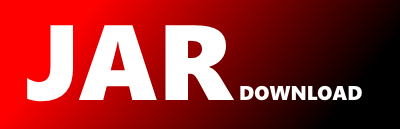
com.pulumi.aws.guardduty.kotlin.Member.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.guardduty.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [Member].
*/
@PulumiTagMarker
public class MemberResourceBuilder internal constructor() {
public var name: String? = null
public var args: MemberArgs = MemberArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend MemberArgsBuilder.() -> Unit) {
val builder = MemberArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Member {
val builtJavaResource = com.pulumi.aws.guardduty.Member(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Member(builtJavaResource)
}
}
/**
* Provides a resource to manage a GuardDuty member. To accept invitations in member accounts, see the `aws.guardduty.InviteAccepter` resource.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const primary = new aws.guardduty.Detector("primary", {enable: true});
* const member = new aws.guardduty.Detector("member", {enable: true});
* const memberMember = new aws.guardduty.Member("member", {
* accountId: member.accountId,
* detectorId: primary.id,
* email: "[email protected]",
* invite: true,
* invitationMessage: "please accept guardduty invitation",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* primary = aws.guardduty.Detector("primary", enable=True)
* member = aws.guardduty.Detector("member", enable=True)
* member_member = aws.guardduty.Member("member",
* account_id=member.account_id,
* detector_id=primary.id,
* email="[email protected]",
* invite=True,
* invitation_message="please accept guardduty invitation")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var primary = new Aws.GuardDuty.Detector("primary", new()
* {
* Enable = true,
* });
* var member = new Aws.GuardDuty.Detector("member", new()
* {
* Enable = true,
* });
* var memberMember = new Aws.GuardDuty.Member("member", new()
* {
* AccountId = member.AccountId,
* DetectorId = primary.Id,
* Email = "[email protected]",
* Invite = true,
* InvitationMessage = "please accept guardduty invitation",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/guardduty"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* primary, err := guardduty.NewDetector(ctx, "primary", &guardduty.DetectorArgs{
* Enable: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* member, err := guardduty.NewDetector(ctx, "member", &guardduty.DetectorArgs{
* Enable: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* _, err = guardduty.NewMember(ctx, "member", &guardduty.MemberArgs{
* AccountId: member.AccountId,
* DetectorId: primary.ID(),
* Email: pulumi.String("[email protected]"),
* Invite: pulumi.Bool(true),
* InvitationMessage: pulumi.String("please accept guardduty invitation"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.guardduty.Detector;
* import com.pulumi.aws.guardduty.DetectorArgs;
* import com.pulumi.aws.guardduty.Member;
* import com.pulumi.aws.guardduty.MemberArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var primary = new Detector("primary", DetectorArgs.builder()
* .enable(true)
* .build());
* var member = new Detector("member", DetectorArgs.builder()
* .enable(true)
* .build());
* var memberMember = new Member("memberMember", MemberArgs.builder()
* .accountId(member.accountId())
* .detectorId(primary.id())
* .email("[email protected]")
* .invite(true)
* .invitationMessage("please accept guardduty invitation")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* primary:
* type: aws:guardduty:Detector
* properties:
* enable: true
* member:
* type: aws:guardduty:Detector
* properties:
* enable: true
* memberMember:
* type: aws:guardduty:Member
* name: member
* properties:
* accountId: ${member.accountId}
* detectorId: ${primary.id}
* email: [email protected]
* invite: true
* invitationMessage: please accept guardduty invitation
* ```
*
* ## Import
* Using `pulumi import`, import GuardDuty members using the primary GuardDuty detector ID and member AWS account ID. For example:
* ```sh
* $ pulumi import aws:guardduty/member:Member MyMember 00b00fd5aecc0ab60a708659477e9617:123456789012
* ```
*/
public class Member internal constructor(
override val javaResource: com.pulumi.aws.guardduty.Member,
) : KotlinCustomResource(javaResource, MemberMapper) {
/**
* AWS account ID for member account.
*/
public val accountId: Output
get() = javaResource.accountId().applyValue({ args0 -> args0 })
/**
* The detector ID of the GuardDuty account where you want to create member accounts.
*/
public val detectorId: Output
get() = javaResource.detectorId().applyValue({ args0 -> args0 })
/**
* Boolean whether an email notification is sent to the accounts. Defaults to `false`.
*/
public val disableEmailNotification: Output?
get() = javaResource.disableEmailNotification().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Email address for member account.
*/
public val email: Output
get() = javaResource.email().applyValue({ args0 -> args0 })
/**
* Message for invitation.
*/
public val invitationMessage: Output?
get() = javaResource.invitationMessage().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Boolean whether to invite the account to GuardDuty as a member. Defaults to `false`. To detect if an invitation needs to be (re-)sent, the this provider state value is `true` based on a `relationship_status` of `Disabled`, `Enabled`, `Invited`, or `EmailVerificationInProgress`.
*/
public val invite: Output?
get() = javaResource.invite().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The status of the relationship between the member account and its primary account. More information can be found in [Amazon GuardDuty API Reference](https://docs.aws.amazon.com/guardduty/latest/ug/get-members.html).
*/
public val relationshipStatus: Output
get() = javaResource.relationshipStatus().applyValue({ args0 -> args0 })
}
public object MemberMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.aws.guardduty.Member::class == javaResource::class
override fun map(javaResource: Resource): Member = Member(
javaResource as
com.pulumi.aws.guardduty.Member,
)
}
/**
* @see [Member].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Member].
*/
public suspend fun member(name: String, block: suspend MemberResourceBuilder.() -> Unit): Member {
val builder = MemberResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Member].
* @param name The _unique_ name of the resulting resource.
*/
public fun member(name: String): Member {
val builder = MemberResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy