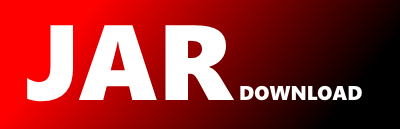
com.pulumi.aws.glue.kotlin.inputs.CrawlerIcebergTargetArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.glue.kotlin.inputs
import com.pulumi.aws.glue.inputs.CrawlerIcebergTargetArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property connectionName The name of the connection to use to connect to the Iceberg target.
* @property exclusions A list of glob patterns used to exclude from the crawl.
* @property maximumTraversalDepth The maximum depth of Amazon S3 paths that the crawler can traverse to discover the Iceberg metadata folder in your Amazon S3 path. Used to limit the crawler run time. Valid values are between `1` and `20`.
* @property paths One or more Amazon S3 paths that contains Iceberg metadata folders as s3://bucket/prefix.
*/
public data class CrawlerIcebergTargetArgs(
public val connectionName: Output? = null,
public val exclusions: Output>? = null,
public val maximumTraversalDepth: Output,
public val paths: Output>,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.glue.inputs.CrawlerIcebergTargetArgs =
com.pulumi.aws.glue.inputs.CrawlerIcebergTargetArgs.builder()
.connectionName(connectionName?.applyValue({ args0 -> args0 }))
.exclusions(exclusions?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.maximumTraversalDepth(maximumTraversalDepth.applyValue({ args0 -> args0 }))
.paths(paths.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [CrawlerIcebergTargetArgs].
*/
@PulumiTagMarker
public class CrawlerIcebergTargetArgsBuilder internal constructor() {
private var connectionName: Output? = null
private var exclusions: Output>? = null
private var maximumTraversalDepth: Output? = null
private var paths: Output>? = null
/**
* @param value The name of the connection to use to connect to the Iceberg target.
*/
@JvmName("eiqiocsifeleegmm")
public suspend fun connectionName(`value`: Output) {
this.connectionName = value
}
/**
* @param value A list of glob patterns used to exclude from the crawl.
*/
@JvmName("uomajyjtlmkgfgnl")
public suspend fun exclusions(`value`: Output>) {
this.exclusions = value
}
@JvmName("phwvwhloaqfareka")
public suspend fun exclusions(vararg values: Output) {
this.exclusions = Output.all(values.asList())
}
/**
* @param values A list of glob patterns used to exclude from the crawl.
*/
@JvmName("sjyyweflpgaawiaj")
public suspend fun exclusions(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy