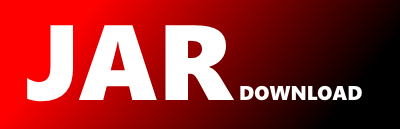
com.pulumi.aws.glue.kotlin.inputs.GetScriptPlainArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.glue.kotlin.inputs
import com.pulumi.aws.glue.inputs.GetScriptPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getScript.
* @property dagEdges List of the edges in the DAG. Defined below.
* @property dagNodes List of the nodes in the DAG. Defined below.
* @property language Programming language of the resulting code from the DAG. Defaults to `PYTHON`. Valid values are `PYTHON` and `SCALA`.
*/
public data class GetScriptPlainArgs(
public val dagEdges: List,
public val dagNodes: List,
public val language: String? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.glue.inputs.GetScriptPlainArgs =
com.pulumi.aws.glue.inputs.GetScriptPlainArgs.builder()
.dagEdges(dagEdges.let({ args0 -> args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) }) }))
.dagNodes(dagNodes.let({ args0 -> args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) }) }))
.language(language?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetScriptPlainArgs].
*/
@PulumiTagMarker
public class GetScriptPlainArgsBuilder internal constructor() {
private var dagEdges: List? = null
private var dagNodes: List? = null
private var language: String? = null
/**
* @param value List of the edges in the DAG. Defined below.
*/
@JvmName("xrfuowkcwlrjduod")
public suspend fun dagEdges(`value`: List) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.dagEdges = mapped
}
/**
* @param argument List of the edges in the DAG. Defined below.
*/
@JvmName("yiwrqsnsllllmdyf")
public suspend fun dagEdges(argument: List Unit>) {
val toBeMapped = argument.toList().map {
GetScriptDagEdgeBuilder().applySuspend { it() }.build()
}
val mapped = toBeMapped
this.dagEdges = mapped
}
/**
* @param argument List of the edges in the DAG. Defined below.
*/
@JvmName("trgfwrjuhylvudjg")
public suspend fun dagEdges(vararg argument: suspend GetScriptDagEdgeBuilder.() -> Unit) {
val toBeMapped = argument.toList().map {
GetScriptDagEdgeBuilder().applySuspend { it() }.build()
}
val mapped = toBeMapped
this.dagEdges = mapped
}
/**
* @param argument List of the edges in the DAG. Defined below.
*/
@JvmName("oatvvupvadxvukbk")
public suspend fun dagEdges(argument: suspend GetScriptDagEdgeBuilder.() -> Unit) {
val toBeMapped = listOf(GetScriptDagEdgeBuilder().applySuspend { argument() }.build())
val mapped = toBeMapped
this.dagEdges = mapped
}
/**
* @param values List of the edges in the DAG. Defined below.
*/
@JvmName("qiffegmruwsbmjhg")
public suspend fun dagEdges(vararg values: GetScriptDagEdge) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.dagEdges = mapped
}
/**
* @param value List of the nodes in the DAG. Defined below.
*/
@JvmName("smiccdcqhhfvlpdr")
public suspend fun dagNodes(`value`: List) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.dagNodes = mapped
}
/**
* @param argument List of the nodes in the DAG. Defined below.
*/
@JvmName("cfmgcbwmijaiodsf")
public suspend fun dagNodes(argument: List Unit>) {
val toBeMapped = argument.toList().map {
GetScriptDagNodeBuilder().applySuspend { it() }.build()
}
val mapped = toBeMapped
this.dagNodes = mapped
}
/**
* @param argument List of the nodes in the DAG. Defined below.
*/
@JvmName("ngorigggvublgohg")
public suspend fun dagNodes(vararg argument: suspend GetScriptDagNodeBuilder.() -> Unit) {
val toBeMapped = argument.toList().map {
GetScriptDagNodeBuilder().applySuspend { it() }.build()
}
val mapped = toBeMapped
this.dagNodes = mapped
}
/**
* @param argument List of the nodes in the DAG. Defined below.
*/
@JvmName("lttqxyoxpdlraotd")
public suspend fun dagNodes(argument: suspend GetScriptDagNodeBuilder.() -> Unit) {
val toBeMapped = listOf(GetScriptDagNodeBuilder().applySuspend { argument() }.build())
val mapped = toBeMapped
this.dagNodes = mapped
}
/**
* @param values List of the nodes in the DAG. Defined below.
*/
@JvmName("vkfknwinbuclmjjk")
public suspend fun dagNodes(vararg values: GetScriptDagNode) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.dagNodes = mapped
}
/**
* @param value Programming language of the resulting code from the DAG. Defaults to `PYTHON`. Valid values are `PYTHON` and `SCALA`.
*/
@JvmName("ubwyqlejgwqhcoqq")
public suspend fun language(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.language = mapped
}
internal fun build(): GetScriptPlainArgs = GetScriptPlainArgs(
dagEdges = dagEdges ?: throw PulumiNullFieldException("dagEdges"),
dagNodes = dagNodes ?: throw PulumiNullFieldException("dagNodes"),
language = language,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy