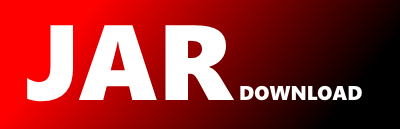
com.pulumi.aws.iam.kotlin.ServerCertificate.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.iam.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
/**
* Builder for [ServerCertificate].
*/
@PulumiTagMarker
public class ServerCertificateResourceBuilder internal constructor() {
public var name: String? = null
public var args: ServerCertificateArgs = ServerCertificateArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ServerCertificateArgsBuilder.() -> Unit) {
val builder = ServerCertificateArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ServerCertificate {
val builtJavaResource = com.pulumi.aws.iam.ServerCertificate(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ServerCertificate(builtJavaResource)
}
}
/**
* Provides an IAM Server Certificate resource to upload Server Certificates.
* Certs uploaded to IAM can easily work with other AWS services such as:
* - AWS Elastic Beanstalk
* - Elastic Load Balancing
* - CloudFront
* - AWS OpsWorks
* For information about server certificates in IAM, see [Managing Server
* Certificates][2] in AWS Documentation.
* ## Example Usage
* **Using certs on file:**
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* import * as std from "@pulumi/std";
* const testCert = new aws.iam.ServerCertificate("test_cert", {
* name: "some_test_cert",
* certificateBody: std.file({
* input: "self-ca-cert.pem",
* }).then(invoke => invoke.result),
* privateKey: std.file({
* input: "test-key.pem",
* }).then(invoke => invoke.result),
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* import pulumi_std as std
* test_cert = aws.iam.ServerCertificate("test_cert",
* name="some_test_cert",
* certificate_body=std.file(input="self-ca-cert.pem").result,
* private_key=std.file(input="test-key.pem").result)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* using Std = Pulumi.Std;
* return await Deployment.RunAsync(() =>
* {
* var testCert = new Aws.Iam.ServerCertificate("test_cert", new()
* {
* Name = "some_test_cert",
* CertificateBody = Std.File.Invoke(new()
* {
* Input = "self-ca-cert.pem",
* }).Apply(invoke => invoke.Result),
* PrivateKey = Std.File.Invoke(new()
* {
* Input = "test-key.pem",
* }).Apply(invoke => invoke.Result),
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi-std/sdk/go/std"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* invokeFile, err := std.File(ctx, &std.FileArgs{
* Input: "self-ca-cert.pem",
* }, nil)
* if err != nil {
* return err
* }
* invokeFile1, err := std.File(ctx, &std.FileArgs{
* Input: "test-key.pem",
* }, nil)
* if err != nil {
* return err
* }
* _, err = iam.NewServerCertificate(ctx, "test_cert", &iam.ServerCertificateArgs{
* Name: pulumi.String("some_test_cert"),
* CertificateBody: pulumi.String(invokeFile.Result),
* PrivateKey: pulumi.String(invokeFile1.Result),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.ServerCertificate;
* import com.pulumi.aws.iam.ServerCertificateArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var testCert = new ServerCertificate("testCert", ServerCertificateArgs.builder()
* .name("some_test_cert")
* .certificateBody(StdFunctions.file(FileArgs.builder()
* .input("self-ca-cert.pem")
* .build()).result())
* .privateKey(StdFunctions.file(FileArgs.builder()
* .input("test-key.pem")
* .build()).result())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* testCert:
* type: aws:iam:ServerCertificate
* name: test_cert
* properties:
* name: some_test_cert
* certificateBody:
* fn::invoke:
* Function: std:file
* Arguments:
* input: self-ca-cert.pem
* Return: result
* privateKey:
* fn::invoke:
* Function: std:file
* Arguments:
* input: test-key.pem
* Return: result
* ```
*
* **Example with cert in-line:**
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const testCertAlt = new aws.iam.ServerCertificate("test_cert_alt", {
* name: "alt_test_cert",
* certificateBody: `-----BEGIN CERTIFICATE-----
* [......] # cert contents
* -----END CERTIFICATE-----
* `,
* privateKey: `-----BEGIN RSA PRIVATE KEY-----
* [......] # cert contents
* -----END RSA PRIVATE KEY-----
* `,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* test_cert_alt = aws.iam.ServerCertificate("test_cert_alt",
* name="alt_test_cert",
* certificate_body="""-----BEGIN CERTIFICATE-----
* [......] # cert contents
* -----END CERTIFICATE-----
* """,
* private_key="""-----BEGIN RSA PRIVATE KEY-----
* [......] # cert contents
* -----END RSA PRIVATE KEY-----
* """)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var testCertAlt = new Aws.Iam.ServerCertificate("test_cert_alt", new()
* {
* Name = "alt_test_cert",
* CertificateBody = @"-----BEGIN CERTIFICATE-----
* [......] # cert contents
* -----END CERTIFICATE-----
* ",
* PrivateKey = @"-----BEGIN RSA PRIVATE KEY-----
* [......] # cert contents
* -----END RSA PRIVATE KEY-----
* ",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := iam.NewServerCertificate(ctx, "test_cert_alt", &iam.ServerCertificateArgs{
* Name: pulumi.String("alt_test_cert"),
* CertificateBody: pulumi.String("-----BEGIN CERTIFICATE-----\n[......] # cert contents\n-----END CERTIFICATE-----\n"),
* PrivateKey: pulumi.String("-----BEGIN RSA PRIVATE KEY-----\n[......] # cert contents\n-----END RSA PRIVATE KEY-----\n"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.ServerCertificate;
* import com.pulumi.aws.iam.ServerCertificateArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var testCertAlt = new ServerCertificate("testCertAlt", ServerCertificateArgs.builder()
* .name("alt_test_cert")
* .certificateBody("""
* -----BEGIN CERTIFICATE-----
* [......] # cert contents
* -----END CERTIFICATE-----
* """)
* .privateKey("""
* -----BEGIN RSA PRIVATE KEY-----
* [......] # cert contents
* -----END RSA PRIVATE KEY-----
* """)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* testCertAlt:
* type: aws:iam:ServerCertificate
* name: test_cert_alt
* properties:
* name: alt_test_cert
* certificateBody: |
* -----BEGIN CERTIFICATE-----
* [......] # cert contents
* -----END CERTIFICATE-----
* privateKey: |
* -----BEGIN RSA PRIVATE KEY-----
* [......] # cert contents
* -----END RSA PRIVATE KEY-----
* ```
*
* **Use in combination with an AWS ELB resource:**
* Some properties of an IAM Server Certificates cannot be updated while they are
* in use. In order for the provider to effectively manage a Certificate in this situation, it is
* recommended you utilize the `name_prefix` attribute and enable the
* `create_before_destroy`. This will allow this provider
* to create a new, updated `aws.iam.ServerCertificate` resource and replace it in
* dependant resources before attempting to destroy the old version.
* ## Import
* Using `pulumi import`, import IAM Server Certificates using the `name`. For example:
* ```sh
* $ pulumi import aws:iam/serverCertificate:ServerCertificate certificate example.com-certificate-until-2018
* ```
*/
public class ServerCertificate internal constructor(
override val javaResource: com.pulumi.aws.iam.ServerCertificate,
) : KotlinCustomResource(javaResource, ServerCertificateMapper) {
/**
* The Amazon Resource Name (ARN) specifying the server certificate.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* The contents of the public key certificate in
* PEM-encoded format.
*/
public val certificateBody: Output
get() = javaResource.certificateBody().applyValue({ args0 -> args0 })
/**
* The contents of the certificate chain.
* This is typically a concatenation of the PEM-encoded public key certificates
* of the chain.
*/
public val certificateChain: Output?
get() = javaResource.certificateChain().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Date and time in [RFC3339 format](https://tools.ietf.org/html/rfc3339#section-5.8) on which the certificate is set to expire.
*/
public val expiration: Output
get() = javaResource.expiration().applyValue({ args0 -> args0 })
/**
* The name of the Server Certificate. Do not include the
* path in this value. If omitted, the provider will assign a random, unique name.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Creates a unique name beginning with the specified
* prefix. Conflicts with `name`.
*/
public val namePrefix: Output
get() = javaResource.namePrefix().applyValue({ args0 -> args0 })
/**
* The IAM path for the server certificate. If it is not
* included, it defaults to a slash (/). If this certificate is for use with
* AWS CloudFront, the path must be in format `/cloudfront/your_path_here`.
* See [IAM Identifiers](https://docs.aws.amazon.com/IAM/latest/UserGuide/Using_Identifiers.html) for more details on IAM Paths.
*/
public val path: Output?
get() = javaResource.path().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The contents of the private key in PEM-encoded format.
*/
public val privateKey: Output
get() = javaResource.privateKey().applyValue({ args0 -> args0 })
/**
* Map of resource tags for the server certificate. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
* > **NOTE:** AWS performs behind-the-scenes modifications to some certificate files if they do not adhere to a specific format. These modifications will result in this provider forever believing that it needs to update the resources since the local and AWS file contents will not match after theses modifications occur. In order to prevent this from happening you must ensure that all your PEM-encoded files use UNIX line-breaks and that `certificate_body` contains only one certificate. All other certificates should go in `certificate_chain`. It is common for some Certificate Authorities to issue certificate files that have DOS line-breaks and that are actually multiple certificates concatenated together in order to form a full certificate chain.
*/
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy