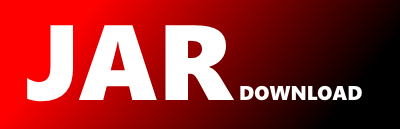
com.pulumi.aws.iam.kotlin.SshKeyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.iam.kotlin
import com.pulumi.aws.iam.SshKeyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Uploads an SSH public key and associates it with the specified IAM user.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const user = new aws.iam.User("user", {
* name: "test-user",
* path: "/",
* });
* const userSshKey = new aws.iam.SshKey("user", {
* username: user.name,
* encoding: "SSH",
* publicKey: "ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAABAQD3F6tyPEFEzV0LX3X8BsXdMsQz1x2cEikKDEY0aIj41qgxMCP/iteneqXSIFZBp5vizPvaoIR3Um9xK7PGoW8giupGn+EPuxIA4cDM4vzOqOkiMPhz5XK0whEjkVzTo4+S0puvDZuwIsdiW9mxhJc7tgBNL0cYlWSYVkz4G/fslNfRPW5mYAM49f4fhtxPb5ok4Q2Lg9dPKVHO/Bgeu5woMc7RY0p1ej6D4CKFE6lymSDJpW0YHX/wqE9+cfEauh7xZcG0q9t2ta6F6fmX0agvpFyZo8aFbXeUBr7osSCJNgvavWbM/06niWrOvYX2xwWdhXmXSrbX8ZbabVohBK41 [email protected]",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* user = aws.iam.User("user",
* name="test-user",
* path="/")
* user_ssh_key = aws.iam.SshKey("user",
* username=user.name,
* encoding="SSH",
* public_key="ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAABAQD3F6tyPEFEzV0LX3X8BsXdMsQz1x2cEikKDEY0aIj41qgxMCP/iteneqXSIFZBp5vizPvaoIR3Um9xK7PGoW8giupGn+EPuxIA4cDM4vzOqOkiMPhz5XK0whEjkVzTo4+S0puvDZuwIsdiW9mxhJc7tgBNL0cYlWSYVkz4G/fslNfRPW5mYAM49f4fhtxPb5ok4Q2Lg9dPKVHO/Bgeu5woMc7RY0p1ej6D4CKFE6lymSDJpW0YHX/wqE9+cfEauh7xZcG0q9t2ta6F6fmX0agvpFyZo8aFbXeUBr7osSCJNgvavWbM/06niWrOvYX2xwWdhXmXSrbX8ZbabVohBK41 [email protected]")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var user = new Aws.Iam.User("user", new()
* {
* Name = "test-user",
* Path = "/",
* });
* var userSshKey = new Aws.Iam.SshKey("user", new()
* {
* Username = user.Name,
* Encoding = "SSH",
* PublicKey = "ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAABAQD3F6tyPEFEzV0LX3X8BsXdMsQz1x2cEikKDEY0aIj41qgxMCP/iteneqXSIFZBp5vizPvaoIR3Um9xK7PGoW8giupGn+EPuxIA4cDM4vzOqOkiMPhz5XK0whEjkVzTo4+S0puvDZuwIsdiW9mxhJc7tgBNL0cYlWSYVkz4G/fslNfRPW5mYAM49f4fhtxPb5ok4Q2Lg9dPKVHO/Bgeu5woMc7RY0p1ej6D4CKFE6lymSDJpW0YHX/wqE9+cfEauh7xZcG0q9t2ta6F6fmX0agvpFyZo8aFbXeUBr7osSCJNgvavWbM/06niWrOvYX2xwWdhXmXSrbX8ZbabVohBK41 [email protected]",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* user, err := iam.NewUser(ctx, "user", &iam.UserArgs{
* Name: pulumi.String("test-user"),
* Path: pulumi.String("/"),
* })
* if err != nil {
* return err
* }
* _, err = iam.NewSshKey(ctx, "user", &iam.SshKeyArgs{
* Username: user.Name,
* Encoding: pulumi.String("SSH"),
* PublicKey: pulumi.String("ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAABAQD3F6tyPEFEzV0LX3X8BsXdMsQz1x2cEikKDEY0aIj41qgxMCP/iteneqXSIFZBp5vizPvaoIR3Um9xK7PGoW8giupGn+EPuxIA4cDM4vzOqOkiMPhz5XK0whEjkVzTo4+S0puvDZuwIsdiW9mxhJc7tgBNL0cYlWSYVkz4G/fslNfRPW5mYAM49f4fhtxPb5ok4Q2Lg9dPKVHO/Bgeu5woMc7RY0p1ej6D4CKFE6lymSDJpW0YHX/wqE9+cfEauh7xZcG0q9t2ta6F6fmX0agvpFyZo8aFbXeUBr7osSCJNgvavWbM/06niWrOvYX2xwWdhXmXSrbX8ZbabVohBK41 [email protected]"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.User;
* import com.pulumi.aws.iam.UserArgs;
* import com.pulumi.aws.iam.SshKey;
* import com.pulumi.aws.iam.SshKeyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var user = new User("user", UserArgs.builder()
* .name("test-user")
* .path("/")
* .build());
* var userSshKey = new SshKey("userSshKey", SshKeyArgs.builder()
* .username(user.name())
* .encoding("SSH")
* .publicKey("ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAABAQD3F6tyPEFEzV0LX3X8BsXdMsQz1x2cEikKDEY0aIj41qgxMCP/iteneqXSIFZBp5vizPvaoIR3Um9xK7PGoW8giupGn+EPuxIA4cDM4vzOqOkiMPhz5XK0whEjkVzTo4+S0puvDZuwIsdiW9mxhJc7tgBNL0cYlWSYVkz4G/fslNfRPW5mYAM49f4fhtxPb5ok4Q2Lg9dPKVHO/Bgeu5woMc7RY0p1ej6D4CKFE6lymSDJpW0YHX/wqE9+cfEauh7xZcG0q9t2ta6F6fmX0agvpFyZo8aFbXeUBr7osSCJNgvavWbM/06niWrOvYX2xwWdhXmXSrbX8ZbabVohBK41 [email protected]")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* user:
* type: aws:iam:User
* properties:
* name: test-user
* path: /
* userSshKey:
* type: aws:iam:SshKey
* name: user
* properties:
* username: ${user.name}
* encoding: SSH
* publicKey: ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAABAQD3F6tyPEFEzV0LX3X8BsXdMsQz1x2cEikKDEY0aIj41qgxMCP/iteneqXSIFZBp5vizPvaoIR3Um9xK7PGoW8giupGn+EPuxIA4cDM4vzOqOkiMPhz5XK0whEjkVzTo4+S0puvDZuwIsdiW9mxhJc7tgBNL0cYlWSYVkz4G/fslNfRPW5mYAM49f4fhtxPb5ok4Q2Lg9dPKVHO/Bgeu5woMc7RY0p1ej6D4CKFE6lymSDJpW0YHX/wqE9+cfEauh7xZcG0q9t2ta6F6fmX0agvpFyZo8aFbXeUBr7osSCJNgvavWbM/06niWrOvYX2xwWdhXmXSrbX8ZbabVohBK41 [email protected]
* ```
*
* ## Import
* Using `pulumi import`, import SSH public keys using the `username`, `ssh_public_key_id`, and `encoding`. For example:
* ```sh
* $ pulumi import aws:iam/sshKey:SshKey user user:APKAJNCNNJICVN7CFKCA:SSH
* ```
* @property encoding Specifies the public key encoding format to use in the response. To retrieve the public key in ssh-rsa format, use `SSH`. To retrieve the public key in PEM format, use `PEM`.
* @property publicKey The SSH public key. The public key must be encoded in ssh-rsa format or PEM format.
* @property status The status to assign to the SSH public key. Active means the key can be used for authentication with an AWS CodeCommit repository. Inactive means the key cannot be used. Default is `active`.
* @property username The name of the IAM user to associate the SSH public key with.
*/
public data class SshKeyArgs(
public val encoding: Output? = null,
public val publicKey: Output? = null,
public val status: Output? = null,
public val username: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.iam.SshKeyArgs = com.pulumi.aws.iam.SshKeyArgs.builder()
.encoding(encoding?.applyValue({ args0 -> args0 }))
.publicKey(publicKey?.applyValue({ args0 -> args0 }))
.status(status?.applyValue({ args0 -> args0 }))
.username(username?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SshKeyArgs].
*/
@PulumiTagMarker
public class SshKeyArgsBuilder internal constructor() {
private var encoding: Output? = null
private var publicKey: Output? = null
private var status: Output? = null
private var username: Output? = null
/**
* @param value Specifies the public key encoding format to use in the response. To retrieve the public key in ssh-rsa format, use `SSH`. To retrieve the public key in PEM format, use `PEM`.
*/
@JvmName("fnljlaoifeqmbllb")
public suspend fun encoding(`value`: Output) {
this.encoding = value
}
/**
* @param value The SSH public key. The public key must be encoded in ssh-rsa format or PEM format.
*/
@JvmName("frhrmvijssasdyde")
public suspend fun publicKey(`value`: Output) {
this.publicKey = value
}
/**
* @param value The status to assign to the SSH public key. Active means the key can be used for authentication with an AWS CodeCommit repository. Inactive means the key cannot be used. Default is `active`.
*/
@JvmName("dnhqfcuempcdnlfk")
public suspend fun status(`value`: Output) {
this.status = value
}
/**
* @param value The name of the IAM user to associate the SSH public key with.
*/
@JvmName("rupiifrhlgdajufg")
public suspend fun username(`value`: Output) {
this.username = value
}
/**
* @param value Specifies the public key encoding format to use in the response. To retrieve the public key in ssh-rsa format, use `SSH`. To retrieve the public key in PEM format, use `PEM`.
*/
@JvmName("gkvxeckdcaxwwieg")
public suspend fun encoding(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encoding = mapped
}
/**
* @param value The SSH public key. The public key must be encoded in ssh-rsa format or PEM format.
*/
@JvmName("hpoelicurdroggsj")
public suspend fun publicKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.publicKey = mapped
}
/**
* @param value The status to assign to the SSH public key. Active means the key can be used for authentication with an AWS CodeCommit repository. Inactive means the key cannot be used. Default is `active`.
*/
@JvmName("upevvxesjeydsmfj")
public suspend fun status(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.status = mapped
}
/**
* @param value The name of the IAM user to associate the SSH public key with.
*/
@JvmName("kbxglojpnlbpgshl")
public suspend fun username(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.username = mapped
}
internal fun build(): SshKeyArgs = SshKeyArgs(
encoding = encoding,
publicKey = publicKey,
status = status,
username = username,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy