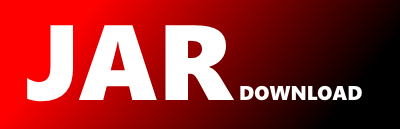
com.pulumi.aws.glue.kotlin.ResourcePolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.glue.kotlin
import com.pulumi.aws.glue.ResourcePolicyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Provides a Glue resource policy. Only one can exist per region.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const current = aws.getCallerIdentity({});
* const currentGetPartition = aws.getPartition({});
* const currentGetRegion = aws.getRegion({});
* const glue-example-policy = Promise.all([currentGetPartition, currentGetRegion, current]).then(([currentGetPartition, currentGetRegion, current]) => aws.iam.getPolicyDocument({
* statements: [{
* actions: ["glue:CreateTable"],
* resources: [`arn:${currentGetPartition.partition}:glue:${currentGetRegion.name}:${current.accountId}:*`],
* principals: [{
* identifiers: ["*"],
* type: "AWS",
* }],
* }],
* }));
* const example = new aws.glue.ResourcePolicy("example", {policy: glue_example_policy.then(glue_example_policy => glue_example_policy.json)});
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* current = aws.get_caller_identity()
* current_get_partition = aws.get_partition()
* current_get_region = aws.get_region()
* glue_example_policy = aws.iam.get_policy_document(statements=[{
* "actions": ["glue:CreateTable"],
* "resources": [f"arn:{current_get_partition.partition}:glue:{current_get_region.name}:{current.account_id}:*"],
* "principals": [{
* "identifiers": ["*"],
* "type": "AWS",
* }],
* }])
* example = aws.glue.ResourcePolicy("example", policy=glue_example_policy.json)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var current = Aws.GetCallerIdentity.Invoke();
* var currentGetPartition = Aws.GetPartition.Invoke();
* var currentGetRegion = Aws.GetRegion.Invoke();
* var glue_example_policy = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Actions = new[]
* {
* "glue:CreateTable",
* },
* Resources = new[]
* {
* $"arn:{currentGetPartition.Apply(getPartitionResult => getPartitionResult.Partition)}:glue:{currentGetRegion.Apply(getRegionResult => getRegionResult.Name)}:{current.Apply(getCallerIdentityResult => getCallerIdentityResult.AccountId)}:*",
* },
* Principals = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementPrincipalInputArgs
* {
* Identifiers = new[]
* {
* "*",
* },
* Type = "AWS",
* },
* },
* },
* },
* });
* var example = new Aws.Glue.ResourcePolicy("example", new()
* {
* Policy = glue_example_policy.Apply(glue_example_policy => glue_example_policy.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json)),
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/glue"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* current, err := aws.GetCallerIdentity(ctx, &aws.GetCallerIdentityArgs{}, nil)
* if err != nil {
* return err
* }
* currentGetPartition, err := aws.GetPartition(ctx, &aws.GetPartitionArgs{}, nil)
* if err != nil {
* return err
* }
* currentGetRegion, err := aws.GetRegion(ctx, &aws.GetRegionArgs{}, nil)
* if err != nil {
* return err
* }
* glue_example_policy, err := iam.GetPolicyDocument(ctx, &iam.GetPolicyDocumentArgs{
* Statements: []iam.GetPolicyDocumentStatement{
* {
* Actions: []string{
* "glue:CreateTable",
* },
* Resources: []string{
* fmt.Sprintf("arn:%v:glue:%v:%v:*", currentGetPartition.Partition, currentGetRegion.Name, current.AccountId),
* },
* Principals: []iam.GetPolicyDocumentStatementPrincipal{
* {
* Identifiers: []string{
* "*",
* },
* Type: "AWS",
* },
* },
* },
* },
* }, nil)
* if err != nil {
* return err
* }
* _, err = glue.NewResourcePolicy(ctx, "example", &glue.ResourcePolicyArgs{
* Policy: pulumi.String(glue_example_policy.Json),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.AwsFunctions;
* import com.pulumi.aws.inputs.GetCallerIdentityArgs;
* import com.pulumi.aws.inputs.GetPartitionArgs;
* import com.pulumi.aws.inputs.GetRegionArgs;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.glue.ResourcePolicy;
* import com.pulumi.aws.glue.ResourcePolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var current = AwsFunctions.getCallerIdentity();
* final var currentGetPartition = AwsFunctions.getPartition();
* final var currentGetRegion = AwsFunctions.getRegion();
* final var glue-example-policy = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .actions("glue:CreateTable")
* .resources(String.format("arn:%s:glue:%s:%s:*", currentGetPartition.applyValue(getPartitionResult -> getPartitionResult.partition()),currentGetRegion.applyValue(getRegionResult -> getRegionResult.name()),current.applyValue(getCallerIdentityResult -> getCallerIdentityResult.accountId())))
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .identifiers("*")
* .type("AWS")
* .build())
* .build())
* .build());
* var example = new ResourcePolicy("example", ResourcePolicyArgs.builder()
* .policy(glue_example_policy.json())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:glue:ResourcePolicy
* properties:
* policy: ${["glue-example-policy"].json}
* variables:
* current:
* fn::invoke:
* Function: aws:getCallerIdentity
* Arguments: {}
* currentGetPartition:
* fn::invoke:
* Function: aws:getPartition
* Arguments: {}
* currentGetRegion:
* fn::invoke:
* Function: aws:getRegion
* Arguments: {}
* glue-example-policy:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - actions:
* - glue:CreateTable
* resources:
* - arn:${currentGetPartition.partition}:glue:${currentGetRegion.name}:${current.accountId}:*
* principals:
* - identifiers:
* - '*'
* type: AWS
* ```
*
* ## Import
* Using `pulumi import`, import Glue Resource Policy using the account ID. For example:
* ```sh
* $ pulumi import aws:glue/resourcePolicy:ResourcePolicy Test 12356789012
* ```
* @property enableHybrid Indicates that you are using both methods to grant cross-account. Valid values are `TRUE` and `FALSE`. Note the provider will not perform drift detetction on this field as its not return on read.
* @property policy The policy to be applied to the aws glue data catalog.
*/
public data class ResourcePolicyArgs(
public val enableHybrid: Output? = null,
public val policy: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.glue.ResourcePolicyArgs =
com.pulumi.aws.glue.ResourcePolicyArgs.builder()
.enableHybrid(enableHybrid?.applyValue({ args0 -> args0 }))
.policy(policy?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ResourcePolicyArgs].
*/
@PulumiTagMarker
public class ResourcePolicyArgsBuilder internal constructor() {
private var enableHybrid: Output? = null
private var policy: Output? = null
/**
* @param value Indicates that you are using both methods to grant cross-account. Valid values are `TRUE` and `FALSE`. Note the provider will not perform drift detetction on this field as its not return on read.
*/
@JvmName("arobbclyaojvmenq")
public suspend fun enableHybrid(`value`: Output) {
this.enableHybrid = value
}
/**
* @param value The policy to be applied to the aws glue data catalog.
*/
@JvmName("jselaweqvsnlymst")
public suspend fun policy(`value`: Output) {
this.policy = value
}
/**
* @param value Indicates that you are using both methods to grant cross-account. Valid values are `TRUE` and `FALSE`. Note the provider will not perform drift detetction on this field as its not return on read.
*/
@JvmName("hoadevrstttfenxd")
public suspend fun enableHybrid(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableHybrid = mapped
}
/**
* @param value The policy to be applied to the aws glue data catalog.
*/
@JvmName("acoowgmaedlvlase")
public suspend fun policy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.policy = mapped
}
internal fun build(): ResourcePolicyArgs = ResourcePolicyArgs(
enableHybrid = enableHybrid,
policy = policy,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy