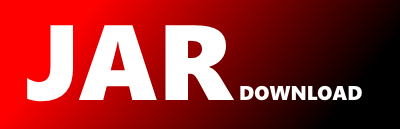
com.pulumi.aws.glue.kotlin.Partition.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.glue.kotlin
import com.pulumi.aws.glue.kotlin.outputs.PartitionStorageDescriptor
import com.pulumi.aws.glue.kotlin.outputs.PartitionStorageDescriptor.Companion.toKotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
/**
* Builder for [Partition].
*/
@PulumiTagMarker
public class PartitionResourceBuilder internal constructor() {
public var name: String? = null
public var args: PartitionArgs = PartitionArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend PartitionArgsBuilder.() -> Unit) {
val builder = PartitionArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Partition {
val builtJavaResource = com.pulumi.aws.glue.Partition(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Partition(builtJavaResource)
}
}
/**
* Provides a Glue Partition Resource.
* ## Example Usage
*
* ```yaml
* resources:
* example:
* type: aws:glue:Partition
* properties:
* databaseName: some-database
* tableName: some-table
* values:
* - some-value
* ```
*
* ## Import
* Using `pulumi import`, import Glue Partitions using the catalog ID (usually AWS account ID), database name, table name and partition values. For example:
* ```sh
* $ pulumi import aws:glue/partition:Partition part 123456789012:MyDatabase:MyTable:val1#val2
* ```
*/
public class Partition internal constructor(
override val javaResource: com.pulumi.aws.glue.Partition,
) : KotlinCustomResource(javaResource, PartitionMapper) {
/**
* ID of the Glue Catalog and database to create the table in. If omitted, this defaults to the AWS Account ID plus the database name.
*/
public val catalogId: Output
get() = javaResource.catalogId().applyValue({ args0 -> args0 })
/**
* The time at which the partition was created.
*/
public val creationTime: Output
get() = javaResource.creationTime().applyValue({ args0 -> args0 })
/**
* Name of the metadata database where the table metadata resides. For Hive compatibility, this must be all lowercase.
*/
public val databaseName: Output
get() = javaResource.databaseName().applyValue({ args0 -> args0 })
/**
* The last time at which the partition was accessed.
*/
public val lastAccessedTime: Output
get() = javaResource.lastAccessedTime().applyValue({ args0 -> args0 })
/**
* The last time at which column statistics were computed for this partition.
*/
public val lastAnalyzedTime: Output
get() = javaResource.lastAnalyzedTime().applyValue({ args0 -> args0 })
/**
* Properties associated with this table, as a list of key-value pairs.
*/
public val parameters: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy