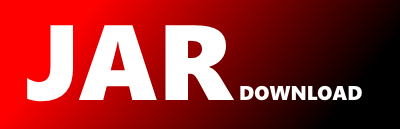
com.pulumi.aws.glue.kotlin.TriggerArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.glue.kotlin
import com.pulumi.aws.glue.TriggerArgs.builder
import com.pulumi.aws.glue.kotlin.inputs.TriggerActionArgs
import com.pulumi.aws.glue.kotlin.inputs.TriggerActionArgsBuilder
import com.pulumi.aws.glue.kotlin.inputs.TriggerEventBatchingConditionArgs
import com.pulumi.aws.glue.kotlin.inputs.TriggerEventBatchingConditionArgsBuilder
import com.pulumi.aws.glue.kotlin.inputs.TriggerPredicateArgs
import com.pulumi.aws.glue.kotlin.inputs.TriggerPredicateArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Manages a Glue Trigger resource.
* ## Example Usage
* ### Conditional Trigger
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.glue.Trigger("example", {
* name: "example",
* type: "CONDITIONAL",
* actions: [{
* jobName: example1.name,
* }],
* predicate: {
* conditions: [{
* jobName: example2.name,
* state: "SUCCEEDED",
* }],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.glue.Trigger("example",
* name="example",
* type="CONDITIONAL",
* actions=[{
* "job_name": example1["name"],
* }],
* predicate={
* "conditions": [{
* "job_name": example2["name"],
* "state": "SUCCEEDED",
* }],
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Glue.Trigger("example", new()
* {
* Name = "example",
* Type = "CONDITIONAL",
* Actions = new[]
* {
* new Aws.Glue.Inputs.TriggerActionArgs
* {
* JobName = example1.Name,
* },
* },
* Predicate = new Aws.Glue.Inputs.TriggerPredicateArgs
* {
* Conditions = new[]
* {
* new Aws.Glue.Inputs.TriggerPredicateConditionArgs
* {
* JobName = example2.Name,
* State = "SUCCEEDED",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/glue"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := glue.NewTrigger(ctx, "example", &glue.TriggerArgs{
* Name: pulumi.String("example"),
* Type: pulumi.String("CONDITIONAL"),
* Actions: glue.TriggerActionArray{
* &glue.TriggerActionArgs{
* JobName: pulumi.Any(example1.Name),
* },
* },
* Predicate: &glue.TriggerPredicateArgs{
* Conditions: glue.TriggerPredicateConditionArray{
* &glue.TriggerPredicateConditionArgs{
* JobName: pulumi.Any(example2.Name),
* State: pulumi.String("SUCCEEDED"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glue.Trigger;
* import com.pulumi.aws.glue.TriggerArgs;
* import com.pulumi.aws.glue.inputs.TriggerActionArgs;
* import com.pulumi.aws.glue.inputs.TriggerPredicateArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Trigger("example", TriggerArgs.builder()
* .name("example")
* .type("CONDITIONAL")
* .actions(TriggerActionArgs.builder()
* .jobName(example1.name())
* .build())
* .predicate(TriggerPredicateArgs.builder()
* .conditions(TriggerPredicateConditionArgs.builder()
* .jobName(example2.name())
* .state("SUCCEEDED")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:glue:Trigger
* properties:
* name: example
* type: CONDITIONAL
* actions:
* - jobName: ${example1.name}
* predicate:
* conditions:
* - jobName: ${example2.name}
* state: SUCCEEDED
* ```
*
* ### On-Demand Trigger
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.glue.Trigger("example", {
* name: "example",
* type: "ON_DEMAND",
* actions: [{
* jobName: exampleAwsGlueJob.name,
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.glue.Trigger("example",
* name="example",
* type="ON_DEMAND",
* actions=[{
* "job_name": example_aws_glue_job["name"],
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Glue.Trigger("example", new()
* {
* Name = "example",
* Type = "ON_DEMAND",
* Actions = new[]
* {
* new Aws.Glue.Inputs.TriggerActionArgs
* {
* JobName = exampleAwsGlueJob.Name,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/glue"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := glue.NewTrigger(ctx, "example", &glue.TriggerArgs{
* Name: pulumi.String("example"),
* Type: pulumi.String("ON_DEMAND"),
* Actions: glue.TriggerActionArray{
* &glue.TriggerActionArgs{
* JobName: pulumi.Any(exampleAwsGlueJob.Name),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glue.Trigger;
* import com.pulumi.aws.glue.TriggerArgs;
* import com.pulumi.aws.glue.inputs.TriggerActionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Trigger("example", TriggerArgs.builder()
* .name("example")
* .type("ON_DEMAND")
* .actions(TriggerActionArgs.builder()
* .jobName(exampleAwsGlueJob.name())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:glue:Trigger
* properties:
* name: example
* type: ON_DEMAND
* actions:
* - jobName: ${exampleAwsGlueJob.name}
* ```
*
* ### Scheduled Trigger
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.glue.Trigger("example", {
* name: "example",
* schedule: "cron(15 12 * * ? *)",
* type: "SCHEDULED",
* actions: [{
* jobName: exampleAwsGlueJob.name,
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.glue.Trigger("example",
* name="example",
* schedule="cron(15 12 * * ? *)",
* type="SCHEDULED",
* actions=[{
* "job_name": example_aws_glue_job["name"],
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Glue.Trigger("example", new()
* {
* Name = "example",
* Schedule = "cron(15 12 * * ? *)",
* Type = "SCHEDULED",
* Actions = new[]
* {
* new Aws.Glue.Inputs.TriggerActionArgs
* {
* JobName = exampleAwsGlueJob.Name,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/glue"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := glue.NewTrigger(ctx, "example", &glue.TriggerArgs{
* Name: pulumi.String("example"),
* Schedule: pulumi.String("cron(15 12 * * ? *)"),
* Type: pulumi.String("SCHEDULED"),
* Actions: glue.TriggerActionArray{
* &glue.TriggerActionArgs{
* JobName: pulumi.Any(exampleAwsGlueJob.Name),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glue.Trigger;
* import com.pulumi.aws.glue.TriggerArgs;
* import com.pulumi.aws.glue.inputs.TriggerActionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Trigger("example", TriggerArgs.builder()
* .name("example")
* .schedule("cron(15 12 * * ? *)")
* .type("SCHEDULED")
* .actions(TriggerActionArgs.builder()
* .jobName(exampleAwsGlueJob.name())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:glue:Trigger
* properties:
* name: example
* schedule: cron(15 12 * * ? *)
* type: SCHEDULED
* actions:
* - jobName: ${exampleAwsGlueJob.name}
* ```
*
* ### Conditional Trigger with Crawler Action
* **Note:** Triggers can have both a crawler action and a crawler condition, just no example provided.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.glue.Trigger("example", {
* name: "example",
* type: "CONDITIONAL",
* actions: [{
* crawlerName: example1.name,
* }],
* predicate: {
* conditions: [{
* jobName: example2.name,
* state: "SUCCEEDED",
* }],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.glue.Trigger("example",
* name="example",
* type="CONDITIONAL",
* actions=[{
* "crawler_name": example1["name"],
* }],
* predicate={
* "conditions": [{
* "job_name": example2["name"],
* "state": "SUCCEEDED",
* }],
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Glue.Trigger("example", new()
* {
* Name = "example",
* Type = "CONDITIONAL",
* Actions = new[]
* {
* new Aws.Glue.Inputs.TriggerActionArgs
* {
* CrawlerName = example1.Name,
* },
* },
* Predicate = new Aws.Glue.Inputs.TriggerPredicateArgs
* {
* Conditions = new[]
* {
* new Aws.Glue.Inputs.TriggerPredicateConditionArgs
* {
* JobName = example2.Name,
* State = "SUCCEEDED",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/glue"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := glue.NewTrigger(ctx, "example", &glue.TriggerArgs{
* Name: pulumi.String("example"),
* Type: pulumi.String("CONDITIONAL"),
* Actions: glue.TriggerActionArray{
* &glue.TriggerActionArgs{
* CrawlerName: pulumi.Any(example1.Name),
* },
* },
* Predicate: &glue.TriggerPredicateArgs{
* Conditions: glue.TriggerPredicateConditionArray{
* &glue.TriggerPredicateConditionArgs{
* JobName: pulumi.Any(example2.Name),
* State: pulumi.String("SUCCEEDED"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glue.Trigger;
* import com.pulumi.aws.glue.TriggerArgs;
* import com.pulumi.aws.glue.inputs.TriggerActionArgs;
* import com.pulumi.aws.glue.inputs.TriggerPredicateArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Trigger("example", TriggerArgs.builder()
* .name("example")
* .type("CONDITIONAL")
* .actions(TriggerActionArgs.builder()
* .crawlerName(example1.name())
* .build())
* .predicate(TriggerPredicateArgs.builder()
* .conditions(TriggerPredicateConditionArgs.builder()
* .jobName(example2.name())
* .state("SUCCEEDED")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:glue:Trigger
* properties:
* name: example
* type: CONDITIONAL
* actions:
* - crawlerName: ${example1.name}
* predicate:
* conditions:
* - jobName: ${example2.name}
* state: SUCCEEDED
* ```
*
* ### Conditional Trigger with Crawler Condition
* **Note:** Triggers can have both a crawler action and a crawler condition, just no example provided.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.glue.Trigger("example", {
* name: "example",
* type: "CONDITIONAL",
* actions: [{
* jobName: example1.name,
* }],
* predicate: {
* conditions: [{
* crawlerName: example2.name,
* crawlState: "SUCCEEDED",
* }],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.glue.Trigger("example",
* name="example",
* type="CONDITIONAL",
* actions=[{
* "job_name": example1["name"],
* }],
* predicate={
* "conditions": [{
* "crawler_name": example2["name"],
* "crawl_state": "SUCCEEDED",
* }],
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Glue.Trigger("example", new()
* {
* Name = "example",
* Type = "CONDITIONAL",
* Actions = new[]
* {
* new Aws.Glue.Inputs.TriggerActionArgs
* {
* JobName = example1.Name,
* },
* },
* Predicate = new Aws.Glue.Inputs.TriggerPredicateArgs
* {
* Conditions = new[]
* {
* new Aws.Glue.Inputs.TriggerPredicateConditionArgs
* {
* CrawlerName = example2.Name,
* CrawlState = "SUCCEEDED",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/glue"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := glue.NewTrigger(ctx, "example", &glue.TriggerArgs{
* Name: pulumi.String("example"),
* Type: pulumi.String("CONDITIONAL"),
* Actions: glue.TriggerActionArray{
* &glue.TriggerActionArgs{
* JobName: pulumi.Any(example1.Name),
* },
* },
* Predicate: &glue.TriggerPredicateArgs{
* Conditions: glue.TriggerPredicateConditionArray{
* &glue.TriggerPredicateConditionArgs{
* CrawlerName: pulumi.Any(example2.Name),
* CrawlState: pulumi.String("SUCCEEDED"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glue.Trigger;
* import com.pulumi.aws.glue.TriggerArgs;
* import com.pulumi.aws.glue.inputs.TriggerActionArgs;
* import com.pulumi.aws.glue.inputs.TriggerPredicateArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Trigger("example", TriggerArgs.builder()
* .name("example")
* .type("CONDITIONAL")
* .actions(TriggerActionArgs.builder()
* .jobName(example1.name())
* .build())
* .predicate(TriggerPredicateArgs.builder()
* .conditions(TriggerPredicateConditionArgs.builder()
* .crawlerName(example2.name())
* .crawlState("SUCCEEDED")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:glue:Trigger
* properties:
* name: example
* type: CONDITIONAL
* actions:
* - jobName: ${example1.name}
* predicate:
* conditions:
* - crawlerName: ${example2.name}
* crawlState: SUCCEEDED
* ```
*
* ## Import
* Using `pulumi import`, import Glue Triggers using `name`. For example:
* ```sh
* $ pulumi import aws:glue/trigger:Trigger MyTrigger MyTrigger
* ```
* @property actions List of actions initiated by this trigger when it fires. See Actions Below.
* @property description A description of the new trigger.
* @property enabled Start the trigger. Defaults to `true`.
* @property eventBatchingConditions Batch condition that must be met (specified number of events received or batch time window expired) before EventBridge event trigger fires. See Event Batching Condition.
* @property name The name of the trigger.
* @property predicate A predicate to specify when the new trigger should fire. Required when trigger type is `CONDITIONAL`. See Predicate Below.
* @property schedule A cron expression used to specify the schedule. [Time-Based Schedules for Jobs and Crawlers](https://docs.aws.amazon.com/glue/latest/dg/monitor-data-warehouse-schedule.html)
* @property startOnCreation Set to true to start `SCHEDULED` and `CONDITIONAL` triggers when created. True is not supported for `ON_DEMAND` triggers.
* @property tags Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
* @property type The type of trigger. Valid values are `CONDITIONAL`, `EVENT`, `ON_DEMAND`, and `SCHEDULED`.
* @property workflowName A workflow to which the trigger should be associated to. Every workflow graph (DAG) needs a starting trigger (`ON_DEMAND` or `SCHEDULED` type) and can contain multiple additional `CONDITIONAL` triggers.
*/
public data class TriggerArgs(
public val actions: Output>? = null,
public val description: Output? = null,
public val enabled: Output? = null,
public val eventBatchingConditions: Output>? = null,
public val name: Output? = null,
public val predicate: Output? = null,
public val schedule: Output? = null,
public val startOnCreation: Output? = null,
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy