* "value": "\"MATCH_CATALOG\"",
* },
* {
* "name": "database",
* "value": f"\"{destination['name']}\"",
* },
* {
* "name": "table_name",
* "value": f"\"{destination_aws_glue_catalog_table['name']}\"",
* },
* ],
* },
* {
* "id": "datasink4",
* "node_type": "DataSink",
* "args": [
* {
* "name": "database",
* "value": f"\"{destination['name']}\"",
* },
* {
* "name": "table_name",
* "value": f"\"{destination_aws_glue_catalog_table['name']}\"",
* },
* ],
* },
* ])
* pulumi.export("scalaCode", example.scala_code)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = Aws.Glue.GetScript.Invoke(new()
* {
* Language = "SCALA",
* DagEdges = new[]
* {
* new Aws.Glue.Inputs.GetScriptDagEdgeInputArgs
* {
* Source = "datasource0",
* Target = "applymapping1",
* },
* new Aws.Glue.Inputs.GetScriptDagEdgeInputArgs
* {
* Source = "applymapping1",
* Target = "selectfields2",
* },
* new Aws.Glue.Inputs.GetScriptDagEdgeInputArgs
* {
* Source = "selectfields2",
* Target = "resolvechoice3",
* },
* new Aws.Glue.Inputs.GetScriptDagEdgeInputArgs
* {
* Source = "resolvechoice3",
* Target = "datasink4",
* },
* },
* DagNodes = new[]
* {
* new Aws.Glue.Inputs.GetScriptDagNodeInputArgs
* {
* Id = "datasource0",
* NodeType = "DataSource",
* Args = new[]
* {
* new Aws.Glue.Inputs.GetScriptDagNodeArgInputArgs
* {
* Name = "database",
* Value = $"\"{source.Name}\"",
* },
* new Aws.Glue.Inputs.GetScriptDagNodeArgInputArgs
* {
* Name = "table_name",
* Value = $"\"{sourceAwsGlueCatalogTable.Name}\"",
* },
* },
* },
* new Aws.Glue.Inputs.GetScriptDagNodeInputArgs
* {
* Id = "applymapping1",
* NodeType = "ApplyMapping",
* Args = new[]
* {
* new Aws.Glue.Inputs.GetScriptDagNodeArgInputArgs
* {
* Name = "mappings",
* Value = "[(\"column1\", \"string\", \"column1\", \"string\")]",
* },
* },
* },
* new Aws.Glue.Inputs.GetScriptDagNodeInputArgs
* {
* Id = "selectfields2",
* NodeType = "SelectFields",
* Args = new[]
* {
* new Aws.Glue.Inputs.GetScriptDagNodeArgInputArgs
* {
* Name = "paths",
* Value = "[\"column1\"]",
* },
* },
* },
* new Aws.Glue.Inputs.GetScriptDagNodeInputArgs
* {
* Id = "resolvechoice3",
* NodeType = "ResolveChoice",
* Args = new[]
* {
* new Aws.Glue.Inputs.GetScriptDagNodeArgInputArgs
* {
* Name = "choice",
* Value = "\"MATCH_CATALOG\"",
* },
* new Aws.Glue.Inputs.GetScriptDagNodeArgInputArgs
* {
* Name = "database",
* Value = $"\"{destination.Name}\"",
* },
* new Aws.Glue.Inputs.GetScriptDagNodeArgInputArgs
* {
* Name = "table_name",
* Value = $"\"{destinationAwsGlueCatalogTable.Name}\"",
* },
* },
* },
* new Aws.Glue.Inputs.GetScriptDagNodeInputArgs
* {
* Id = "datasink4",
* NodeType = "DataSink",
* Args = new[]
* {
* new Aws.Glue.Inputs.GetScriptDagNodeArgInputArgs
* {
* Name = "database",
* Value = $"\"{destination.Name}\"",
* },
* new Aws.Glue.Inputs.GetScriptDagNodeArgInputArgs
* {
* Name = "table_name",
* Value = $"\"{destinationAwsGlueCatalogTable.Name}\"",
* },
* },
* },
* },
* });
* return new Dictionary
* {
* ["scalaCode"] = example.Apply(getScriptResult => getScriptResult.ScalaCode),
* };
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/glue"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := glue.GetScript(ctx, &glue.GetScriptArgs{
* Language: pulumi.StringRef("SCALA"),
* DagEdges: []glue.GetScriptDagEdge{
* {
* Source: "datasource0",
* Target: "applymapping1",
* },
* {
* Source: "applymapping1",
* Target: "selectfields2",
* },
* {
* Source: "selectfields2",
* Target: "resolvechoice3",
* },
* {
* Source: "resolvechoice3",
* Target: "datasink4",
* },
* },
* DagNodes: []glue.GetScriptDagNode{
* {
* Id: "datasource0",
* NodeType: "DataSource",
* Args: []glue.GetScriptDagNodeArg{
* {
* Name: "database",
* Value: fmt.Sprintf("\"%v\"", source.Name),
* },
* {
* Name: "table_name",
* Value: fmt.Sprintf("\"%v\"", sourceAwsGlueCatalogTable.Name),
* },
* },
* },
* {
* Id: "applymapping1",
* NodeType: "ApplyMapping",
* Args: []glue.GetScriptDagNodeArg{
* {
* Name: "mappings",
* Value: "[(\"column1\", \"string\", \"column1\", \"string\")]",
* },
* },
* },
* {
* Id: "selectfields2",
* NodeType: "SelectFields",
* Args: []glue.GetScriptDagNodeArg{
* {
* Name: "paths",
* Value: "[\"column1\"]",
* },
* },
* },
* {
* Id: "resolvechoice3",
* NodeType: "ResolveChoice",
* Args: []glue.GetScriptDagNodeArg{
* {
* Name: "choice",
* Value: "\"MATCH_CATALOG\"",
* },
* {
* Name: "database",
* Value: fmt.Sprintf("\"%v\"", destination.Name),
* },
* {
* Name: "table_name",
* Value: fmt.Sprintf("\"%v\"", destinationAwsGlueCatalogTable.Name),
* },
* },
* },
* {
* Id: "datasink4",
* NodeType: "DataSink",
* Args: []glue.GetScriptDagNodeArg{
* {
* Name: "database",
* Value: fmt.Sprintf("\"%v\"", destination.Name),
* },
* {
* Name: "table_name",
* Value: fmt.Sprintf("\"%v\"", destinationAwsGlueCatalogTable.Name),
* },
* },
* },
* },
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("scalaCode", example.ScalaCode)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glue.GlueFunctions;
* import com.pulumi.aws.glue.inputs.GetScriptArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = GlueFunctions.getScript(GetScriptArgs.builder()
* .language("SCALA")
* .dagEdges(
* GetScriptDagEdgeArgs.builder()
* .source("datasource0")
* .target("applymapping1")
* .build(),
* GetScriptDagEdgeArgs.builder()
* .source("applymapping1")
* .target("selectfields2")
* .build(),
* GetScriptDagEdgeArgs.builder()
* .source("selectfields2")
* .target("resolvechoice3")
* .build(),
* GetScriptDagEdgeArgs.builder()
* .source("resolvechoice3")
* .target("datasink4")
* .build())
* .dagNodes(
* GetScriptDagNodeArgs.builder()
* .id("datasource0")
* .nodeType("DataSource")
* .args(
* GetScriptDagNodeArgArgs.builder()
* .name("database")
* .value(String.format("\"%s\"", source.name()))
* .build(),
* GetScriptDagNodeArgArgs.builder()
* .name("table_name")
* .value(String.format("\"%s\"", sourceAwsGlueCatalogTable.name()))
* .build())
* .build(),
* GetScriptDagNodeArgs.builder()
* .id("applymapping1")
* .nodeType("ApplyMapping")
* .args(GetScriptDagNodeArgArgs.builder()
* .name("mappings")
* .value("[(\"column1\", \"string\", \"column1\", \"string\")]")
* .build())
* .build(),
* GetScriptDagNodeArgs.builder()
* .id("selectfields2")
* .nodeType("SelectFields")
* .args(GetScriptDagNodeArgArgs.builder()
* .name("paths")
* .value("[\"column1\"]")
* .build())
* .build(),
* GetScriptDagNodeArgs.builder()
* .id("resolvechoice3")
* .nodeType("ResolveChoice")
* .args(
* GetScriptDagNodeArgArgs.builder()
* .name("choice")
* .value("\"MATCH_CATALOG\"")
* .build(),
* GetScriptDagNodeArgArgs.builder()
* .name("database")
* .value(String.format("\"%s\"", destination.name()))
* .build(),
* GetScriptDagNodeArgArgs.builder()
* .name("table_name")
* .value(String.format("\"%s\"", destinationAwsGlueCatalogTable.name()))
* .build())
* .build(),
* GetScriptDagNodeArgs.builder()
* .id("datasink4")
* .nodeType("DataSink")
* .args(
* GetScriptDagNodeArgArgs.builder()
* .name("database")
* .value(String.format("\"%s\"", destination.name()))
* .build(),
* GetScriptDagNodeArgArgs.builder()
* .name("table_name")
* .value(String.format("\"%s\"", destinationAwsGlueCatalogTable.name()))
* .build())
* .build())
* .build());
* ctx.export("scalaCode", example.applyValue(getScriptResult -> getScriptResult.scalaCode()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: aws:glue:getScript
* Arguments:
* language: SCALA
* dagEdges:
* - source: datasource0
* target: applymapping1
* - source: applymapping1
* target: selectfields2
* - source: selectfields2
* target: resolvechoice3
* - source: resolvechoice3
* target: datasink4
* dagNodes:
* - id: datasource0
* nodeType: DataSource
* args:
* - name: database
* value: '"${source.name}"'
* - name: table_name
* value: '"${sourceAwsGlueCatalogTable.name}"'
* - id: applymapping1
* nodeType: ApplyMapping
* args:
* - name: mappings
* value: '[("column1", "string", "column1", "string")]'
* - id: selectfields2
* nodeType: SelectFields
* args:
* - name: paths
* value: '["column1"]'
* - id: resolvechoice3
* nodeType: ResolveChoice
* args:
* - name: choice
* value: '"MATCH_CATALOG"'
* - name: database
* value: '"${destination.name}"'
* - name: table_name
* value: '"${destinationAwsGlueCatalogTable.name}"'
* - id: datasink4
* nodeType: DataSink
* args:
* - name: database
* value: '"${destination.name}"'
* - name: table_name
* value: '"${destinationAwsGlueCatalogTable.name}"'
* outputs:
* scalaCode: ${example.scalaCode}
* ```
*
* @param argument A collection of arguments for invoking getScript.
* @return A collection of values returned by getScript.
*/
public suspend fun getScript(argument: GetScriptPlainArgs): GetScriptResult =
getScriptResultToKotlin(getScriptPlain(argument.toJava()).await())
/**
* @see [getScript].
* @param dagEdges List of the edges in the DAG. Defined below.
* @param dagNodes List of the nodes in the DAG. Defined below.
* @param language Programming language of the resulting code from the DAG. Defaults to `PYTHON`. Valid values are `PYTHON` and `SCALA`.
* @return A collection of values returned by getScript.
*/
public suspend fun getScript(
dagEdges: List,
dagNodes: List,
language: String? = null,
): GetScriptResult {
val argument = GetScriptPlainArgs(
dagEdges = dagEdges,
dagNodes = dagNodes,
language = language,
)
return getScriptResultToKotlin(getScriptPlain(argument.toJava()).await())
}
/**
* @see [getScript].
* @param argument Builder for [com.pulumi.aws.glue.kotlin.inputs.GetScriptPlainArgs].
* @return A collection of values returned by getScript.
*/
public suspend fun getScript(argument: suspend GetScriptPlainArgsBuilder.() -> Unit): GetScriptResult {
val builder = GetScriptPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getScriptResultToKotlin(getScriptPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy